MSBuild Task Tutorial: Label SourceSafe Project
Create your own MSBuild Task to label SourceSafe projects
Introduction
While attempting to automate my build process using MSBuild, I have been unable to find a MSBuild Task to label projects in Visual SourceSafe. So, I decided to roll my own using C#. Since you are reading this, you are probably aware that MSBuild is the new build platform from Microsoft. If you are unfamiliar with MSBuild, check out the list of resources at the bottom of this page for an introduction.
Tools Involved
During the course of this tutorial, I use the following tools:
- Visual Studio 2005
- Microsoft Visual Studio 2005 Web Deployment Project
- Microsoft Visual SourceSafe 6.0
- MSBuild
Step 1: Create your MSBuild Task Project
Start by opening Visual Studio 2005 and creating a class library project. Name it something clever, like VSSTasks
.
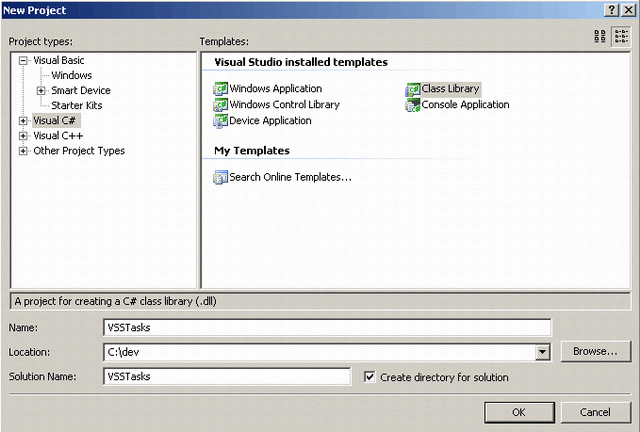
Step 2: Add the Label Project Class
Create a new class file and name it LabelProject.cs.

Add the following code to the LabelProject.cs file:
using Microsoft.Build.Framework;
using SourceSafeTypeLib;
namespace VSSTask
{
public class LabelProject : ITask
{
#region Properties
private IBuildEngine engine;
public IBuildEngine BuildEngine
{
get { return engine; }
set { engine = value; }
}
private ITaskHost host;
public ITaskHost HostObject
{
get { return host; }
set { host = value; }
}
private string vssINIPath;
[Required]
public string VSSINIPath
{
get { return vssINIPath; }
set { vssINIPath = value; }
}
private string vssUserName;
public string VSSUserName
{
get { return vssUserName; }
set { vssUserName = value; }
}
private string vssPassword;
public string VSSPassword
{
get { return vssPassword; }
set { vssPassword = value; }
}
private string vssProjectPath;
[Required]
public string VSSProjectPath
{
get { return vssProjectPath; }
set { vssProjectPath = value; }
}
private string vssLabelText = "";
/// <SUMMARY>
/// Provide a string up to 31 characters long.
/// </SUMMARY>
[Required]
public string VSSLabelText
{
get { return vssLabelText; }
set
{
// only get the first 31 characters
if (value.Length > 0)
vssLabelText = value.Substring(0, 31);
}
}
private string vssCommentText =
"Label generated automatically by MSBuild VSSTask";
public string VSSCommentText
{
get { return vssCommentText; }
set { vssCommentText = value; }
}
#endregion Properties
public bool Execute()
{
// Create SourceSafe ActiveX object
SourceSafeTypeLib.VSSDatabase sourceSafeObject =
new SourceSafeTypeLib.VSSDatabase();
// Open a connection to the SourceSafe database=
// using the srcsafe.ini file and a valid
// userid/password combination.
sourceSafeObject.Open(vssINIPath, vssUserName, vssPassword);
// Get reference to project we wish to label
VSSItem vssProjectItem =
sourceSafeObject.get_VSSItem(vssProjectPath, false);
vssProjectItem.Label(vssLabelText, vssCommentText);
string message = "VSS Label Successfully Set to " + vssLabelText;
BuildMessageEventArgs args = new BuildMessageEventArgs(
message, string.Empty, "SetVSSLabel", MessageImportance.Normal);
engine.LogMessageEvent(args);
sourceSafeObject = null;
vssProjectItem = null;
return true;
}
}
}
Step 3: Add Necessary References
You will need to add two references to your VSSTasks
project. First, add a reference to the Microsoft.Build.Framework
. It should be located under the .NET tab on the Add Reference screen.
