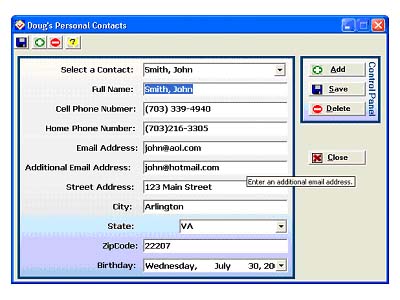
Introduction
This application was created to give me a place to easily access the applications
I often use without having to click on a desktop short cut. I also wanted
a place to keep track of all my personal contacts and their information like
cell phone numbers and email addresses. I didn’t want to bother with using
Outlook to keep track of all this information since it’s a pain in the neck,
plus I don't use it at home. I wanted something simple and portable based
on xml. Customizing this application for your personal needs will take only seconds.
Features
When you run the application, if you click on the Tools button and then select My Contacts, you’ll see the application pictured above which I created to store all my personal contact’s information. This information is stored in a xml file called
My_Contacts.xml. It is really easy to add and delete contacts through this interface which does all the xml work and updates for you.
One cool feature of this application is when you open it up, if you right click the blue background behind the Collapsible Panel, you can add and delete notes you want leave yourself as reminders. This is something I find useful, although you may not.
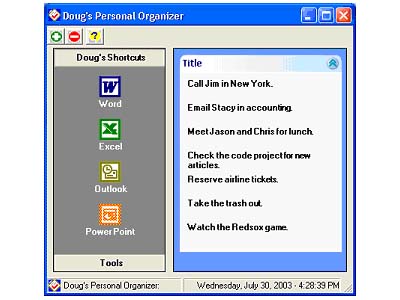
Using the code
This application is not really complicated or to involved, but I find it useful so I thought others might as well.
The code below shows how you can add/update a contact's information in the
xml file. First I pull the data from the windows controls, then I send it
to a helper function which adds the data to the My_Contacts.xml file. The
second function returns a MyContacts object (which is defined in the code)
after it searches the My_Contacts.xml for all your entered personal contacts,
creating your personal contacts list to display.
private void AddContact( XmlDocument XmlDoc, int iContactID,
string sFullName, string sCellPhone, string sHomePhone,
string sEmail, string sEmail2, string sAddress, string sCity,
string sState, string sZip, string sBDay )
{
XmlElement xNodeParent = XmlDoc.DocumentElement;
XmlElement xElemInvestment = XmlDoc.CreateElement( TagContact );
xElemInvestment.SetAttribute( TagContactID, m_iNextID.ToString() );
xNodeParent.AppendChild( xElemInvestment );
sFullName = txt_Name.Text;
sCellPhone = txt_Cell.Text;
sHomePhone = txt_Home.Text;
sEmail = txt_Email.Text;
sEmail2 = txt_Email2.Text;
sAddress = txt_Address.Text;
sCity = txt_City.Text;
sState = cmb_State.Text;
sZip = txt_Zip.Text;
sBDay = dt_DateTimePicker.Value.ToLongDateString();
AddXmlElement( XmlDoc, xElemInvestment, TagFullName, sFullName );
AddXmlElement( XmlDoc, xElemInvestment, TagCellPhone, sCellPhone );
AddXmlElement( XmlDoc, xElemInvestment, TagHomePhone, sHomePhone );
AddXmlElement( XmlDoc, xElemInvestment, TagEmail, sEmail );
AddXmlElement( XmlDoc, xElemInvestment, TagEmail2, sEmail2 );
AddXmlElement( XmlDoc, xElemInvestment, TagStreetAddress, sAddress );
AddXmlElement( XmlDoc, xElemInvestment, TagCity, sCity );
AddXmlElement( XmlDoc, xElemInvestment, TagState, sState );
AddXmlElement( XmlDoc, xElemInvestment, TagZipCode, sZip );
AddXmlElement( XmlDoc, xElemInvestment, TagBDay, sBDay );
m_XmlDocument.Save( m_sXmlFileName );
m_iContactID;
}
private XmlElement FindContactInXmlFile( XmlDocument doc,
string sContactID )
{
XmlElement xNodeFound = null;
XmlElement xRoot = doc.DocumentElement;
XmlNodeList xNodeList = xRoot.GetElementsByTagName( TagContact );
foreach( XmlNode xNodeContact in xNodeList )
{
if( xNodeContact is System.Xml.XmlElement)
{
XmlElement xContact = (XmlElement) xNodeContact;
string sIDFound = xContact.GetAttribute( "ContactID" );
if( sIDFound != null && sIDFound == sContactID )
{
xNodeFound = xContact;
break;
}
}
}
return xNodeFound;
}
Points of Interest
By using an xml flat file in place of a database, the possibilities are endless for storing and retrieving data.
People I'd like to thank
I like to thank Marc Clifton for his Outlook Bar, this is a great control.
I changed a couple of small things but this is a first class submission. I'd
also like to thank Derek Lakin for his Windows XP style Collapsible Panel
Bar, this also a great control!
History
Introduction