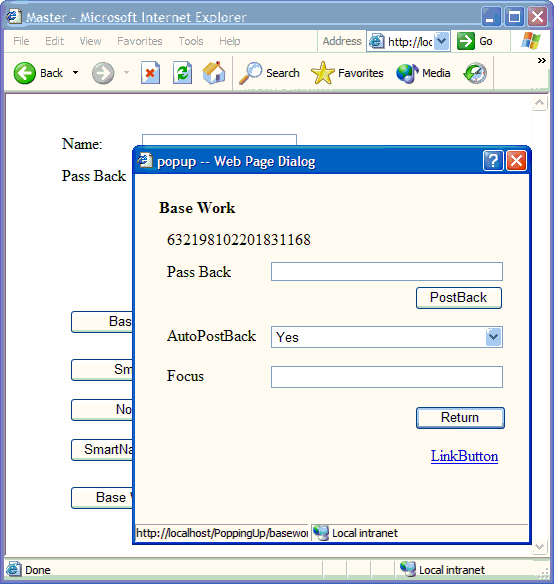
Introduction
Ever tried to create a pop-up in ASP.NET? Well, in this article, we will see different ways to create them. We'll also see a few issues surrounding the different ways.
Background
As developers, we often have to think about the user interface. Good developers create a library of user interface templates that they can reuse from application to application. A template or tool often used in user interfaces (UI) is the pop-up or modal form. A modal form places the user into a state where they have to perform an action on the form to continue any further in the application. An example of this UI tool in a screen workflow is the common File Save As window. When a user calls this common Window menu item, a screen shows asking for the name to save the file as. The users on the File Save As screen typically can’t do anything else with the application until they complete this screen. Many consider this UI workflow to be clean and user friendly. So, how does one go about creating modal forms in ASP.NET?
Issue
ASP.NET 1.0 and 1.1 didn’t come with a mechanism to support the concept of modal forms. In fact, many Internet browsers lack built-in support for the concept of modal forms.
What can we do?
Fortunately, Microsoft’s Internet Explorer (IE) has a special function to open a modal form. While cruising through the web, I found various postings supplying pop-up solutions for later versions of IE. And to them I say, “Thank you!”. Pulling their solutions together, I was able to build a simple sample showing their techniques. Hopefully, you can include some of the findings here in your Internet Explorer applications.
Call Popup:
To start things off, let’s look at how to call a pop-up form with IE. Later, we’ll look into how to make simple modal forms. In the sample code, we have a simple web form, ‘master’. To master, I added a few ASP.NET buttons to open different flavors of modal forms. The buttons have names that correspond with the pop-up form they will open, like btnBaseWork
. Normally, when a user presses a button, a post-back event occurs, sending the event to the server for processing. In the solutions here, the click event happens on the client, a pop-up form shows, and then the click event is passed to the server. Our real goal is to prevent further activity on the master form until the user completes work on the pop-up form. To make this happen, we’ll use ASP.NET code to add modal-opening JavaScript to the ‘onclick
’ event for the button, in the Page_Load
event:
btnBaseWork.Attributes.Add("onclick",
"window.showModalDialog('BaseWork.aspx','BaseWork',
'dialogWidth:400px;dialogHeight:300px;');");
If you want to know more about showModalDialog
and its properties, check out Microsoft’s MSDN site. It's not hard to find; look for an article by DHTML Dude called ‘The Importance of Good Dialog’, and for details, look for ‘showModalDialog Method’.
How does the modal form know what to show, receive information? In our case, let's say we want it to show the address for a given customer. There are actually many different ways to do this, the easiest is to set a value in Session or to place the value on the querystring
. If you plan on using a value in session, the session value needs to be set before the button is clicked; a tricky task. The following is an example for passing the value via a querystring
.
System.int32 myAddressID = 100;
String javascript = "window.showModalDialog('addresspop.aspx?AddressID=" +
myAddressID.ToString() + "','Address','dialogWidth:400px;dialogHeight:400px;');"
btnOpenPopUp.Attributes.Add("onclick",javascript);
That’s fairly simple.
Make Modal Forms
Well, we’ve taken care of calling the pop-up/modal window, what about making the modal form?
Solution 1, Base:
The modal form in IE is a little unusual. One way to overcome this weirdness involves placing the following statement in the header of the intended modal form:
<base target="_self">
What the heck does this do? Essentially, it tells the form that it is the target of itself when it comes to various functions, crazy. Beyond that, I have no idea and am not too worried about it, just like the MSDN documentation at time of investigation wasn’t too worried about documenting it. For us, it means the pop-up form can postback to itself. That’s all that needs to be done for this solution. The sample code provides several samples of this solution.
Solution 2, Smart Navigation:
The next technique uses functionality from ASP.NET. The solution here merely involves setting the smart-navigation on for the pop-up form. As a side comment, I wonder why Microsoft didn’t tell us exactly what smart navigation does to the generated client code and when. Observation finds that when smart navigation normally executes, the result is an IFRAME
in the form that encompasses the form's controls. Hence when the form postbacks, it essentially postbacks within the IFRAME
. Again, adding smart navigation to the pop-up form will result in the form's controls placed in an IFRAME
, mostly. You could have created the form with your own IFRAME
and then another form to go into the IFRAME
, but that’s extra work. To make smart navigation work with a pop-up, we need to add one more little trick. Remember, pop-up/modal forms are a little different. IE with default settings likes to cache forms, especially pop-up forms. Heck, modal forms are cached, all over the place. The following code added to the Page_Load
event helps to prevent this caching:
Response.Cache.SetNoServerCaching();
Response.Cache.SetCacheability(System.Web.HttpCacheability.NoCache);
Response.Cache.SetNoStore();
Response.Cache.SetExpires(new DateTime(1900,01,01,00,00,00,00));
There are variations to this, but I tried to stick with ASP.NET code. In the sample code, we’ll call different modal forms to demonstrate various characteristics of IE modal forms.
