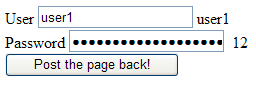
Disclaimer
The author does not accept responsibility for any effects, adverse or otherwise, that this code may have on you, your computer, your sanity, your dog, and anything else that you can think of. Use it at your own risk. (Deja Vu? I also love the Allegro disclaimer.)
Quick and dirty tour
So, you don't like to read all articles you see on the web, or you don't have time for it? Then, just download the source files, and open the solution as a web project on your Visual Studio 2005 IDE, and click Play.
Background
You only need to have a basic knowledge of ASP.NET and C# to use and benefit from the article.
Introduction
Stop where you are! Don't think about reading this article thinking about a magic solution for the world's problems. It is a very simple way of caring about more things than just the problem at hand. And also, minding about collateral effects of apparent feasible solutions.
Understanding the problem at hand
You have a page with a textbox on password mode that you need to persist the state during postbacks. .NET keeps erasing its content when you postback the page, and you want it no more! You just have to keep its data. Simple, right? Not really. I'll explain why it is so simple to explain and it is so tricky to solve.
The illusion of solving a problem
During my early years as a .NET developer, a few years ago, I stuck on this very problem, and by using my favorite friend consulting (Google), I ended up with a widely used solution (for newbies, of course).
It was something like this: you write the password field value back to itself on every page_load
event. Wow! Let's see how it would look like:
protected void Page_Load(object sender, EventArgs e)
{
txtPassword.Attributes.Add("value", txtPassword.Text);
}
That will solve the problem of losing the password at a very high cost. Run the DoesNotSolveTheProblem.aspx page on the source solution. Type a user and password, click on the button, and see that the password is indeed persisted. Now, click on View Source on your browser. Look for the txtPassword
HTML tag:
<input name="txtPassword" type="password"
maxlength="128" id="txtPassword" value="123" />
See anything that shouldn't be there? You are exposing the password, in plain text!
Solving the problem
There is a way for solving this problem very easily, it is a little tricky though. First, you need to keep the password on the server side, so a way to do so is using a server-side variable...not! It would be erased at every page load, so we go for ViewState.
It would look like this:
protected string TypedPassword
{
get
{
if (ViewState["TypedPassword"] != null)
{
return Convert.ToString(ViewState["TypedPassword"]);
}
return null;
}
set
{
ViewState["TypedPassword"] = value;
}
}
We also need to keep a fake data on the field (like a bunch of '*'s) to inform the user that there is data present in that field. We also need a way of detecting that the user typed a password, and that it is not the fake password we kept in the password field. Remember that '*' is a valid character for passwords!
Here comes the trick.
Passwords on modern Windows systems (post Windows 98 versions) can have up to 128 characters, so we limit the HTML password field to 128 characters by using its MaxLength
property. We set the fake password mask to 129 '*' characters.
On every page load, we test if the password field is not blank and if it is different from the fake mask to detect if any change occurred.
if (txtPassword.Text.Trim().Length > 0 && txtPassword.Text != PasswordMask)
{
TypedPassword = txtPassword.Text;
txtPassword.Attributes.Add("value", PasswordMask);
}
Since the user can not type more than 128 characters, he can enter a password full of '*' characters and have a valid password. And, how can we set 129 characters on a MaxLength
=128 field? Well, this validation occurs on the client side, so we are able to do whatever we want on server-side.
Now, the last part of the cryptic show. When the user clicks on the password field, we have to erase its content (it is 129 '*' chars, not the password itself, remember?) and let the user type the new password. To do so, we add a simple JavaScript code.
This way, we do not compromise the security since the password is not in plain text on the page source. However, it is encoded (base64) in the ViewState hidden field, which can be used without encoding. My little piece of advice: don't.
See KeepPassword.aspx for the complete source.
Solving the right problem
This monster of keeping the password was born because we created it by using a bad design for our systems. If you put a little more effort on the design of an application, you could avoid these kind of tricky problems and come up with clean and robust approaches.
BetterDesign.aspx page has a complete example of a different way of looking at the same problem.
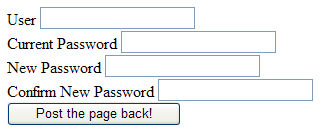
As you can see, there is no need to keep the password. When the user wants to change it, he just types it and that's all! Plus, asking for the current password and for the new password confirmation would improve security and avoid costly typos when setting new passwords.
You will see that this last solution does not use the typed password for storage purposes, but its hashcode (unique number generated from a string) instead. It is a common way of dealing with sensible data (like a password), and it is very simple too.
Catches
Besides the ViewState encoding situation I've mentioned before, there is another piece of information I must give you. Two strings can generate the same hashcode. Yes, I lied. But, it is such a remote possibility that we usually forget about it for most applications. Caring about it and storing plain text passwords instead would be a blatant mistake.
A few comments on hashcode algorithms are also necessary. The GetHashCode()
method used in this solution is good for learning purposes, but should not be used in real systems. Instead, you should use the .NET provided (keyed or nonkeyed) hash algorithms like RIPEMD160Managed
, SHA1CryptoServiceProvider
, or SHA1512Managed
(example below, on jimmy_b comments). You can find lots of articles about the subject on CodeProject, like this.
Also, I'm not using validators or any other .NET feature here for the sake of simplicity. But, it doesn't mean you shouldn't be using it. :)
Conclusion
That's all I have to offer you for now. Please feel free to ask for new functionality, report bugs, or to tell me how boring is this stuff.
References
Some great resources on the web:
History
- 05-31-2007:
- Added a few comments on hash algorithms, thanks to jimmy_b.
- 05-25-2007:
- Changed "encryption" to "encoding", which is the correct term for ViewState. Thanks to William who pointed it out!
- 05-24-2007: