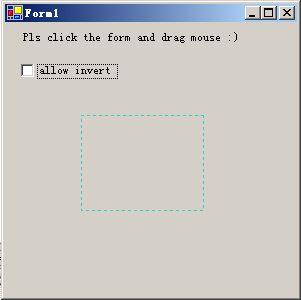
Introduction
A rubber band or focus rectangle is a rectangle that tracks with the mouse pointer while you hold down the left mouse button. The TrackRubberBand method of MFC CRectTracker class allows developers to do that. But .NET has not. I write a simple class RectTraker in C#. It has a same method named TrackRubberBand to help developer track a rectangle rubber band in any .net windows. I hope it's helpful to you. Main source code:
public bool TrackRubberBand(Control control,
Point point, bool allowInvert)
{
bool ok = true;
rect = new Rectangle(point.X, point.Y,
point.X, point.Y);
Graphics g = control.CreateGraphics();
Point pt1, pt2;
pt1 = control.PointToScreen(point);
pt2 = pt1;
DrawRectangle(pt1, pt2);
WinAPI.SetCapture(control.Handle);
while (true)
{
MSG msg = new MSG();
WinAPI.GetMessage(msg, IntPtr.Zero, 0, 0);
switch (msg.message)
{
case WM_MOUSEMOVE:
{
DrawRectangle(pt1, pt2);
if (allowInvert)
pt2 = new Point(msg.x, msg.y);
else
pt2 = new Point(Math.Max(pt1.X, msg.x),
Math.Max(pt1.Y, msg.y));
pt2 = AdjustPt2(pt1, pt2);
DrawRectangle(pt1, pt2);
break;
}
case WM_RBUTTONUP:
case WM_MBUTTONUP:
case WM_LBUTTONUP:
{
goto ExitLoop;
}
case WM_KEYDOWN:
{
if (msg.wParam == VK_ESCAPE)
{
ok = false;
goto ExitLoop;
}
break;
}
case WM_LBUTTONDOWN:
case WM_MBUTTONDOWN:
case WM_RBUTTONDOWN:
break;
}
}
ExitLoop:
DrawRectangle(pt1, pt2);
WinAPI.ReleaseCapture();
g.Dispose();
if (ok)
rect = new Rectangle(pt1,
new Size(pt2.X-pt1.X, pt2.Y-pt1.Y));
return ok;
}
private void DrawRectangle(Point pt1, Point pt2)
{
Rectangle rc = new Rectangle(pt1,
new Size(pt2.X-pt1.X, pt2.Y-pt1.Y));
ControlPaint.DrawReversibleFrame( rc, Color.Red,
FrameStyle.Dashed );
}
To use this class, just like below:
private void Form1_MouseDown(object sender,
System.Windows.Forms.MouseEventArgs e)
{
RectTracker r = new RectTracker();
Point pt = new Point(e.X, e.Y);
r.TrackRubberBand(this, pt, true);
}
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.