jQuery for Beginners






4.81/5 (80 votes)
This article helps you to understand the JQuery with examples.
Introduction
jQuery is a JavaScript library which has a wide range of actions such as event handling, animation, HTML document traversing, and AJAX interaction for web development. jQuery simplifies JavaScript programming.
$(document).ready Method
The document ready event executes already when the HTML-Document is loaded and the DOM is ready, even if all the graphics are not loaded yet.
$(document).ready(function() {
alert("document is ready");
});
Selectors
jQuery provides a simple way to select single element or group of elements. You can access element by type (div
, span
, p
), id, CSS class and attribute, etc. I have explained the basic selectors here. You can find some other selectors in the attached project with examples.
jQuery | Example Code | Description |
$('element') |
$('blockquote').css('color','red'); |
It selects all elements with the given tag name. In this example, it will return all <blockquote> elements in the document. |
$('#id') |
$('#item1').css('color','red'); |
It selects single element with the given id. If more than one element has been assigned the same id, first match result will be returned. |
$('.class') |
$('.myCss').css('color','red'); |
It selects all the elements with given class. |
Sliding Effect
jQuery provides three methods to show or hide elements in sliding behavior.
SlideDown(speed, callback)
: This method gradually increases the height of the elements, from hidden to visible.SlideUp(speed, callback)
: This method gradually decreases the height of the elements, from visible to hidden.SlideToggle(speed, callback)
: This method toggles betweenSildeUp()
andSlideDown()
for selected elements.
All three methods has "Speed
" and "callback
" parameters.
The Speed
parameter can have the following values:
- slow
- normal
- fast
- milliseconds, e.g., 100, 500, 1000, etc.
The callback parameter is the name of a function that executes after the function completes.
Sliding Example
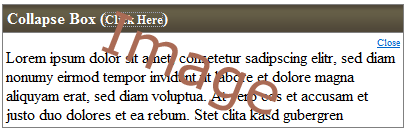
According to your website design, decide collapsible Area and element on which you will handle Slide
behavior. In this example, I created Box
using DIV
s and choose DIV
having id “contentArea
” for sliding.
<div class="box" style="width: 400px">
<div class="title">
Collapse Box (<a id="lnkCollapse"
href="#" style="color: White; font-size: 12px;
text-decoration: none;">Click Here</a>)
</div>
<div id="contentArea" class="content">
<div align="right"><a id="lnkClose"
href="#" style="font-size: 10px;">
Close</a></div>
Your text will be here
</div>
</div>
Register click event of the HTML element and call SlideToggle
and SlidUp
method for the “contentArea
”. More details of this example are available in the attached project.
$(document).ready(function () {
$("#lnkCollapse").click(function () {
$("#contentArea").slideToggle("slow");
});
$("#lnkClose").click(function () {
$("#contentArea").slideUp(200);
});
});
Fade Effect
jQuery also provides four methods to gradually change the opacity of the selected element using Fade effect.
fadeTo(speed, opacity, callback)
: This method changes the opacity of selected elements to specified opacity.fadeIn(speed, callback)
: This method gradually increases the opacity of the elements, from hidden to visible.fadeOut(speed, callback)
: This method gradually decreases the opacity of the elements, from visible to hidden.fadeToggle(speed, callback)
: This method toggles betweenFadeIn()
andFadeOut()
for selected elements.
In this example, I will set the opacity of the image on hover. Add images in to the DIV
and assign id “fadeExp1
” to div
.
<div id="fadeExp1">
<img src="Images/b1.jpg" width="100px" height="100px" />
<img src="Images/b2.jpg" width="100px" height="100px" />
<img src="Images/b3.jpg" width="100px" height="100px" />
</div>
In ready()
function, set the default opacity of all images using $(“#fadeExp1 img”).fadeTo(0, 0.3);
statement. Then register Hover
event on each image and provide two functions in it, one for mouse over and one for mouse out and set the opacity of element there. See example code below:
$(document).ready(function () {
$("#fadeExp1 img").fadeTo(0, 0.3);
$("#fadeExp1 img").hover(function () {
$(this).fadeTo("slow", 1.0);
},
function () {$(this).fadeTo("slow", 0.3);
}
);
});
Animation Effect
jQuery also provides an easy way to animate element. The syntax of the animate
method is .animate( properties, [duration], [easing], [complete])
.
properties
: A map of CSS properties, which changes during animationduration
:String
or number to determine the duration of the animationeasing
: The name of easing function to use for the transitioncomplete
: A function to call on the complete of animation
A very cool and simple example for the icons list in your website, simply register hover event on the image. On mover over, set top=-15
and on mouse out set top=0
again.
$("#divSocial a img").hover(
function () {
$(this).animate({ top: "-15" });
},
function () {
$(this).animate({ top: "0" });
}
);
Don’t forget to set the relative position of the image.
#divSocial a img
{
position: relative;
border: none;
}
Create Tab Control
You can easily create a sophisticated tab control using jQuery. Let's start learning how can we quickly create a tab control. Create Tab Header or tabs by assigning IDs to each element as I assigned “tab1
”, “tab2
” and “tab3
”. Set first element as selected by assigning CSS class “selectTabHeader
”.
Now create Tab content area. I created separate div
s for each tab content. Assign same id “tab-data
” to content div
as I assigned in given example and also assign dummy CSS class to div
having same id as parent tab button contains. I assign CSS class “tab1
”, “tab2
” and “tab3
”.
