Easy XML Parsing in C#





4.00/5 (13 votes)
The easiest way to get XML parsing in C#
Introduction
Suppose you have an XML Schema with XML files that satisfy it and you need to get data out of the XML files in a way that is easy to read and maintain. There are quite a few ways to approach this problem in any language (C# included). The XmlSerializer
is the one stop shop for quick and easy XML parsing in C#. The purpose of this article it to provide an overview to generating C# object bindings for a schema and parsing an XML file using those bindings. This is accomplished by demonstrating this practice using two different sets of XML Schemas and XML files.
Why Not Use XmlNode / XMLDocument?
The readability and maintainability of your XML parsing code can be improved using the methods outlined in this article. Say you were given a baseline of code and were told that it was receiving a NullReferenceException
on the following line:
string s = xmlnode[x].LastChild.InnerText;
You may probably start by debugging to step through and verify xmlnode
, xmlnode[x]
and LastChild
were valid. You may find out that your XML file does not contain a LastChild
for a given xmlnode[x]
. You will need to dig through the schema manually, verify what the xmlnode[x]
s are all about and start playing XML / code detective. The problem here is, from the code itself, you have no real guidance as to what structure you are dealing with. Now, suppose you were given the following line:
string s = shiporder.Items[1].quantity;
This line gives you more insight into what your code is meaning to do. In addition, it provides the reader with a definition of the structures these operations will be performed on; no detective work required.
Background
In order to use the following samples, you will need to have a working copy of Visual Studio 2005 or 2008. If you are unfamiliar with the xsd.exe utility distributed with these environments, I would recommend running a /? at the command prompt to get familiar with it. In addition, a basic understanding of XML and XML Schema documents is always handy. The two XML schemas used for this article were obtained from here.
Using the Code
The code provided in csharpxmlparsing.zip contains two example schemas, XML files and test code. Two examples provided demonstrate how different schemas look and feel when writing the parsing code. Here's a rundown on the contents.
The following files relate to the notes
example:
notes.xml - notes data file
notes.xsd - notes schema
notesmain.cs - What you would have to write to parse notes XML files
The following files relate to the shiporder
example:
shipmain.cs - What you would have to write to parse shiporder xml files
shiporder.xml - shiporder data file
shiporder.xsd - shiporder schema
The two files that represent what you will need to write if you would need to parse the XML can be found in notesmain.cs and shipmain.cs. Notesmain.cs has been provided here to give you a reasonably good example of what the parsing code looks and feels like:

So, how do you use these examples? Perform the following steps:
- Unzip the csharpxmlparsing.zip file to a desired location
- Start up a Visual Studio 2005/2008 Command Prompt
- cd to the path (location) where you uncompressed csharpxmlparsing.zip
- Run the file named go.bat
This step will produce two new files named notes.cs and shiporder.cs. These are the C# classes that are used to represent the structure of the provided schemas. These files should never be modified by hand. In addition, two executable files will be produced, named: notes.exe and shipmain.exe. After being built, these two executable files are then executed, so their output can be displayed. The output should look similar to that found below:
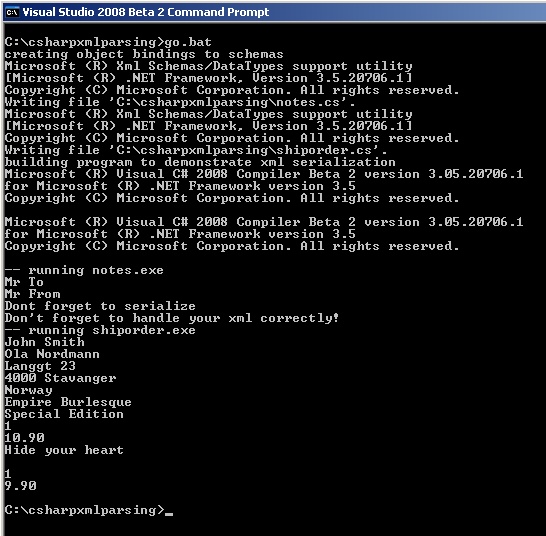
Points of Interest
What happens if your schema changes? Using this method, simply regenerate your object bindings using the xsd.exe utility and modify your code to support old structures that have changed or new structures introduced.
History
- 2nd March, 2008: Initial post