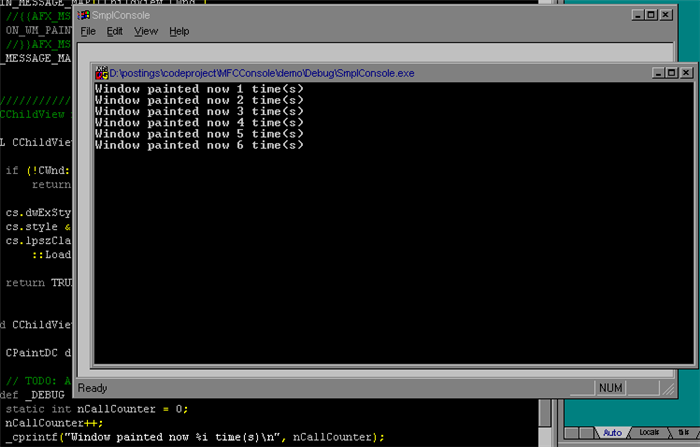
Introduction
It's simply a matter of three function calls to print a message to a console in your MFC application. There are several reasons why you might want to send your debugging output to a console. Mine were, that first, I didn't have an IDE on the PC where I tested the debug-builds of my app and second, the Mainframe was not always visible, which made matters worse.
A last word of warning beforehand: Closing the console window will exit your application! Let's go:
Creating the Console
To create a console window, you need to call AllocConsole()
. This function does not take any parameters and returns a BOOL
, indicating whether the console has been created or not. You should create the console sometime in the beginning of your code. In the sample, I've put the code into the CWinApp::OnInitInstance()
override. Like this:
BOOL CSmplConsoleApp::InitInstance()
{
#ifdef _DEBUG
if (!AllocConsole())
AfxMessageBox("Failed to create the console!", MB_ICONEXCLAMATION);
#endif
return TRUE;
pFrame->LoadFrame(IDR_MAINFRAME,
WS_OVERLAPPEDWINDOW | FWS_ADDTOTITLE, NULL,
NULL);
pFrame->ShowWindow(SW_SHOW);
pFrame->UpdateWindow();
}
It is important that you create your console before you create the main window. If you don't, the console will still be created, but your debugging messages send with _cprintf()
will not arrive in your console. I couldn't figure out why it is like this, only that it is like this. If somebody has an explanation, please let me know.
Writing to the Console
Send your output to the console via _cprintf()
, which is prototyped like this:
int _cprintf( const char *format [, argument] ... );
The format has the same form and function as the format parameter for the printf()
function. Please look up the details in your documentation. And don't forget to #include <conio.h>
wherever you use _cprintf()
.
The CChildView::OnPaint()
override in the demo application looks like this:
void CChildView::OnPaint()
{
CPaintDC dc(this);
#ifdef _DEBUG
static int nCallCounter = 0;
nCallCounter++;
_cprintf("Window painted now %i time(s)\n", nCallCounter);
#endif
}
Getting Rid of the Console
Somewhere near the end of your program, you should call FreeConsole()
to free the console <g>
. Again, this function does not take any parameters and returns a BOOL
indicating success or failure. Here is what the CWinApp::ExitInstance()
override in the demo project looks like:
int CSmplConsoleApp::ExitInstance()
{
#ifdef _DEBUG
if (!FreeConsole())
AfxMessageBox("Could not free the console!");
#endif
return CWinApp::ExitInstance();
}
Hope that helps!
License
This article has no explicit license attached to it, but may contain usage terms in the article text or the download files themselves. If in doubt, please contact the author via the discussion board below.
A list of licenses authors might use can be found here.
I'm into photography a lot. And yes, I do code as well. Mostly C# these days.