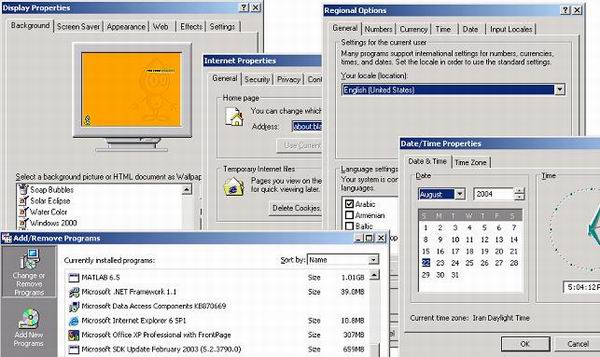
Introduction
RunDll32.exe is one of the most useful tools that Microsoft developed first for internal use, but because of wide application of this simple utility, delivered to developers. With this simple utility, one can launch system dialogs like applets of Control Panel or dialogs that are hidden in some DLLs like Format dialog or Device Manager Property Sheet dialog.
For testing the Rundll32.exe, it's enough to click the Start button then choose Run... from Start menu, and type this syntax:
Rundll32.exe <name of dll> <entry point function> <arguments>
For example, for launching the Format dialog, it's enough to run this statement:
Rundll32.exe Shell32.dll SHFormatDrive
Shell32.dll is a DLL that hides the Format Dialog and SHFormatDrive
is the entry point function for launching the Format dialog.
RunDll32 Internal
The entry point function that Rundll32.exe tries to load has the syntax shown below (for Unicode):
void <entry point function> (HWND hwndStub, HINSTANCE hAppInstance,
LPWSTR lpCmdLine, int nCmdShow);
and for ANSI:
void <entry point function> (HWND hwndStub, HINSTANCE hAppInstance,
LPSTR lpCmdLine, int nCmdShow);
hwndStub
is the handle of a window that calls the function. hAppInstance
is the instance handle of the application, lpCmdLine
is the command line argument of the entry point, and nCmdShow
specifies how to show the dialog.
The RunDll32.dll simply loads the library (*.dll), then try loading the desired function by using GetProcAddress()
. We can also do this by writing this code:
#ifdef _UNICODE
typedef void (_stdcall *PFUNCTION_ENTRYPOINT)(HWND hwndStub,
HINSTANCE hAppInstance,
LPWSTR lpCmdLine,
int nCmdShow);
#else
typedef void (_stdcall *PFUNCTION_ENTRYPOINT)(HWND hwndStub,
HINSTANCE hAppInstance,
LPSTR lpCmdLine,
int nCmdShow);
#endif
PFUNCTION_ENTRYPOINT pEntryPoint=NULL;
HINSTANCE hInst=AfxGetInstanceHandle();
HMODULE hModule = LoadLibrary(DllName);
if (hModule)
{
pEntryPoint = (PFUNCTION_ENTRYPOINT) GetProcAddress(hModule, FunctionName);
}
if (pEntryPoint)
{
pEntryPoint(hParent, hInst, CommandLine, SW_SHOW);
}
CSystemDialog
CSystemDialog
is a very simple class to do this stuff automatically. It has only one member function:
void DoModal(int iDialogID, HWND hParent);
iDialogID
is the identifier of the dialog and hParent
is the handle of the main window of your program. Here is an example:
CSystemDialog dlg;
dlg.DoModal(SD_GAME_CONTROLLERS, m_hWnd);
The definition of the class is as below:
#ifndef _SYSTEM_DIALOGS_H_
#define _SYSTEM_DIALOGS_H_
#if _MSC_VER > 1000
#pragma once
#endif // _MSC_VER > 1000
#define SD_FORMAT 1
#define SD_INTERNET_OPTIONS 2
#define SD_ADD_REMOVE_PROGRAMS 3
#define SD_DATE_TIME 4
#define SD_DISPLAY 5
#define SD_MODEM 6
#define SD_MULTIMEDIA 7
#define SD_MOUSE 8
#define SD_NETWORK 9
#define SD_PASSWORD 10
#define SD_SYSTEM 11
#define SD_REGIONAL_SETTINGS 12
#define SD_SOUNDS 13
#define SD_GAME_CONTROLLERS 14
#define SD_KEYBOARD 15
#define SD_DEVICE_MANAGER 16
typedef struct tagSystemDialog
{
int iSystemDialogID;
TCHAR cDllName[100];
char cFuncName[256];
TCHAR cCommand[100];
} SystemDialog;
class CSystemDialog
{
public:
void DoModal(int iDialogID, HWND hParent);
CSystemDialog();
virtual ~CSystemDialog();
};
#endif // _SYSTEM_DIALOGS_H_
Enjoy!