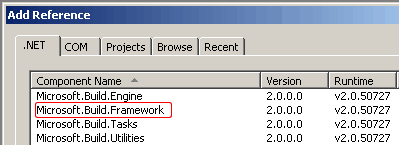
Adding the reference to the Microsoft SourceSafe 6.0 Type Library (SSAPI.DLL), which is installed along with SourceSafe, is slightly more complicated. I assume that you have a local copy of Visual SourceSafe installed. You will need to browse to the SSAPI.DLL file via the Add Reference screen.
In my case, the path to the SSAPI.DLL is:
C:\Program Files\Microsoft Visual Studio\VSS\win32\SSAPI.DLL
Step 4: Implementation
Compile your new VSSTasks
project. Atta boy!
I use Microsoft Visual Studio 2005 Web Deployment Project to create my MSBuild project files. I highly recommend it, if you are automating the build process of an ASP.NET project. Check out the list of resources at the bottom of this page for examples of how to get started with the Web Deployment Project. For the sake of this article, I will assume that you have already added a Web Deployment Project to your own ASP.NET project in Visual Studio 2005.
Inside of your Web deployment project file (*.wdproj), you will add a reference to your newly created LabelProject
task.
<USINGTASK TaskName="VSSTask.LabelProject"
AssemblyFile="C:\dev\VSSTasks\VSSTasks\bin\Debug\VSSTasks.dll" />
Then add the following PropertyGroup
element:
<PROPERTYGROUP>
<VSSINIPATH>C:\Program Files\Microsoft Visual Studio\VSS\srcsafe.ini</VSSINIPATH>
<VSSUSERNAME>IPFreely</VSSUSERNAME>
<VSSPASSWORD>unGuessablePassword</VSSPASSWORD>
<VSSPROJECTPATH>$/MyWebProject</VSSPROJECTPATH>
<VSSLABELTEXT>Testing Label v1</VSSLABELTEXT>
<VSSCOMMENTTEXT>Label generated by MSBuild using LableProject task.</VSSCOMMENTTEXT>
</PROPERTYGROUP>
Finally, add the task you just created to the After Build target element:
<Target Name="AfterBuild">
<LabelProject VSSINIPath="$(VSSINIPath)"
VSSUserName="$(VSSUserName)"
VSSPassword="$(VSSPassword)"
VSSProjectPath="$(VSSProjectPath)"
VSSLabelText="$(VSSLabelText)"
VSSCommentText="$(VSSCommentText)" />
</Target>
Step 5: It's Run Time, Baby!
Build your Web Deployment project either via the Visual Studio 2005 IDE, or you can do so using MSBuild and the command line.
Example:
C:\MyProjects\MyWeb>msbuild MyWeb.sln /p:Configuration=Debug
The output from the build process should include the following text:
VSS Label Successfully Set to Testing LabelProject Class
Step 6: Verify that VSS Project was Labeled
Open Visual SourceSafe. Right click your project and select Properties. Under the General tab, you should see the Last Label text, followed by the text you specified in the VSSLabelText
property in your Web Deployment Project file.

Congratulations, you have just labeled a Visual SourceSafe project using a MSBuild task, created with C# and Visual Studio 2005.
Conclusion
Don't stop now. You are only getting started. Why not create a task to get the latest version of your project from SourceSafe?
Resources
- MSBuild Tutorials and Resources
- MSBuild: How to Write a Task
- I used Novick Software's Creating a Daily Visual SourceSafe (VSS) Label with A SQL Agent Job tutorial, to figure out how to label a Visual SourceSafe project using C#.
You should now see both the Microsoft.Build.Framework
and the SourceSafeTypeLib
in your list of references.
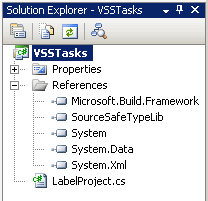
History
- 28th February, 2006: Initial post