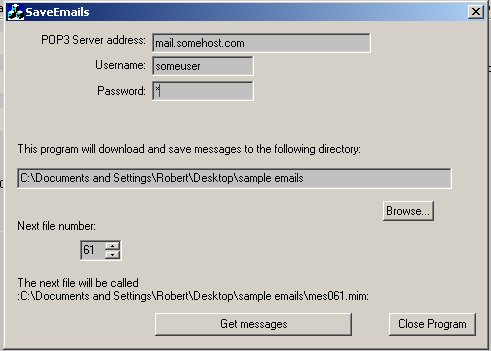
Introduction
rEmail is a set of tutorials I am writing describing how I am building tools to download, interpret and send email messages (including attachments). After the very basic first article I am moving on to the still easy one of grabbing emails from a POP3 server. If you are reading this and about to jump back and read the first article, it would be useful for you to know that you only have to download the files from this article, not the first one.
In this article I will take you on a quick tour of a class I have written called rPOP3_Client_Connection
. When constructed, this class logs into an email server. You can get the number of messages waiting, get any message and delete any message. When the class is destroyed, it will log off for you.
Using the code
- Download and unzip the files. Start a new hello world console application. (I am calling mine
xxtest
.)
- Project -> Insert Project into workspace and select rPOP3_Client.dsp.
- Make xxtest dependent on rPOP3_Client.dsp.
- And add a line underneath
#include stdafx.h
:
#include "../rPOP3_CLient/rPOP3_Client_Connection.h"
Your code should compile at this point.
Add the following into the main function:
rPOP3_Client_Connection* m_pPop3 = new
POP3_Client_Connection("user","pass","mail.host.com");
if (m_pPop3->Valid()) {
printf("Logged in ok\n");
};
delete m_pPop3;
Compiling this will give you lots of link errors. In the same way as the last article, link with ws2_32.lib. You should now be able to run it. This class works like my previous one and you should call GetStatus
if you are having problems. Look at the constructor to find out what the values returned mean.
Each message in the mailbox has a number. Once you call GetNumMessages
you know that the numbers go from one up to the value returned. I won’t bother showing you how to delete message because it’s easy but I will show you GetMessage
because it is interesting.
#include "stdafx.h"
#include "../rPOP3_CLient/rPOP3_Client_Connection.h"
int main(int argc, char* argv[])
{
printf("Hello World!\n");
rPOP3_Client_Connection* m_pPop3 = new
POP3_Client_Connection("user","pass","mail.host.com");
if (m_pPop3->Valid()) {
char buf[512];
int bytes_read=0;
int total_bytes_read = 0;
int ret = 0;
while ((ret=m_pPop3->GetMessage(&bytes_read,
2,buf,512)) == -2) {
total_bytes_read += bytes_read;
for (int e=0;e<bytes_read;e++) printf("%c",buf[e]);
};
total_bytes_read += bytes_read;
for (int e=0;e<bytes_read;e++) printf("%c",buf[e]);
printf("\n*******************\nThere were %d bytes read\n",
total_bytes_read);
};
delete m_pPop3;
return 0;
}
This is all the code for this article. Run this and you will see an email message. GetMessage
's parameters are as follows:
int *bytes_read
- Pointer to an integer. After the function call, this will hold the number of bytes that have been read.
unsigned int num
- Number of the message to be read. Don’t try reading higher than what GetNumMessages
said you could. Making my function check this value is in range would be a wasteful exercise so you have to get it right.
char* buf
- Buffer into which to chuck the data
int max_siz
- How much data I can chuck (maximum size of the buffer).
Sometimes the email message don’t fit in the buffer. If this happens, you must call GetMessage
again and it will continue giving you the same message, even if you specify a different number. Don’t let this catch you out and always check its return value wasn’t –2
. Originally this caused me problems as it meant that all subsequent commands I sent were messed up by the fact the server was still waiting for me to finish reading the last command back. I have solved this problem now and the class remembers if there is more data to be read. Thus if you try sending another command when GetMessage
hasn’t finished, the class will clear off any remaining data before executing the command. It works quite well but doesn’t lose an important email this way!!!
Next...
Not satisfied? Well, the next article I will write will describe the way I interpret the information we have got from the server. We will have to deal with the minefield that is MIME. I have been reading information from about five or six different RFC documents and I am about half way through creating a basic implementation. Base64 encoding can be fun, honest! In fact, I am quite pleased with what I have so far, but I think it may go to four articles since it won’t be the simplest task. Then finally I plan to tackle SMTP and sending emails back out on to the internet. Also, after writing this workable POP3 class, I have found out that the IT department where I work uses IMAP mail servers. If I can’t twist their arm to turn POP3 on, I may be writing yet another article.
For the next bit, I decided I will need lots of examples of MIME messages to test my code on. MS Outlook doesn’t seem to allow me to get access to the raw MIME messages like Netscape does so I have wrote a program called ‘save emails’ that uses my class to grab emails from my server in raw form. It won’t delete the messages from the server just saves them in text files.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.