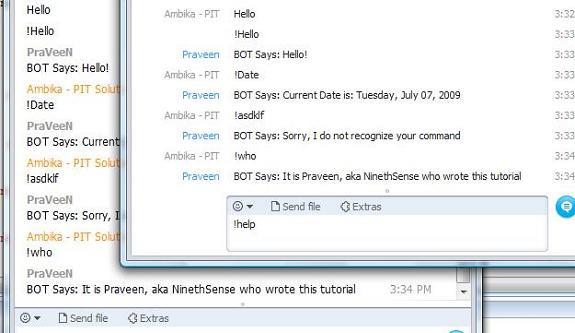
Introduction
The motive of this article is to explain:
- How to make Skype work with .NET.
- How to make an answering bot.
When you type a command !Date (say), it will display the current date. You can extend this application to make your own bots which can handle your own requests.
Some months earlier, I wrote a Skype plug-in for Cropper (an Open Source screen capture tool) called SendToSkype based on the same concept. You can check it here if you wish.
Prerequisites
- Skype. I used version 4.0.0.227.
- I used Visual C# 2008 Express Edition. But you can use any edition.
- COM wrapper - Skype4COM which you an download here. I used release 1.0.31.0 which is the latest available at the time of writing this article.
What is the Skype4COM COM wrapper?
Skype4COM is an interface which represents the Skype API as objects, with properties, commands, and events and notifications. Use Skype4COM in any ActiveX environment, such as Visual Studio or Delphi, and use familiar scripting languages, such as Visual Basic, PHP, or JavaScript. Read more here.
When you develop an app from scratch...
- Download Skype4COM.dll.
- 'Add Reference' to this DLL on your project.
Sample code
Below is the code of the sample application attached. I would like to paste the whole code here since it is only a few lines.
using System;
using System.Windows.Forms;
using SKYPE4COMLib;
namespace SkypeBing
{
public partial class Form1 : Form
{
private Skype skype;
private const string trigger = "!";
private const string nick = "BOT";
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
skype = new Skype();
skype.Attach(7, false);
skype.MessageStatus +=
new _ISkypeEvents_MessageStatusEventHandler(skype_MessageStatus);
}
private void skype_MessageStatus(ChatMessage msg,
TChatMessageStatus status)
{
if (msg.Body.IndexOf(trigger) == 0)
{
string command = msg.Body.Remove(0, trigger.Length).ToLower();
skype.SendMessage(msg.Sender.Handle, nick +
" Says: " + ProcessCommand(command));
}
}
private string ProcessCommand(string str)
{
string result;
switch (str)
{
case "hello":
result = "Hello!";
break;
case "help":
result = "Sorry no help available";
break;
case "date":
result = "Current Date is: " +
DateTime.Now.ToLongDateString();
break;
case "time":
result = "Current Time is: " +
DateTime.Now.ToLongTimeString();
break;
case "who":
result = "It is Praveen, aka NinethSense " +
"who wrote this tutorial";
break;
default:
result = "Sorry, I do not recognize your command";
break;
}
return result;
}
}
}
Remember!
When you try to run this application, make sure Skype is also running. You will be getting a dialog like the one below requesting your permission to communicate your app with Skype.

So do not get nervous. It is nothing unusual.
Source code explanation
Declaration
Once you have referenced Skype4COM.dll, you will be able to use SKYPE4COMLib
which is our core Skype communication component.
using SKYPE4COMLib;
Next, I created a private object skype
of type SKYPE4COMLib.Skype
so that I can use this on all the inner methods (Form1_Load()
, skype_MessageStatus()
, and ProcessCommand()
).
I used two identifiers to store the trigger key and a nick name so that the ordinary chat messages we use in Skype will not have confusions with the triggers.
private const string trigger = "!";
private const string nick = "BOT";
An example of a chat looks like this:
>>Blah
>>!hello
>>BOT Says: Hello!
You can change the trigger and nick according to your taste.
Create a Skype object and attach to Skype
skype = new Skype();
skype.Attach(7, false);
Here, 7 is a Skype protocol.
Listen to incoming messages
You need to create a new event based on _ISkypeEvents_MessageStatusEventHandler
for this.
skype.MessageStatus +=
new _ISkypeEvents_MessageStatusEventHandler(skype_MessageStatus);
All the messages you type in the Skype chat window will be monitored and passed to a method named skype_MessageStatus()
for processing the incoming messages/commands.
Process commands
Here comes the core bot operation. In the method skype_MessageStatus
, our application checks whether the incoming message is a command. Also, to avoid case sensitivity, I convert the message to lower case so that in the processing method (ProcessCommand()
), I need to check only the lowercase commands.
if (msg.Body.IndexOf(trigger) == 0)
{
string command = msg.Body.Remove(0, trigger.Length).ToLower();
skype.SendMessage(msg.Sender.Handle, nick + " Says: " +
ProcessCommand(command));
}
skype.SendMessage()
is the command to send a string to the Skype text window. msg.Sender.Handle
defines to which Skype user this message is to be send.
Here comes the command processing part. This method simply checks the incoming commands and returns a pre-defined text. I am sure not much explanation is required here.
private string ProcessCommand(string str)
{
string result;
switch (str)
{
case "hello":
result = "Hello!";
break;
case "help":
result = "Sorry no help available";
break;
case "date":
result = "Current Date is: " + DateTime.Now.ToLongDateString();
break;
case "time":
result = "Current Time is: " + DateTime.Now.ToLongTimeString();
break;
case "who":
result = "It is Praveen, aka NinethSense who wrote this tutorial";
break;
default:
result = "Sorry, I do not recognize your command";
break;
}
return result;
}
You can see it is very easy to extend the number of commands by simply adding more case
statements.
History
- Initial post: 07, July 2009.