SQLite GUI






3.94/5 (23 votes)
A GUI for SQLite in .NET
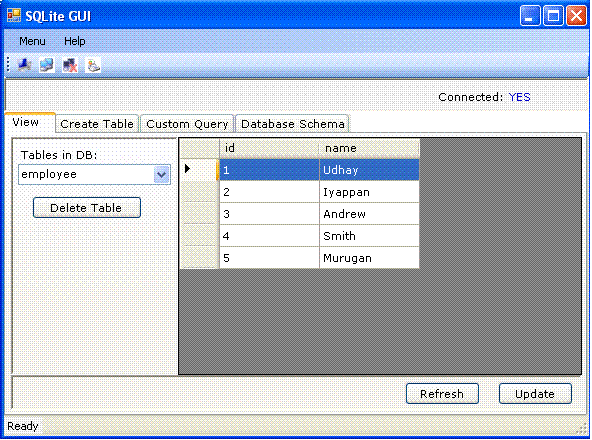
Introduction
This article describes how to create, insert, update SQLite
database from GUI.
Description
SQLite
GUI is a simple GUI for creating a new SQLite
database and opening an existing SQLite
database. It can also create, delete and update tables in the database. All the available tables in the database are shown. The records of various types including BLOB
are displayed in the DataGridView
. And the data in the DataGridView
can be printed. The schema for all the available tables is displayed.
Dependencies
The SQLite.NET
assembly is available here. Add the reference SQLite.Net.dll to the project and use the namespace Finisar.SQLite
to work with SQLite
.
Finisar.SQLite
Finisar.SQLite
is an ADO.NET Data Provider for accessing SQLite
-Databases using the .NET-Framework. In SQLite
, a complete database is stored in a single disk file. SQLite is zero-configuration - no setup or administration needed. SQLite
itself is "a small C library that implements a self-contained, embeddable, zero-configuration SQL database engine" (quoted from the sqlite.org Website). The Finisar.SQLite
Data Provider is an easy way to use the SQLite
-Database in .NET languages like C# and VB.NET.
Sample Code
try
{
//Connection string
string connString = String.Format("Data Source={0};New=False;Version=3", DB_NAME);
//Connecting database
SQLiteConnection sqlconn = new SQLiteConnection(connString);
//Open the connection
sqlconn.Open();
//Query
string CommandText = "Select name from sqlite_master;";
//SQLiteDataAdapter to fill the DataSet
SQLiteDataAdapter dataAdapter = new SQLiteDataAdapter(CommandText, sqlconn);
dataAdapter.Fill(ds);
//Get the collection of rows from the DataSet
DataRowCollection dataRowCol = ds.Tables[0].Rows;
//Add the tables available in the DB to the combo box
foreach (DataRow dr in dataRowCol)
{
tablecombobox.Items.Add(dr["name"]);
}
//Close the Connection
sqlconn.Close();
}
catch (SQLiteException sqlex)
{
MessageBox.Show(sqlex.Message);
}
To Do
Update cannot be performed on table without primary key. The SelectCommand
must also return at least one primary key or unique column. If none are present, an InvalidOperation exception is generated, and the commands are not generated. The alternative way is create select
, insert
and update
command for that particular table. Refer to MSDN. It will be available in the next version of SQLite
GUI.
History
- 10th March, 2007: Initial post