So Far So Good:
At this point, we’re ready to walk through the provided sample discussing findings that are good and a few that are a little unfortunate.
In observing the running code, I really wanted to monitor when certain events happened, without drastically affecting the client output. So on each form, I included a Label
that I use to show the current tick. In various locations, mostly buttons, I send the current tick to the tick-label and to the output window. On some locations, I change the text in a TextBox
to also show tick information. Having the tick information in these locations will allow us to see if the click event occurred on the server, but resulted in no changes to the client HTML. Now, let’s review the forms and what they tried to show.
- BaseWork:
This form has nothing special and uses Solution 1. The buttons work and even the postback for a DropDownList
works. The link button works. Pretty much everything works!
- BaseScroll:
Very similar to the BaseWork form using Solution 1, but sized different. Upon experimentation, it can be quickly found that the scroll position and element focus are not maintained. How often should a pop-up form show a scrollable data entry screen?
- SmartNav:
A simple screen with smart navigation on and everything appears to work fine. However, if you click on the buttons, you’ll notice, not every click event actually results in a change on the client. The output window will show that the event did occur on the server. Very strange. Here’s another strange finding, the ‘Return’ button with a RegisterStartUpScript
to close the form always works. The link button doesn’t work. Again, strange. Did I mention this is strange?
- SmartNavNoWork:
An even simpler screen than SmartNav with smart navigation turned on. However, nothing appears to work. It doesn’t appear any of the postback events work. Well they do work, but the client doesn’t change. You can see this by watching the output window where I send tick information and the tick label on the form. Running the form normally, I observed that smart navigation changed very little of the generated HTML. There must be some strange logic in the smart navigation code, but that’s not the concern here. The concern is how to get smart navigation to work consistently.
- SmartNavWorkScroll:
This screen has been sized to see if smart navigation still supports its scrolling feature. After a little testing, you will find it does maintain scroll position and element focus. This form looks simple like SmartNavNoWork. What did I change to make the smart navigation even add smart navigation code to the generated HTML? Well, I added a DropDownList
with a width of 0px and AutoPostBack
set to true. Did I mention things are very strange?
- SmartNavWorkReg:
Another simple form using smart navigation. However, it too does not have ‘enough’ controls for smart navigation to generate smart navigation code on the client. What’s fun is that I added a RegisterStartUpScript
that sends an alert whenever the Page_Load
event fires. For our confusion, the RegisterStartUpScript
code fires on the client through every Page_Load
event, even though the client HTML doesn’t appear to change.
- BaseWithGrid:
Now, we’re getting to the good stuff, a form using Solution 1 with a DataGrid
. Paging works. Editing works. Updating works. Cancel works. From this quick example, one could almost conclude that a DataGrid
works using base. I say almost, cause I didn’t test everything.
- SmartNavWithGrid:
This form uses Solution 2 with a DataGrid
. This one has to work. Nope. There are a few gotchas, or should I say issues. On the good side, this one worked without a 0 width-AutoPostBack
DropDownList
. However, the page buttons generated from the DataGrid
won’t work. Meaning you may have to implement your own paging and may be sorting. Yuk.
Smart Navigation in General:
Here are a few other quick findings about the smart navigation solution. In order for it to work, the user pretty much has to run JavaScript on the client. No biggy there. Now for the nasty one, make sure the IE browser is set to check for a new page on every visit to a page. If the user changes this setting, smart navigation itself may have problems. In VS 2003, watch out for a little bug. If you change the smartNavigation
, enableViewState
, and a few other items through the Properties window, the designer may change the InitializeComponent
event to contain nothing. A way to get around this bug is to make your changes in the HTML designer.
That was quick, and well it should be. You really need to see the sample code. This article and supporting code shows how to make a few different types of modal forms, shows issues with them, and how to overcome a few issues with them. To improve upon this, one might include adding a control to simulate smart navigation's return to a control/scroll position. There’s even a few steps to turn all this into a framework. (See this page. Be prepared to wait; it takes a while to show.) The last great improvement would be to make pop-ups work with other browsers (see this page for a potential solution). Unfortunately, that’s all the time I have for now…good luck.
History
None yet.
A biography in this little spot...sure.
I've worked at GTE HawaiianTel. I've worked at Nuclear Plants. I've worked at Abbott Labs. I've consulted to Ameritech Cellular. I've consulted to Zurich North America. I've consulted to International Truck and Engine. Right now, I've consulted to Wachovia Securities to help with various projects. I've been to SHCDirect and now at Cision.
During this time, I've used all kinds of tools of the trade. Keeping it to the more familier tools, I've used VB3 to VB.NET, ASP to ASP/JAVASCRIPT/XML to ASP.NET. Currently, I'm developing with C# and ASP.NET. I built reports in Access, Excel, Crystal Reports, and Business Objects (including the Universes and ETLS). Been a DBA on SQL Server 4.2 to 2000 and a DBA for Oracle. I've built OLTP databases and DataMarts. Heck, I've even done Documentum. I've been lucky to have created software for the single user to thousands of concurrent users.
I consider myself fortunate to have met many different people and worked in many environments. It's through these experiences I've learned the most.