Multiple Desktops






4.71/5 (21 votes)
Simple Dialog based Win32 application that provides multiple desktops for windows platform as available in the Linux System
Introduction
This is a simple Win32 Dialog based application that provides multiple desktop support on windows platform as present in the LINUX system. The application provides you the option to create 1 to 3 desktops. Through these different desktops, you can easily separate your applications. This helps a lot when you have some application that you don't want to close by mistake as you can put it on other desktops. The application also shows the last state of each newly created desktop snapshot in the default desktop.
Screen Shot
Following is the screen shot of the window which shows the last state of all the other desktops. You will be able to see this window only when you are in the default desktop(if you are in another desktop, just press WIN + 1 key to switch to the default desktop). Just click on the Tray Icon to see this window. You can go to another desktop by simply clicking on the Image of that desktop.
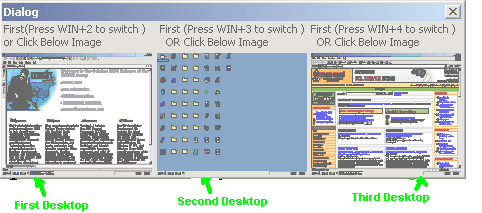
Background
The application uses the CreateDesktop
and SwitchDesktop()
API to create and switch the desktop. It also uses the StretchBlt
API to capture the desktop and to show it.
CreateDesktop
: It creates a new desktopSwitchDesktop
: It makes a desktop visible and activates it. This enables the desktop to receive input from the userStretchBlt
: It is used to copy the source rectangle to the destination rectangle by stretching or compressing it
For better understanding of the above APIs, one can refer to MSDN.
Using the Code
There are three different dialog boxes used in this application. The main Dialog box is used to create and switch the desktop.
The following is the code used in Multidesktop
:
//Check the registry for previous setting.
//It show the Number of desktops user require
INT NoOfDesk = CheckRegValue(_T("NoOfDesk"));
//Put the Application ICON in system Tray
PutInTaskBar(hWndDlg);
//Add the Applications path in the System Startup
AddToRun(AppPath)
//registers the Hot key
AddHotKey(hWndDlg);
//function retrieves a handle to the desktop that receives user input
//i.e. Default Desktop.
hOriginalInput = OpenInputDesktop(0, FALSE, DESKTOP_SWITCHDESKTOP);
// Create new Desktops Depends on Last Setting
for (int i=0 ; i<NoOfDesk; i++)
{
hDesktop[i] = CreateDesktop(DeskName[i], NULL, NULL, 0, GENERIC_ALL, NULL);
}
//Will Open Process in new desktop
//user init process is required to start the explorer and
//initialize the new desktop
//At First time only
if(FirstTime)
{
for (int i=0; i<NoOfDesk; i++)
{
//Will Start the Init Process in Each Desktop
OpenProc(_T("userinit.exe"),DeskName[i]);
}
FirstTime = FALSE;
}
Now we have created the new desktops and saved the handle of the default desktop. Following is the code that shows how to switch to the new desktop. I have used two techniques to switch to the new desktop.
- Switch desktop through Hot Key
I have used four hot keys to switch to and from the new desktop:
- Win + 1 is used to switch to the default desktop
- Win + 2 is used to switch to the first desktop
- Win + 3 is used to switch to the second desktop
- Win + 4 is used to switch to the third desktop
The following code shows how I have done this:
case WM_HOTKEY: { HDESK hNewDesk; //WIN + 1 Pressed Change to Default Desktop if (wParam == GlobalAddAtom(L"First_Desk")) { hNewDesk = hOriginalInput; DeskNo = -1; //WIN + 2 || WIN+ 3 || WIN + 4 Pressed Previously if (OtherKey) { flag = TRUE; OtherKey = FALSE; } else // WIN + 2 || WIN+ 3 || WIN + 4 NOT PRESSED PREVIOUSLY { flag = FALSE; } } //WIN + 2 Pressed Changed to New Desktop if (wParam == GlobalAddAtom(L"Second_Desk")) { hNewDesk = hDesktop[0]; PrvDeskNo = 0; OtherKey = TRUE; } .... //Same for Win + 3 and Win + 4 .... SwitchDesktop( hNewDesk ) //Switch to Desired Desktop. if ( flag ) { //Capture the Screen of Desktop and Show in dialog Box. CaptureScreen(hStaticDesk[PrvDeskNo]); } }
- Switch the desktop when you click on the desktop screen shot.
The following code shows how I have done this:
case WM_COMMAND: if (LOWORD(wParam) == IDC_Desk2 ) { SwitchDesktop( hDesktop[0] ); OtherKey = TRUE; PrvDeskNo = 0; break; } ... ... //almost same for other Click Events.
Points of Interest
I am working in a software company where our local site has all the songs listed and the software that plays it. But as it opens as a web browser, most of the time, people shut it down by mistake when they are surfing. One of my friends asked me if I could help him, and I came up with the idea of having a software that provides multiple desktops. Rather than downloading it from the Internet, I thought it would be better to develop my own. Then I came across this beautiful and useful application.
History
- Submitted on 27th October, 2007