Simple Example of MVC (Model View Controller) Design Pattern for Abstraction
Simple example of MVC (Model View Controller) design pattern for abstraction

Introduction
Model-view-controller (MVC) is a pattern used to isolate business logic from the user interface. Using MVC, the Model represents the information (the data) of the application and the business rules used to manipulate the data, the View corresponds to elements of the user interface such as text, checkbox items, and so forth, and the Controller manages details involving the communication between the model and view. The controller handles user actions such as keystrokes and mouse movements and pipes them into the model or view as required.
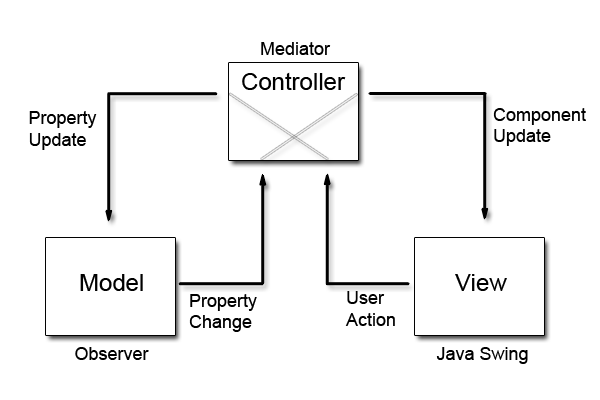
Background
Using the Code
Note: I strongly recommend you download the code to view it, it will be much easier.
Here I will show an example of our good old friend calculator in a MVC architecture. A brief overview; the Form will house the view and events will be passed to the controller who will then call methods on our model such as ADD/Subtract/NumberPress. The model takes care of all the work and it holds the current state of the calculator. The tough thing about MVC is where to slice it apart can be confusing. The end goal is a pluggable UI and perhaps multiple controllers attached to the same model. So one way to test if you did it right is to quickly write another UI and plug it in.
A typical MVC patterns instantiation looks something like the following. A few important things to notice; the controller takes an interface to the view and model. It is important to know that the view will typically interact with the controller if it needs notification of events which are fired via the view (such as a button click). In this case, I have the controllers constructor pass a reference to itself to the view class.
static class Program
{
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
// Note: The view should not send to the model but it is often useful
// for the view to receive update event information from the model.
// However you should not update the model from the view.
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
frmCalcView view = new frmCalcView();
CalculatorModel model = new CalculatorModel();
CalcController controller = new CalcController(model, view);
Application.Run(view);
}
}
/// <summary>
/// The controller process the user requests.
/// Based on the user request, the Controller calls methods in the View and
/// Model to accomplish the requested action.
/// </summary>
class CalcController : IController
{
ICalcModel model;
ICalcView view;
public CalcController( ICalcModel model, ICalcView view)
{
this.model = model;
this.view = view;
this.view.AddListener(this); // Pass controller to view here.
}
public void OnClick( int number )
{
view.Total = model.SetInput(number).ToString();
}
public void OnAdd()
{
model.ChangeToAddState();
}
}
Notice that the view does not interact with the model, it simply receives update requests from the controller. The controller will access the view through the Total property. The view also passes click events on to the controller.
This View shouldn't know about the Controller, except we need to give it notification of some events so we pass in a IController
. We will invoke event handlers on the controller via IController
.
/// <summary>
/// Windows Form that will host our MVC based functionality.
///
/// </summary>
public partial class frmCalcView : Form, ICalcView
{
IController controller;
public frmCalcView( )
{
InitializeComponent();
}
/// <summary>
/// The view needs to interact with the controller to pass the click events
/// This could be done with delegates instead.
/// </summary>
/// <param name="controller"></param>
public void AddListener( IController controller )
{
this.controller = controller;
}
private void lbl_Click(object sender, EventArgs e)
{
// Get the text out of the label to determine the letter and pass the
// click info to the controller to distribute.
controller.OnClick((Int32.Parse(((Label)sender).Text)));
}
private void lblPlus_Click(object sender, EventArgs e)
{
controller.OnAdd();
}
#region ICalcView Members
public string Total
{
get
{
return textBox1.Text;
}
set
{
textBox1.Text = value;
}
}
#endregion
}
Now we will take a look at the model. Notice that it should do the "work" of the calculator and it handles the state.
/// <summary>
/// Calculator model, The model is independent of the user interface.
/// It doesn't know if it's being used from a text-based, graphical, or web interface
/// This particular model holds the state of the application and the current value.
/// The current value is updated by SetInput
/// </summary>
class CalculatorModel : ICalcModel
{
public enum States { NoOperation, Add, Subtract };
States state;
int currentValue;
public States State
{
set { state = value; }
}
public int SetInput ( int number )
{
if (state == States.NoOperation)
{
currentValue = number;
}
else if (state == States.Add)
{
currentValue = Add(currentValue , number );
}
return currentValue;
}
public void ChangeToAddState()
{
this.state = States.Add;
}
public int Add( int value1, int value2 )
{
return value1 + value2;
}
public int Subtract(int value1, int value2)
{
throw new System.ApplicationException(" Not implemented yet");
}
}
Other Information
MVC is often seen in web applications, where the view is the actual HTML page, and the controller is the code that gathers dynamic data and generates the content within the HTML. Finally, the model is represented by the actual content, usually stored in a database or XML files, and the business rules that transform that content based on user actions.
Though MVC comes in different flavors, control flow generally works as follows:
- The user interacts with the user interface in some way (e.g. presses a button).
- A controller handles the input event from the user interface, often via a registered handler or callback.
- The controller notifies the model of the user action, possibly resulting in a change in the model's state. (e.g. controller updates user's shopping cart).
- A view uses the model (indirectly) to generate an appropriate user interface (e.g. the view produces a screen listing the shopping cart contents). The view gets its own data from the model. The model has no direct knowledge of the view.
- The user interface waits for further user interactions, which begins the cycle anew.
By decoupling models and views, MVC helps to reduce the complexity in architectural design, and to increase flexibility and reuse.
History
- 8th April, 2008: Initial post