Google Maps StreetView






4.38/5 (5 votes)
A user friendly way of using the Google Maps streetview
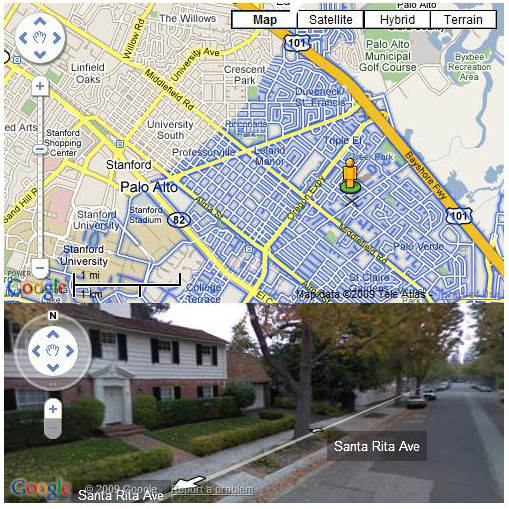
Introduction
Most Internet applications now use Google Maps. Google has provided a very rich API to use in our own applications. For the Google Maps, the latest feature is the "Panoroma view". Using it, I have created this Google Maps example. In this example, when the Google StreetView Man is dragged, the streets are highlighted wherever street view is enabled by Google. When it is dropped, the normal map is displayed. This way, the maps will be more little user friendly. This example uses JavaScript to call the Google Maps API.
Using the Code
In this part of the article, I will describe how to load Google Maps into an HTML page. Within the head
section of an HTML document, include this script reference to the Google Maps API; the key should be changed if you need to upload this HTML in to a web server.
<script
src="http://maps.google.com/maps?file=api&v=2&key=abcdefg&sensor=true_or_false"
type="text/javascript"></script>
Within the same HTML section, add the JavaScript function below. This function calls a Google map and displays it in a <div id="map_canvas"...
.
<script type="text/javascript">
var marker = null;
var myPano = null;
function initialize() {
if (GBrowserIsCompatible()) {
map = new GMap2(document.getElementById("map_canvas"));
map.setCenter(new GLatLng(37.4419, -122.1419), 13);
map.setUIToDefault();
}
}
</script>
The HTML document should have <div id="map_canvas"...
and from the body onload
, the initialize()
method should be called. Here is the full listing of code for loading a Google map into an HTML document.
<html>
<head>
<script
src="http://maps.google.com/maps?file=api&v=2&key=abcdefg&sensor=true_or_false"
type="text/javascript"></script>
<script type="text/javascript">
function initialize() {
if (GBrowserIsCompatible()) {
map = new GMap2(document.getElementById("map_canvas"));
map.setCenter(new GLatLng(37.4419, -122.1419), 13);
map.setUIToDefault();
}
}
</script>
</head>
<body onload="initialize()">
<div id="map_canvas" style="width: 500px; height: 300px"></div>
</body>
</html>
From this point, I will describe how to add panorama view to the above Google map. To add panorama view, we need to add another <div id="stview"...
to the HTML document, and the JavaScript should be change accordingly.
Adding a div
tag into the HTML body
section:
<div id="stview" style="width: 500px; height: 200px"></div>
The next step is to create a draggable marker on the map. The code for creating and adding a Google maker is listed here:
var latlang = map.getCenter();
var icon = new GIcon();
var imageUrl = "http://maps.google.com/intl/en_us/mapfiles/cb/man_arrow-0.png";
icon.image = imageUrl;
icon.iconSize = new GSize(49,52);
icon.iconAnchor = new GPoint(25,36);
icon.infoWindowAnchor = new GPoint(25,6);
marker = new GMarker(latlang, {"icon":icon, "draggable":true});
map.addOverlay(marker);
After adding a marker in to the map, the map should have listener events to listen when drag, drop events occur. To do this with Google maps, we should call the GEvent.addListener()
method. The code for handling drag and drop events is listed below. The GStreetviewOverlay
object should be defined before calling the addListener()
event.
var streetOverlay = new GStreetviewOverlay();
GEvent.addListener(marker, "dragstart", function()
{
map.addOverlay(streetOverlay);
}
);
GEvent.addListener(marker, "dragend", function(overlay,latlang)
{
map.removeOverlay(streetOverlay);
openWindow();
}
);
Finally, the panorama view should be opened. I wrote a separate method to do this. The openWindow()
method is called when a drop event occurs.
function openWindow()
{
myPano = new GStreetviewPanorama(document.getElementById("stview"));
var latlang = marker.getLatLng();
myPano.setLocationAndPOV(latlang);
}
Done. For complete code listings, please download the demo project.
Points of Interest
The variables marker
and myPono
should be global variables.
<script type="text/javascript">
var marker = null;
var myPano = null;
function initialize() {
if (GBrowserIsCompatible()) {
................
................
History
- 17th May, 2009: Initial post
- 14th October, 2010: Updated demo
This is my first article on CodeProject.