Ajax Streaming Sample






1.67/5 (2 votes)
Nov 24, 2007
2 min read

42220

290
An article on how to implement AJAX
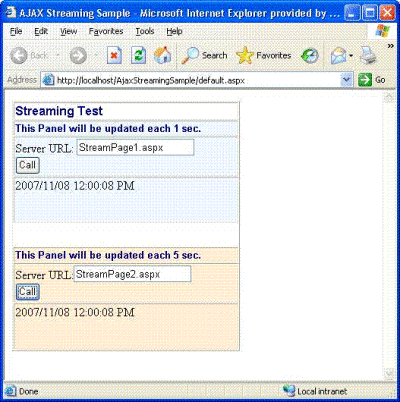
Introduction
In one of my ASP.NET 1.1 projects, I had to update two different controls on a page through asynchronous AJAX calls at different time intervals.
As a sample to simulate this type of behavior, I decided to create a web page with two labels having Date/Time stamp. One of those labels should be updated every second and another one should be updated every 5 seconds.
In my sample I have the base page with two labels that calls HTML data from two other pages to populate the labels with the current Date/Time at different time intervals through asynchronous AJAX calls.
This article is an attempt to provide a good working sample of such an approach.
Background
XmlHttpRequest
is an object using which you can send/get any data to/from the server using JavaScript.
To implement an AJAX call, you actually need to have two web pages: the one that is visible to the end user and the second one that actually generated the required content for the first web page. The first page calls the second one through XmpHttpRequest
implemented in JavaScript.
Using the Code
In the sample below, we will have three web pages:
- default.aspx - The page that will be shown to the end user
- StreamPage1.aspx - This will provide the content to the section of default.aspx page that will be updated every second
- StreamPage2.aspx - This will provide the content to the section of default.aspx page that will be updated every 5 seconds.
In the provided sample, StreamPage1.aspx and StreamPage2.aspx web pages are the same and just have one label - Label1
that shows the current date/time on Page_Load
event:
private void Page_Load(object sender, System.EventArgs e)
{
Label1.Text=DateTime.Now.ToString();
}
The default.aspx page is also simple and just provides two sections with the text box with the calling web page name, the input button that calls the JavaScript function doCall1()
or doCall2()
and the <DIV id='panel1'></DIV>
and <DIV id='panel2'></DIV>
sections that will actually accept the HTML return by StreamPage1.aspx or StreamPage2.aspx through XmlHttpRequest
.
Let's consider in more detail the major part of the project - AJAX.js.
// Creating the instance of the XmlHttpRequest
// This is done differently for different browsers
// Branch for native XMLHttpRequest object
var client=null;
if (window.XMLHttpRequest)
{
client = new XMLHttpRequest();
}
// Branch for IE/Windows ActiveX version
else if (window.ActiveXObject)
{
client = new ActiveXObject("Microsoft.XMLHTTP");
}
//Sending information to server
function doCall1(datenow)
{
try
{
// Here we provide the URL of the web page that will provide
// the content for the main page;
var url=document.getElementById("txtStreamingPage1").value
// The parameter ?d= in the next line of code is important
// Without it the event - onreadystatechange - will never happen
url=url+'?d='+datenow client.open("GET", url);
client.onreadystatechange = callBack1;
client.send();
}
catch(ex)
{
alert(ex.message);
}
// This sets the time interval for 1 second
setTimeout('doCall1(Date())', 1000);
}
// Waiting and processing server response
function callBack1(response)
{
try
{
if(client.readyState == 4 && client.status == 200)
{
document.getElementById("panel1").innerHTML=client.responseText;
}
}
catch(ex)
{
alert(ex.message);
}
}
The function doCall2()
works the same, but it is called on the 5 seconds interval:
// This sets the time interval for 5 seconds
setTimeout('doCall2(Date())', 5000);
Points of Interest
Using the above technique, it is possible to update various parts of the page with different data asynchronously. This can be useful when working with data that should be refreshed to the user not only when the user posts back the page, but rather at certain time intervals.