Unraveling the Netflix API - Part III - Accessing Subscriber Account Information
Demonstrates how to access the Netflix subscriber's account information

Contents
- Introduction
- Background
- Accessing a Netflix Subscriber Account
- Example Application
- Conclusion
- History
Introduction
In the previous article in this series, I showed you the basics of constructing a signed request to search the Netflix catalog for titles and parsing those results into data objects in a generic manner. This article explains how to obtain permission to access a subscriber's account information and how to browse through the account.
Background
This article will show you how to:
- obtain authorization to access a Netflix subscriber's account.
- access the information in a subscriber's account.
Accessing a Netflix Subscriber Account
Gaining access to a subscriber's account information is relatively simple, although you might not think so after trying to wade through the Netflix API documentation. Let's walk through it on the following illustration.
Note: I will assume you have read my previous articles and are familiar with the terminology I will be using in this walkthrough.
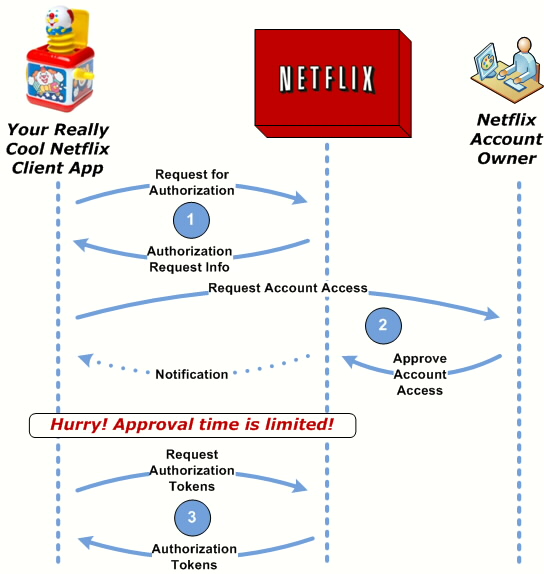
Step 1 - Obtaining a Request for Authorization
The first step is to obtain information from Netflix that enables us to create a request to a subscriber to access their account information. To do this, you make a signed request to http://api.netflix.com/oauth/request_token. The result of this request is a set of information containing:
Item | Description |
|
A temporary security token that is used in a Protected request to obtain the security tokens for accessing the subscriber's account. |
oauth_token_secret |
A temporary security key that is used in a Protected request to obtain the security tokens for accessing the subscriber's account. |
application_name |
The name of your application that is associated with your Consumer Key and Consumer Secret. This is also used in the Protected request to obtain the security tokens for accessing the subscriber's account. |
login_url |
A base URL constructing the Web request that is presented to the subscriber for authorizing access to their account. |
Step 2 - Requesting Account Access
The next step is to construct a Web request that is used to display the authorization request in a browser to the subscriber. The following code snippet shows how this request is constructed using the login_url
and the application_name
items that were returned in the request for authorization information, along with your Consumer Key. Optionally, you can include the URL of a callback handler, which Netflix will call as a notification when the subscriber accepts your request for account access. This example does not use the callback handler, but rather relies on a modal dialog window to synchronize the application processing with the subscriber's acceptance of the authorization request.
// build the access request string
string requestUrl = HttpUtility.UrlDecode((string)authItems["login_url"]) + "&";
// if you wish to specify a callback URL, you can include it here...
// requestUrl += "oauth_callback=my_callback_url=" + callbackUrl + "&";
requestUrl += "oauth_consumer_key=" + consumerKey + "&" +
"application_name=" + authItems["application_name"];
In this example, a dialog is displayed that contains a Web browser control that will display the request to the subscriber. After the subscriber has accepted the request for authorization, she/he is instructed to close this dialog window, which returns control to the account access request processing.
// launch a Web browser using the constructed access request
frmBrowserPopup dlg = new frmBrowserPopup();
dlg.Url = requestUrl;
dlg.ShowDialog();
Step 3 - Obtaining Account Access Authorization Tokens
The final step, now that the subscriber had granted access to his/her account information, is to make one more request to Netflix to obtain a set of permanent tokens that will be used in all Protected requests to obtain information from the subscriber's account.
You may recall that there were two unused parameters in the previous calls to the OAuth.GenerateSignature
method, identified in the example code as the "token
" and "tokenSecret
". These represent an "access token" and a "token secret", respectively and must be used in Protected requests as proof that the caller has been granted access to the requested protected resources. If you refer back to Step 1 in this discussion, you will find that the response contained two elements that I described as "temporary security" items. These are access tokens that will now be used to request the long-living access tokens that allow us to access the subscriber's account information. The reasons that these tokens are temporary are:
- The temporary tokens can only be used once to obtain the subscriber's account access tokens. Once used, they are invalidated.
- The temporary tokens have a limited lifetime. The Netflix documentation does not specify how long these tokens are valid, so it behoves you to use them as quickly as possible.
The following code snippet illustrates the use of the access tokens to generate a signature and normalized request parameters for a Protected request.
// generate request signature
string sig = GenerateSignature(new Uri(requestUrl),
_consumerKey, _consumerSecret,
_oauth_token, _oauth_token_secret,
"GET", GenerateTimeStamp(), GenerateNonce(),
out normalizedUrl, out normalizedRequestParameters);
The response for the Protected request for the subscriber's account access authorization is a set of information containing:
Item | Description |
oauth_token |
The access token that is used in Protected requests to obtain account information for the subscriber. |
oauth_token_secret |
The access key that is used in Protected requests to obtain account information for the subscriber. |
user_id |
The Netflix identity of the subscriber. This can be used to construct the base URL for account information requests. |
These items should be persisted in some manner for future Protected request access to the subscriber's account. There shouldn't be any security issues regarding the protection of this information because these values are only valid with your signed requests - assuming you are keeping your Consumer Key and Consumer Secret well protected.
Account information requests are fairly well described in the Netflix documentation, so I won't go into great detail here. Basically, they consist of the base URL http://api.netflix.com/users/ to which is appended the user_id
followed by any additional qualifiers for specific information in the subscriber's accounts such as rental and instant view queue, rental history, reviews, etc. You will discover all of these areas as you use the Netflix Account Explorer example application that I've included with this article.
Example Application
The example application I've included with this article demonstrates how to obtain account access authorization from a subscriber, and lets you explore the various nooks and crannies of a Netflix account.
Program Design
The program consists of three Windows forms:
- The main form is used for browsing the subscriber's account information.
- The account access request form is used to step through the sequence of obtaining access to a subscriber's account.
- The browser form is popped up to allow the subscriber to grant permission for the application to access their account.
When the application starts, it creates an instance of the NetflixAuth
class, described below, that checks to see if authorization data is available. If so, the application form proceeds to account browsing mode. If the authorization data is not available, then the account access request form is launched. On this form, you step through the process of requesting and obtaining account access from the subscriber, and then you return to the main application form to proceed with browsing the subscriber's account information. Let me start by describing some of the features of the code in this example.
NetflixAuth Class
The NetflixAuth
class is a simple helper that abstracts the storage and retrieval of the subscriber's account access authorization. In practice, this data might be stored in a more reliable manner than just serializing it to a disk file, such as storing it in a database. However, for this example it is sufficient to demonstrate the concept.
NetflixRequest Class
The NetflixRequest
class is the same one used in the Netflix Catalog Explorer example from the previous article in this series with the following additions:
- There is a new method,
ProtectedRequest
, in addition to the previousNonAuthRequest
andSignedRequest
methods. - There are a set of two of methods for each of the three request methods, one of which returns the raw response from the request, and the other that returns the response in an
XmlDocument
object as was done in the previous article. The reason for returning the raw response is that the authentication response contains a set of query string parameters rather than as XML, and therefore this needs to be received and parsed differently. You might also wish to parse the returned XML in a different manner, rather than loading it into anXmlDocument
object, or you might simply wish to view the raw response for testing and debugging. - There is now a new alternate constructor for this class that allows you to specify all four parameters required for creating a Protected request (
ConsumerKey
,ConsumerSecret
,AccessToken
,AccessSecret
).
Main Application Form
The application form consists of the usual TextBox
controls for entering your Consumer Key and Consumer Secret. If this is the first time the application has been run, then the subscriber account access has not yet been granted and so the only action enabled on the form is the Authorize (request authorization) button.
Account Access Request Form
Clicking Authorize (or ReAuthorize if authorization has already been granted), launches the account access request form. If the account access authorization has not been obtained before, the form displays as shown below, indicating that the first step is to generate an authorization request.

