A must have list of ASP.NET MVC Interview Questions and Answers with concepts and necessary code examples. If you understand following key concepts, you will definitely feel more comfortable during an interview. But along with that you need to prepare your practical skills on ASP.NET MVC technology. You can get more related stuff at the end of this article but let's first move forward and get answers to following questions.
1. Explain MVC (Model-View-Controller) in general?
MVC (Model-View-Controller) is an architectural software pattern that basically decouples various components of a web application. By using MVC pattern, we can develop applications that are more flexible to changes without affecting the other components of our application.
- "Model" is basically domain data.
- "View" is user interface to render domain data.
- "Controller" translates user actions into appropriate operations performed on model.
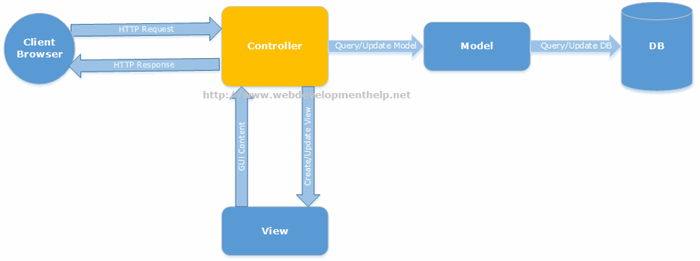
2. What is ASP.NET MVC?
ASP.NET MVC is a web development framework from Microsoft that is based on MVC (Model-View-Controller) architectural design pattern. Microsoft has streamlined the development of MVC based applications using ASP.NET MVC framework.

3. Difference between ASP.NET MVC and ASP.NET WebForms?
ASP.NET Web Forms uses Page controller pattern approach for rendering layout, whereas ASP.NET MVC uses Front controller approach. In case of Page controller approach, every page has its own controller, i.e., code-behind file that processes the request. On the other hand, in ASP.NET MVC, a common controller for all pages processes the requests.
Follow the link for the difference between the ASP.NET MVC and ASP.NET WebForms.
4. What are the Core features of ASP.NET MVC?
Core features of ASP.NET MVC framework are:
- Clear separation of application concerns (Presentation and Business Logic). It reduces complexity that makes it ideal for large scale applications where multiple teams are working.
- It’s an extensible as well as pluggable framework. We can plug components and further customize them easily.
- It provides extensive support for URL Routing that helps to make friendly URLs (means friendly for human as well as Search Engines).
- It supports for Test Driven Development (TDD) approach. In ASP.NET WebForms, testing support is dependent on Web Server but ASP.NET MVC makes it independent of Web Server, database or any other classes.
- Support for existing ASP.NET features like membership and roles, authentication and authorization, provider model and caching etc.
Follow for detailed understanding of the above mentioned core features.
5. Can you please explain the request flow in ASP.NET MVC framework?
Request flow for ASP.NET MVC framework is as follows:
Request hits the controller coming from client. Controller plays its role and decides which model to use in order to serve the request further passing that model to view which then transforms the model and generates an appropriate response that is rendered to the client.

You can follow the link, in order to understand the Complete Application Life Cycle in ASP.NET MVC.
6. What is Routing in ASP.NET MVC?
In case of a typical ASP.NET application, incoming requests are mapped to physical files such as .aspx file. On the other hand, ASP.NET MVC framework uses friendly URLs that more easily describe user’s action but not mapped to physical files. Let’s see below URLs for both ASP.NET and ASP.NET MVC.
<br />
http:
http:
<br />
http:
http:
ASP.NET MVC framework uses a routing engine, that maps URLs to controller classes. We can define routing rules for the engine, so that it can map incoming request URLs to appropriate controller.
Practically, when a user types a URL in a browser window for an ASP.NET MVC application and presses “go” button, routing engine uses routing rules that are defined in Global.asax file in order to parse the URL and find out the path of corresponding controller.
You can find complete details of ASP.NET MVC Routing here.
7. What is the difference between ViewData, ViewBag and TempData?
In order to pass data from controller to view and in next subsequent request, ASP.NET MVC framework provides different options i.e., ViewData
, ViewBag
and TempData
.
Both ViewBag
and ViewData
are used to communicate between controller and corresponding view. But this communication is only for server call, it becomes null
if redirect occurs. So, in short, it's a mechanism to maintain state between controller and corresponding view.
ViewData
is a dictionary
object while ViewBag
is a dynamic property (a new C# 4.0 feature). ViewData
being a dictionary
object is accessible using string
s as keys and also requires typecasting for complex types. On the other hand, ViewBag
doesn't have typecasting and null
checks.
TempData
is also a dictionary
object that stays for the time of an HTTP Request. So, Tempdata
can be used to maintain data between redirects, i.e., from one controller to the other controller.

You can easily find detailed examples for implementation of ViewBag, ViewData and TempData here.
8. What are Action Methods in ASP.NET MVC?
I already explained about request flow in ASP.NET MVC framework that request coming from client hits controller first. Actually MVC application determines the corresponding controller by using routing rules defined in Global.asax. And controllers have specific methods for each user actions. Each request coming to controller is for a specific Action
Method. The following code example, “ShowBooks
” is an example of an Action
method.
public ViewResult ShowBooks(int id)
{
var computerBook = db.Books.Where(p => P.BookID == id).First();
return View(computerBook);
}
Action methods perform certain operation using Model and return result back to View. As in above example, ShowBook is an action method that takes an Id as input, fetch specific book data and returns back to View as ViewResult. In ASP.NET MVC, we have many built-in ActionResults type:
- ViewResult
- PartialViewResult
- RedirectResult
- RedirectToRouteResult
- ContentResult
- JsonResult
- EmptyResult
- and many more...
For a complete list of available ActionResults types with Helper methods, please click here.
Important Note: All public methods of a Controller in ASP.NET MVC framework are considered to be Action Methods by default. If we want our controller to have a Non Action Method, we need to explicitly mark it with NonAction attribute as follows:
[NonAction]
public void MyNonActionMethod() { ….. }
9. Explain the role of Model in ASP.NET MVC?
One of the core features of ASP.NET MVC is that it separates the input and UI logic from business logic. Role of Model in ASP.NET MVC is to contain all application logic including validation, business and data access logic except view, i.e., input and controller, i.e., UI logic.
Model is normally responsible for accessing data from some persistent medium like database and manipulate it, so you can expect that interviewer can ask questions on database access topics here along with ASP.NET MVC Interview Questions.
10. What are Action Filters in ASP.NET MVC?
If we need to apply some specific logic before or after action methods, we use action filters. We can apply these action filters to a controller or a specific controller action. Action filters are basically custom classes that provide a means for adding pre-action or post-action behavior to controller actions.

For example:
Authorize
filter can be used to restrict access to a specific user or a role. OutputCache
filter can cache the output of a controller action for a specific duration.
Related Web Development Tutorials
CodeProject