How to retrieve a visitor's IP address
Every visitor to your site or web application has an IP address. It is quite handy to be able to get that address. It can be used for security logging, or perhaps tracing. It can also be used to determine where they are in the world, or at least where their ISP is.
The difficulty is when they are behind a proxy of some sort, and you can only see the IP address of the proxy server. So, here are the code snippets in ASP.NET that first check for an IP addresses that's forwarded from behind a proxy, and if there's none, then just gets the IP address. Here's the same IP retriever with proxy detection in .NET, but in VB.NET:
Dim nowip As String
nowip = Request.ServerVariables("HTTP_X_FORWARDED_FOR")
If nowip = "" Then
nowip = Request.ServerVariables("
End If
How do I convert an IP address to an IP number?
IP address (IPV4) is divided into four sub-blocks. Each sub-block has a different weight number, each powered by 256. The IP number is being used in the database because it is efficient to search between a range of numbers in the database.
The beginning IP number and the ending IP number are calculated based on the following formula:
IP Number = 16777216*w + 65536*x + 256*y + z (1)
where:
IP Address = w.x.y.z
For example, if the IP address is "202.186.13.4", then its IP number is "3401190660" based on the above formula.
IP Address = 202.186.13.4
So, w = 202, x = 186, y = 13 and z = 4
IP Number = 16777216*202 + 65536*186 + 256*13 + 4
= 3388997632 + 12189696 + 3328 + 4
= 3401190660
To reverse the IP number to the IP address:
w = int ( IP Number / 16777216 ) % 256
x = int ( IP Number / 65536 ) % 256
y = int ( IP Number / 256 ) % 256
z = int ( IP Number ) % 256
where, %
is the mod operator and int
returns the integer part of the division.
How do I retrieve the country name and the country code from the IP number?
Search the IP-country database to match a unique record that has the IP number that fits between the beginning IP number and the ending IP number.
For example, the IP address "202.186.13.4" is equivalent to the IP number "3401190660". It belongs to the following record in the database because it is between the beginning and the the ending of the IP number:
"3401056256","3401400319","MY","MALAYSIA"
From the recordset, the country name is Malaysia and the country code is MY. Here is a useful link.
How do I use this database
CSV file format
The CSV file contains four fields:
- Beginning of the IP address range
- Ending of the IP address range
- Two-character country code based on ISO 3166
- Three-character country code based on ISO 3166
- Country name based on ISO 3166
"0033996344","0033996351","GB","GBR","UNITED KINGDOM"
"0050331648","0083886079","US","USA","UNITED STATES"
"0094585424","0094585439","SE","SWE","SWEDEN"
Field | Data Type | Field Description |
IP_FROM | NUMERICAL (DOUBLE) | Beginning of the IP address range. |
IP_TO | NUMERICAL (DOUBLE) | Ending of the IP address range. |
COUNTRY_CODE2 | CHAR(2) | Two-character country code based on ISO 3166. |
COUNTRY_CODE3 | CHAR(3) | Three-character country code based on ISO 3166. |
COUNTRY_NAME | VARCHAR(50) | Country name based on ISO 3166 |
Download CSV database
Convert to Access database
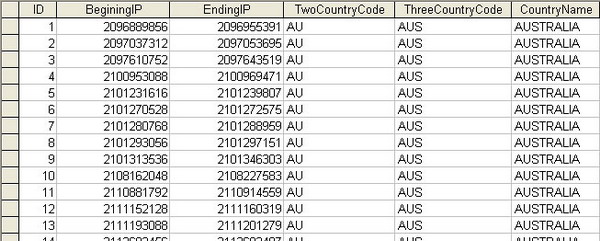
Here is the code-behind:
Partial Class How_to_Convert_IP_Address_Country _
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object,_
ByVal e As System.EventArgs) Handles Me.Load
Dim nowip As String
nowip = Request.ServerVariables("HTTP_X_FORWARDED_FOR")
If nowip = "" Then
nowip = Request.ServerVariables("
End If
If txtIPAddress.Text = "" Then
txtIPAddress.Text = nowip
End If
lblError.Text = ""
End Sub
Protected Sub Button1_Click(ByVal sender As Object,_
ByVal e As System.EventArgs) Handles Button1.Click
On Error GoTo HandleError
Dim dottedip As String
Dim Dot2LongIP As Double
Dim PrevPos As Double
Dim pos As Double
Dim num As Double
dottedip = txtIPAddress.Text
For i = 1 To 4
pos = InStr(PrevPos + 1, dottedip, ".", 1)
If i = 4 Then
pos = Len(dottedip) + 1
End If
num = Int(Mid(dottedip, PrevPos + 1, pos - PrevPos - 1))
PrevPos = pos
Dot2LongIP = ((num Mod 256) * (256 ^ (4 - i))) + Dot2LongIP
Next
txtIPNumber.Text = Dot2LongIP
HandleError:
lblError.Text = Err.Description
End Sub
End Class
Here is the output:

This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.