When Request Account Access is clicked, a Signed request is sent to Netflix asking for information to be used to present the subscriber with an account access request. The returned information is returned and a URL is formed, as previously described. A BrowserPopup
window is launched (see below) which is directed to the URL created from the access request information given to us by Netflix.

After the subscriber clicks "Yes, Link This Account", they receive the following confirmation. At this point, the subscriber's approval job is done and she/he can close the browser form, which returns her/him to the main application.

Returning to the account access request form, you see that we still do not yet have access to the subscriber's account. This requires one more request, this time a Protected request, using the information that we received from the "Request Account Access" request. Clicking Request Access Info sends this request to Netflix.

The reply from Netflix gives us, at long last, the information we need to access the subscriber's account. This information is stored by the NetflixAuth
object and the account access request form now indicates that we have the authorization we need. Clicking Done closes this form, returning to the main application form, which then verifies through the NetflixAuth
object that we now have access to the subscriber's account information.

Navigating the Subscriber's Account
Once the account access authorization has been received, or if the authorization has been granted previously before the application is started, the form retrieves the basic account information for the subscriber and loads this into a TreeView
control. The TreeView
control loader is straight from the MSDN examples with the following enhancements:
- Any nodes that have attributes are expanded to display those attributes as subordinate elements.
- Attributes that are links to additional resource information are identified as clickable by means of setting the text color to red and adding tooltip text that indicates this.
- A double-click handler is established to allow for navigating the subscriber's account.
When you double-click one of the identified resource links, the application then extracts the URL for that link and sends a Protected Request off for that information. The new location is then pushed onto a history stack and the Back button is enabled so that you can navigate backwards from the new virtual location in the subscriber's account.

As you can see, any resource links ("href
" attributes) are identified as clickable by color and by a tooltip message. Double-clicking a resource link will reload the TreeView
with the information contained at that logical endpoint and also save the previous location in the history list. Clicking Back takes you back to the most previous resource endpoint.
One last feature is that the application form starts out in a relatively minimal size. For better viewing, you can stretch the form window to fit your screen and the TreeView
will expand with it.
Conclusion
This article has described and demonstrated how to obtain permission to access a subscriber's account, and how to navigate the information contained in a subscriber's account. In the next, and final, article in this series I will show you how to manage a subscriber's account using the HTTP POST
and DELETE
methods.
History
- September 4, 2009 - Original submission
- September 8, 2009 - Updated source code