Flex HTTPService with ASP.NET






4.44/5 (9 votes)
A beginners tutorial on HTTPService of Flex and ASP.NET
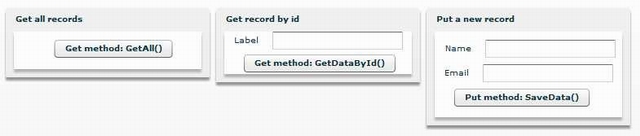
Introduction
This tutorial is to demonstrate the use of HTTPService feature in Flex 2+ with ASP.NET. There are many ways in Adobe Flex to communicate with ASP.NET (or simply, server side languages). HttpService, WebService, WebORB, FluorineFx, etc. In this tutorial, I concentrate only on HTTPService.
<mx:HTTPService />
The attached sample demonstrates how to send and receive data to-and-from ASP.NET. Keep in mind that HTTPService is useful only for handling small amount of data. We will pass requests from flex application as parameters and send back the requested data in XML format.
Role of ASP.NET in HTTPService
HTTPService can handle XML, text, and some more types of data. We will make use of XML for the sample application. ASP.NET application will generate data based on the requests from Flex application. Here is a typical method I used to generate the data in XML format:
private void GetAll()
{
SqlDataAdapter da = new SqlDataAdapter
("SELECT id, name, email FROM test", con);
// Bad code. Just for demo purpose
DataSet ds = new DataSet();
da.Fill(ds);
ds.DataSetName = "parent";
ds.Tables[0].TableName = "child";
// Output data in XML format
Response.Write("<?xml version=\"1.0\" encoding=\"utf-8\"?>");
Response.Write(ds.GetXml());
}
The output of this method looks like this:
<?xml version="1.0" encoding="utf-8"?>
<parent>
<child>
<id>2</id>
<name>Praveen.V.Nair</name>
<email>ninethsense@hotmail.com</email>
</child>
</parent>
Since Flex can send any number of requests for different purposes, I use a switch
checking like:
switch (Request["call"]) {
case "GetDataById":
GetDataById(int.Parse(Request["id"]));
break;
case "GetAll":
GetAll();
break;
case "SaveData":
SaveData(Request["name"], Request["email"]);
break;
case "AnotherMethod":
// your method call
break;
}
It is all about ASP.NET. Now comes the Flex part. You know Flex is more concentrated on UI.
Role of Flex
The core thing is HTTPService. This you need to use like:
<mx:HTTPService id="hsData" url="http://localhost/flextest/Default.aspx"
fault="httpFaultHandler(event)"
result="httpResultHandler(event)"
method="POST"
/>
Please check the documentation for different properties it supports. I used only what we need for the sample application. Well, now we can use its send
method to request data from ASP.NET. httpservice.send()
is how we will do this. If we need to send parameters, we will need to use a flex object
for that. See the example:
private function PopulateDataGridAll(event:MouseEvent):void {
var params:Object = {};
params["call"] = "GetAll";
hsData.send(params);
}
Note that params
is the object I use to store parameters and send to server. After the send()
method, data will be returned to the flex application which we will populate in flex DataGrid
. DataGrid
component code looks like this:
<mx:DataGrid id="dg" dataProvider="{hsData.lastResult.parent.child}">
<mx:columns>
<mx:DataGridColumn headerText="ID" dataField="id"/>
<mx:DataGridColumn headerText="Name" dataField="name"/>
<mx:DataGridColumn headerText="EMail" dataField="email"/>
</mx:columns>
</mx:DataGrid>
Well, the columns details are not necessary. It will show all columns if you do not use it. (Remember the AutoGenerateColumns
feature of ASP.NET).
In the above code, dataProvider
property uses hsData.lastResult.parent.child
where, parent and child are nodes of the XML input. Check the sample XML output of ASP.NET provided on top of this article for more understanding.
If you run the application, you will see the application similar to the screenshot provided. To demonstrate the flex-ASP.NET communication, I added the below mentioned features:
- Get all records (ASP.NET method:
GetAll()
) from server and show inDataGrid
- Get a record (ASP.NET method:
GetDataById()
) from server and show inDataGrid
- Put a record (ASP.NET method:
SaveData()
) to server and show inDataGrid
History
- 11th June, 2009: Initial post