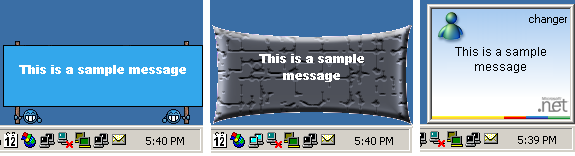
Introduction
A few weeks ago, I saw an MSN messenger popup class on CodeProject written by Prateek Kaul. Since I needed a taskbar popup with skin support, I decided to write my own new class.
This is my first submission to Codeproject, since I think my code begins to be mature enough to be posted.
Compatibility
This class needs MFC to be either statically or dynamically linked in your project; it has been written, compiled and tested under Visual Studio .NET (but should work with VC6).
How to Use the Class
The class is very easy to use: Just add TaskbarNotifier.h and TaskbarNotifier.cpp into your project, then include TaskbarNotifier.h into your application, add a CTaskbarNotifier
member variable in the header file of your Dialog
or Window
class.
In the OnInitDialog
or OnCreate
member functions, add the following lines:
m_wndTaskbarNotifier.Create(this);
m_wndTaskbarNotifier.SetSkin(IDB_MY_BITMAP_RESOURCE);
m_wndTaskbarNotifier.SetTextFont("Arial",90,TN_TEXT_NORMAL,TN_TEXT_UNDERLINE);
m_wndTaskbarNotifier.SetTextColor(RGB(0,0,0),RGB(0,0,200));
m_wndTaskbarNotifier.SetTextRect(CRect(10,40,m_wndTaskbarNotifier1.m_nSkinWidth-10,
m_wndTaskbarNotifier1.m_nSkinHeight-25));
Then when you want to show the popup animation, just call anywhere m_wndTaskNotifier.Show("Text to display");
this will show the animation of the window appearing and then disappearing. You can call again the Show()
method whenever you want. If the popup was still here, the text is just replaced, if it was disappearing, it maximizes again.
Be sure not to allocate the CTaskbarNotifier
each time you want to show a message, because the skinning function takes a little CPU time to generate the region used to make a non rectangular window.
Class Documentation
int Create(CWnd *pWndParent);
Creates the popup window which remains hidden until Show()
is called.
BOOL SetSkin(UINT nBitmapID,short red=-1,short green=-1,short blue=-1);
BOOL SetSkin(LPCTSTR szFileName,short red=-1,short green=-1,short blue=-1);
Those two functions assign a skin to the popup, they take as parameter either a Bitmap
resource ID or the path of a bitmap file.
The optional parameters are the RGB values for the transparency color for the bitmap. Use these parameters only if you want a non rectangular window; if these parameters are left blank, no Region is created.
void SetTextFont(LPCTSTR szFont,int nSize,int nNormalStyle,int nSelectedStyle);
This function permits to specify the Font used for displaying the text (two styles are possible, one for the normal state and one when the mouse is over the window)
nNormalStyle
or nSelectedStyle
can be one or a combination of these parameters:
TN_TEXT_NORMAL
TN_TEXT_BOLD
TN_TEXT_ITALIC
TN_TEXT_UNDERLINE
void SetTextColor(COLORREF crNormalTextColor,COLORREF crSelectedTextColor);
This function sets the color of the text when in normal or selected state.
void SetTextRect(RECT rcText);
This function allows to define a rectangle within the bitmap
which will be the clipping zone for displaying the text.
void Show(LPCTSTR szCaption,DWORD dwTimeToShow=800,DWORD dwTimeToStay=3000,
DWORD dwTimeToHide=500,int nIncrement=1);
Displays the popup window animation:
szCaption
: Text to display dwTimeToShow
: Duration in milliseconds for the popup to be fully visible dwTimeToStay
: Duration in milliseconds for the popup to be stay visible dwTimeToHide
: Time in millisecond for the popup to completely disappear nIncrement
: Pixel increment for moving the window during the animation (the higher, the faster)
Show can be called even if the popup is already visible, being hiding or showing.
void Hide();
Manually hides the popup.
Additional Notes
When the user clicks on the popup, a message is being sent to its parent window (WM_TASKBARNOTIFIERCLICKED
defined in TaskbarNotifier.h), you can intercept it by adding in your Message Map macro:
ON_MESSAGE(WM_TASKBARNOTIFIERCLICKED,OnTaskbarNotifierClicked)
The fact I'm not releasing the HRGN
handle is because when you call SetWindowRgn()
function, the GDI takes care of the object deletion.
Conclusion
Thanks to Prateek Kaul whose code inspired me to write this class and to Vander Nunes for his article about skinning a window.
I hope this class will be useful to you, if you find any memory or GDI leaks, please let me know. If you have suggestions to enhance the functionalities of this class, please post a comment.
License
This article has no explicit license attached to it, but may contain usage terms in the article text or the download files themselves. If in doubt, please contact the author via the discussion board below.
A list of licenses authors might use can be found here.
I live in Santa Clara CA and work as a software engineer for SAP Business Objects.
My areas of expertise are user interface developments in Eclipse RCP / SWT / Draw 2D and C#