<div id="tabArea">
<div id="tabHeader">
<a id="tab1" class="tab selectTabHeader">Tab 1 </a>
<a id="tab2" class="tab">Tab 2</a>
<a id="tab3" class="tab">Tab 3 </a>
</div>
<div id="tabData">
<div id="tab-data" class="tab1 selectedTab">
tab 1 data
</div>
<div id="tab-data" class="tab2">
tab 2 data
</div>
<div id="tab-data" class="tab3">
tab 3 data
</div>
</div>
</div>
By default, hide all tab content area using CSS and display only selected tab contents.
#tab-data
{
display: none;
}
#tab-data.selectedTab
{
display: block;
color: black;
background: white;
height: 400px;
padding: 5px;
}
Register click event of all header buttons. On the click of element, remove “selectTabHeader
” CSS class from last selected element and then assign selected CSS class to clicked element by using command.
$('.selectTabHeader').removeClass('selectTabHeader');
$(this).addClass('selectTabHeader');
Use the same technique for content area. Remove CSS class “selectedTab
” from last selected tab and assign this class to content area of selected element.
Simply get the id of click element. Suppose user clicked on “tab2
”. Remove the class "selectedTab
" of last selected content using command.
var v = this.id;
$('.selectedTab').removeClass('selectedTab');
$('.' + v).addClass('selectedTab');
Now you have to show the content area of the selected element. Simply find the HTML element which has the CSS class same as the id of selected element. Suppose “tab2
", assign "selectedTab
" CSS class to that element.
$(document).ready(function () {
$('#tabHeader a').click(function () {
// Set header
$('.selectTabHeader').removeClass('selectTabHeader');
$(this).addClass('selectTabHeader');
// Show Actual area
var v = this.id;
$('.selectedTab').removeClass('selectedTab');
$('.' + v).addClass('selectedTab');
});
});
Create Carousel
After reading previous topics, now you have enough knowledge to create advance UI controls. In this example, I will explain how to create a Carousel, which will change the images in sliding style. User can also change image using navigation buttons and also he can directly go to image using number options. Write the HTML as given below:
<div class="advanceCarousel">
<div class="carouselWindow">
<div class="imageReel">
<div class="image">
<a href="#" target="_blank" >
<img src="images/b1.jpg" />
</a>
<div class="description">
Here is a description of first image
</div>
</div>
<div class="image">
<a href="#" target="_blank" >
<img src="images/b2.jpg" />
</a>
<div class="description">
Description of second image
</div>
</div>
<div class="image">
<a href="#" target="_blank" >
<img src="images/b3.jpg" />
</a>
<div class="description">
I am going to write 3rd image.
</div>
</div>
</div>
</div>
<div class="paggingBar">
<div style="float: left;">
<a class="aNav" href="javascript:RotatePrevious();"><<</a>
</div>
<div style="float: right;">
<a class="aNav" href="javascript:RotateNext();">>></a>
</div>
<div class="paging">
<a href="#" rel="1">1</a>
<a href="#" rel="2">2</a>
<a href="#" rel="3">3</a>
</div>
</div>
</div>
In the ready
function, assign active CSS class to the first element. After that, determine the width of the total reel. Get the width of the window and then get the total number of images. Multiply the Width by size, you will get the total width of the reel. Then assign this width to the imageReel
CSS class.
$(".paging a:first").addClass("active");
//Get size of images, number of images, Determine the size of the image reel.
var imageWidth = $(".carouselWindow").width();
var imageSum = $(".imageReel .image img").size();
var imageReelWidth = imageWidth * imageSum;
//Adjust the image reel to its new size
$(".imageReel").css({ 'width': imageReelWidth });
Image rotating method also has very simple logic. You just need to determine the left position of the Image reel. So get the index of the active image. Multiply it with image width to determine to slide distance. Then animate the left CSS property.
rotate = function () {
var triggerID = $active.attr("rel") - 1; //Get number of times to slide
var image_reelPosition = triggerID * imageWidth; //Determines the distance
//the image reel needs to slide
$(".paging a").removeClass('active'); //Remove all active class
$active.addClass('active');
//Slider Animation
$(".imageReel").animate({
left: -image_reelPosition
}, 500);
};
startRotation()
is a method which kicks off the rotation. It selects the next active element after some interval and calls rotate()
to display this active image.
function startRotation() {
play = setInterval(function () {
$active = $('.paging a.active').next();
if ($active.length == 0) {
$active = $('.paging a:first'); //go back to first
}
rotate(); //Trigger the paging and slider function
}, 7000); //Timer speed in milliseconds
}
Register click event on the page number, when user will click, select it as active element and immediately slide to that image.
$(".paging a").click(function () {
$active = $(this); //Activate the clicked paging
//Reset Timer
RotateImmediate();
return false; //Prevent browser jump to link anchor
});
Slide next and previous functionality is also very simple. Get current element and find its next or previous where you want to navigate and after selecting call RoatateImmediate()
method.
function RotateNext() {
var next = $('.paging a.active').next();
if (next.length > 0) {
$active = next;
RotateImmediate();
}
}
function RotatePrevious() {
var next = $('.paging a.active').prev();
if (next.length > 0) {
$active = next;
RotateImmediate();
}
}
History
- 28th August, 2011: Initial post.