Introduction
This project will show a different way how to:
- enter only integer value in a
TextBox
.
- enter only the characters you want and avoid certain invalid characters in a
TextBox
.
- highlight the E-Mail address in a
TextBox
in color.
- and check whether the E-Mail address is a valid or an invalid one.
through the class System.Text.RegularExpressions.Regex()
. It will also show how you can use ErrorProvider
and ToolTip
when you enter false values in a TextBox
.
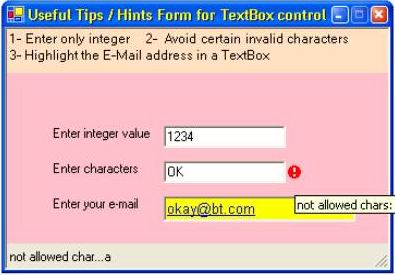
Code and How it works
I use 3 TextBox
es. textboxInteger
is for integer values, textboxEmail
for E-Mail addresses, and textboxChars
for char
values. Let's start with TextBox
'textboxInteger
'.
As mentioned before, you can enter here only integer values. Other characters will not be accepted.
Here is the method for input integer:
private void textboxInteger_KeyPress(object sender,
System.Windows.Forms.KeyPressEventArgs e)
{
if (!Char.IsDigit(e.KeyChar) && e.KeyChar !=(char)8)
{
this.statusBar1.Text="incorrect key...";
e.Handled=true;
} else
this.statusBar1.Text="OK..";
}
As you can see, we check whether the char
you typed in the TextBox
is a decimal digit or backspace-key for deleting the char
s. If it's not, we won't pass it on to the control so that you can't see it in the TextBox(e.Handled=true)
. Otherwise, we display the status bar text saying "OK.." which means you entered correct decimal digits.
TextBox 'textboxChars'
We want here to avoid entering certain invalid characters (i.e., 'a','b','c','#','*','1'). To solve this, I implemented 3 methods in Form1.cs:
mTextboxCharsKeyPressWithForeach
mTextboxCharsKeyPressWithIF
mTextboxCharsKeyPressWithSwitch
that I prefer:
private void mTextboxCharsKeyPressWithSwitch(object sender,
System.Windows.Forms.KeyPressEventArgs e)
{
this.statusBar1.Text="OK...";
switch(e.KeyChar)
{
case 'a':
case 'b':
case 'c':
case '#':
case '*':
case '1':
e.Handled=true;
this.errorProvider1.SetError(this.textboxChars,
"not allowed chars: 'a','b','c','#','*','1'");
this.statusBar1.Text="not allowed char..."+e.KeyChar;
break;
default:
this.errorProvider1.SetError(this.textboxChars, "");
break;
}
}
If the chars you typed are 'a', 'b', 'c', '#', '*', '1', then we use an ErrorProvider
and ToolTip
control on the TextBox
. An error icon appears next to the TextBox
. If you hold the mouse pointer over the error icon, a tool tip appears displaying the error message.
TextBox 'textboxEmail'
As soon as you typed a correct e-mail address, it will change the BackColor
of TextBox
to yellow and ForeColor
to blue underlined. And it will also show in a MessageBox
the user name and host name. If you miss entering after @, it will show it's not a valid e-mail address. The method for the TextBox
'textboxEmail
':
private void textboxEmail_TextChanged(object sender, System.EventArgs e)
{
if (this.textboxEmail.Text.IndexOf("@") != -1
&& this.textboxEmail.Text.IndexOf(".") != -1)
{
this.textboxEmail.BackColor=Color.Yellow;
this.textboxEmail.ForeColor=Color.Blue;
this.textboxEmail.Font=new Font("Arial",
10, FontStyle.Underline);
}
else
{
this.textboxEmail.BackColor=Color.White;
this.textboxEmail.ForeColor=Color.Black;
this.textboxEmail.Font=new Font("Arial",
10, FontStyle.Regular);
}
}
The indexOf
method returns an integer value indicating the beginning of the substring within the String
object. If the substring is not found, a -1 is returned. If a valid e-mail address was entered, BackColor
and ForeColor
will be changed and the FontStyle
will be "FontStyle.Underline
". Otherwise, it will change to old style.
To check whether it's a valid or invalid e-mail address, I use with the help of regular expression, a pattern match to validate the e-mail address. Here is the method.
private void textboxEmail_Validating(object sender,
System.ComponentModel.CancelEventArgs e)
{
Regex emailregex = new Regex("(?[^@]+)@(?.+)");
Match mmatch = emailregex.Match(this.textboxEmail.Text);
if ( mmatch.Success )
{
MessageBox.Show("UserName: " +
mmatch.Groups["user"].Value+"\n"+"HostName: " +
mmatch.Groups["host"].Value,"E-Mail Information");
this.statusBar1.Text="";
}
else
{
MessageBox.Show("invalid e-mail address...","E-Mail Information");
e.Cancel=true;
}
}
The Match
object returns regardless of whether any matches were found in the source string. With the Success
property, we can decide whether to continue processing the Match
object or to display an error message. If successful, we display the "user" and "host" named groups within the Groups
collection of the Match
object. Otherwise, you try it again. Make sure that the first half of the string does not have an @ symbol.
In conclusion
I've designed this program to show the basics to the beginners. Who knows maybe the experts could make good use of some hints/tips here.
Enjoy and good coding!
H�seyin Altindag