Recently in my previous post, I have posted about Different ways of bootstrapping AngularJS app, where you can either auto-bootstrap using ng-app
attribute or manually bootstrap using angular.bootstrap
function. And also mentioned in angular interview questions that "Only one AngularJS application can be auto-bootstrapped per HTML document." Which means that your page can only have single ng-app
attribute. If you put multiple ng-app
attribute, only the first will be considered and the rest will be ignored.
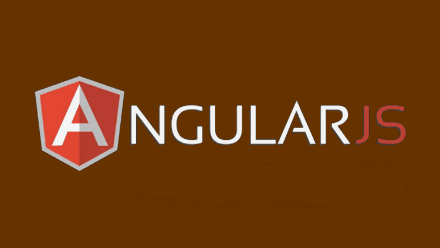
You may also like:
Let's see with an example. In the below code, there are 2 ng-app
present on the page.
<div ng-app="firstApp">
<div ng-controller="FirstController">
<p>1: {{ desc }}</p>
</div>
</div>
<div ng-app="secondApp">
<div ng-controller="SecondController">
<p>2: {{ desc }}</p>
</div>
</div>
<script src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.3.7/angular.js"></script>
<script type="text/javascript">
var firstApp = angular.module('firstApp', []);
firstApp.controller('FirstController', function($scope) {
$scope.desc = "First app.";
});
var secondApp = angular.module('secondApp', []);
secondApp.controller('SecondController', function($scope) {
$scope.desc = "Second app.";
});
</script>
And below is the output. Out of 2 ng-app
present on the page, the first one gets initialized and works as expected. Output also shows the same.
1: First app.
2: {{ desc }}
Demo
Then how can you define 2 different angular apps on the same page? Well, there are a couple of ways to implement this.
Manually Bootstrapping Each App
You can manually bootstrap both the applications using angular.bootstrap()
as we have seen in Different ways of bootstrapping AngularJS app.
var firstApp = angular.module('firstApp', []);
firstApp.controller('FirstController', function($scope) {
$scope.desc = "First app. ";
});
var secondApp = angular.module('secondApp', []);
secondApp.controller('SecondController', function($scope) {
$scope.desc = "Second app. ";
});
var dvFirst = document.getElementById('dvFirst');
var dvSecond = document.getElementById('dvSecond');
angular.element(document).ready(function() {
angular.bootstrap(dvFirst, ['firstApp']);
angular.bootstrap(dvSecond, ['secondApp']);
});
Since we are bootstrapping them manually, make sure to remove ng-app
attribute from the HTML page.
Demo
Manually Bootstrapping Second App
You can also manually bootstrap the second app, where the first app gets initialized via ng-app
.
var firstApp = angular.module('firstApp', []);
firstApp.controller('FirstController', function($scope) {
$scope.desc = "First app. ";
});
var secondApp = angular.module('secondApp', []);
secondApp.controller('SecondController', function($scope) {
$scope.desc = "Second app. ";
});
var dvSecond = document.getElementById('dvSecond');
angular.element(document).ready(function() {
angular.bootstrap(dvSecond, ['secondApp']);
});
So make sure that there is <span class="code"><gs class="GINGER_SOFTWARE_mark" ginger_software_uiphraseguid="bbf4c6ea-fa2a-499f-a3a5-7ee3a11891e3" id="babccdc4-72b1-4b4e-ac0a-8a788266ba74"><code>ng
-app present on the page for first app.
Demo
Using Dependency Injection to Inject Both in Root App
You can define a root level app using ng-app
and then inject both the apps as module in root app.
var rootApp = angular.module('rootApp', ['firstApp','secondApp']);
var firstApp = angular.module('firstApp', []);
firstApp.controller('FirstController', function($scope) {
$scope.desc = "First app. ";
});
var secondApp = angular.module('secondApp', []);
secondApp.controller('SecondController', function($scope) {
$scope.desc = "Second app. ";
});
And make sure any parent node (body/Parent div) is assigned with ng-app=rootApp"
.
Demo
Hope you find this useful!!!!!CodeProject