Download demo project - 83 Kb Download 32-bit source - 20 Kb Download 16-bit source - 13 Kb 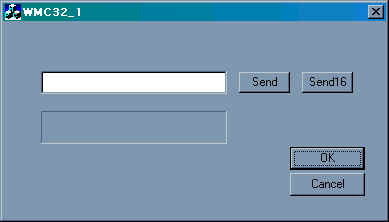
This demo shows how to send and receive message between apps (32-bit and 16-bit) using
WM_COPYDATA.
There are two samples. One is 32-bit and one is 16-bit. The 32-bit sample will check and see whether it is already running and if so, will
start another instance with a different window header.
- The 'Send' button will send the text from the editbox to the other 32-bit app.
- The 'Send16' button will send the text to the 16-bit app.
The received data will show up in the static box.Only 2 instances of the 32-bit apps are supported in this demo. The 16-bit app will
only send and receive data to/from the 32-bit apps.
The exchange of data is performed by finding the other application (using FindWindow) and sending
a WM_COPYDATA message to that window:
CString strWindowTitle = _T("Window Name");
CString strDataToSend = _T("This is a message to send");
LRESULT copyDataResult;
CWnd *pOtherWnd = CWnd::FindWindow(NULL, strWindowTitle);
if (pOtherWnd)
{
COPYDATASTRUCT cpd;
cpd.dwData = 0;
cpd.cbData = strDataToSend.GetLength();
cpd.lpData = (void*)strDataToSend.GetBuffer(cpd.cbData);
copyDataResult = pOtherWnd->SendMessage(WM_COPYDATA,
(WPARAM)AfxGetApp()->m_pMainWnd->GetSafeHwnd(),
(LPARAM)&cpd);
strDataToSend.ReleaseBuffer();
}
else
{
AfxMessageBox("Unable to find other app.");
}
}
The other app should handle the WM_COPYDATA message in the following manner
BEGIN_MESSAGE_MAP(CMyWnd, CWnd)
...
ON_WM_COPYDATA()
...
END_MESSAGE_MAP()
...
BOOL CMyWnd::OnCopyData(CWnd* pWnd, COPYDATASTRUCT* pCopyDataStruct)
{
CString strRecievedText = (LPCSTR) (pCopyDataStruct->lpData);
return CMyWnd::OnCopyData(pWnd, pCopyDataStruct);
}
From firmware in Assembly code to GUIs in C#; done it all.
Currently mainly programming C, C++ at the Windows system level.
Living in Palm Bay, FL.