Simple RSS Reader in C#






3.29/5 (7 votes)
Simple RSS Reader in C# using XSL
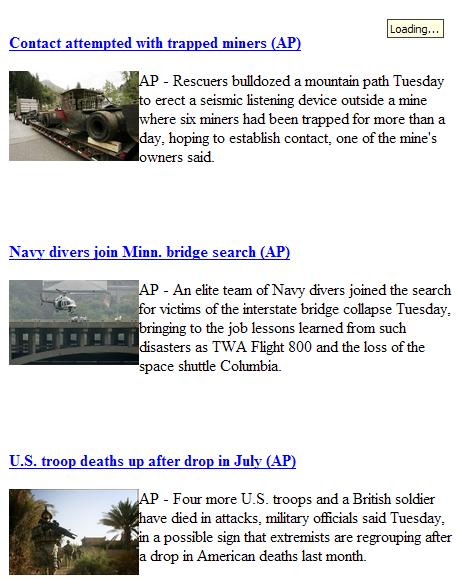
Introduction
This is a simple way of reading an RSS from a feed using C# and XSL.
Using the Code
There are two major parts in this solution. One is the Page that requests and renders the feed and another one will be the XSL which transforms the XML feed.
The first is the default.aspx.cs. We need to import the following references first:
using System.Xml;
using System.IO;
using System.Net;
Then let's go to the fun part:
// Declare your Variables
string xmlsrc = "http://rss.news.yahoo.com/rss/topstories";
string Password = "xxxxxx";
string UserAccount = "xxxxxx";
string DomainName = "xxxxxx";
string ProxyServer = "192.168.1.1:8080";
string xslsrc = "RSS91.xsl";
if (xmlsrc != "")
{
// Make Remote Request
HttpWebRequest wr = (HttpWebRequest)WebRequest.Create(xmlsrc);
if (UserAccount != "")
{
wr.Credentials = new NetworkCredential(UserAccount, Password, DomainName);
}
// Set Proxy Server
if (ProxyServer != "")
{
wr.Proxy = new WebProxy(ProxyServer, true, new string[] { }, wr.Credentials);
}
// Set the HTTP properties
wr.Timeout = 10000;
// 10 seconds
// Read the Response
WebResponse resp = wr.GetResponse();
Stream stream = resp.GetResponseStream();
// Load XML Document
XmlTextReader reader = new XmlTextReader(stream);
reader.XmlResolver = null;
XmlDocument doc = new XmlDocument();
doc.Load(reader);
xmlRSS.Document = doc;
}
xmlRSS.TransformSource = xslsrc;
}
Then the XSL which formats the feed visually. This is how the page will look like the default.aspx just renders it:
<?xml version="1.0"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:output method="html" indent="yes"/>
<xsl:param name="TITLE"/>
<xsl:template match="rss">
<!-- Do not show channel image -->
<xsl:for-each select="channel/item">
<br>
<strong>
<a href="{link}" target="_main">
<xsl:value-of select="title"/>
</a>
</strong>
<br></br>
<!-- only display markup for description if it's present -->
<xsl:value-of select="description" disable-output-escaping="yes"/>
</br>
<br></br>
</xsl:for-each>
</xsl:template>
<xsl:template match="description">
<br>
<xsl:value-of select="."/>
</br>
</xsl:template>
</xsl:stylesheet>
History
- 7th August, 2007: Initial post