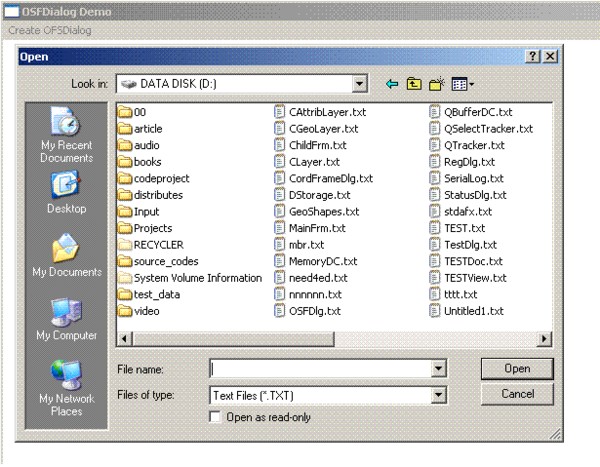
Introduction
This article introduces a simple class "COSFDialog
" that provides a File Open/Save dialog functionality using Win32SDK. This is an alternative to similar MFC classes. But the main purpose of this code is to avoid MFC dependencies and to provide a simple and clear (I hope) mechanism to get file paths from the user.
Using the code
Everything is simple.To put it to work you need to:
- Add files "OSFDlg.h" and "OSFDlg.cpp" to your project.
- Include OSFDlg header file in your module:
#include "OSFDlg.h"
- Declare and initialize three variables:
TCHAR szFilter[] = TEXT ("Text Files (*.TXT)\0*.txt\0") \
TEXT ("All Files (*.*)\0*.*\0\0") ;
TCHAR szDefExtention[] = TEXT("txt\0");
static COSFDialog osfDlg;
Of course you can specify any subset of file extensions as per your requirements.
And then, in your code you should simply call COSFDialog
's member functions FileOpenDlg
and FileSaveDlg
to present the appropriate dialog box to the user. FileOpenDlg
function provides the possibility to create FileOpen
dialog that allows multiple or single selection. I will explain about it shortly. To retrieve paths to selected file, you need to call appropriate member functions: GetFileName
(for dialog version with single selection) or GetNextFileName
(for multiple selection version). The following code examples demonstrate all these actions:
if(osfDlg.FileOpenDlg(szFilter, szDefExtention, NULL, TRUE))
{
PCTSTR strFile;
while((strFile = osfDlg.GetNextFileName()) != TCHAR('\0'))
{
ifstream ifs;
ifs.open(strFile);
ifs.close();
}
}
if(osfDlg.FileOpenDlg(szFilter, szDefExtention, NULL, FALSE))
{
ifstream ifs;
ifs.open(osfDlg.GetFileName());
ifs.close();
}
if(osfDlg.FileSaveDlg(szFilter, szDefExtention, NULL))
{
HANDLE hFile = CreateFile(osfDlg.GetFileName(),
GENERIC_WRITE,
0,
NULL,
CREATE_ALWAYS,
FILE_ATTRIBUTE_NORMAL |
FILE_FLAG_OVERLAPPED,
NULL);
CloseHandle(hFile);
}
I have to explain a little about arguments that we pass to member functions FileOpenDlg
and FileSaveDlg
. As you can see from the code example, FileOpenDlg
function has four arguments. Here is how this function is declared in "OSFDialog.h"
BOOL FileOpenDlg(PTSTR szFilter,
PTSTR szDefExt,
PTSTR szDlgTitle,
BOOL fMultSel);
You can specify the dialog title as a third parameter:
if(osfDlg.FileOpenDlg(szFilter, szDefExtention,
TEXT("OPEN MY FAVORITE FILES:)"), TRUE))
{
}
Or simply pass NULL
for this parameter.
FileSaveDlg
function has three arguments that have the same meaning as the first three arguments in the function FileOpenDlg
BOOL FileSaveDlg(PTSTR szFilter, PTSTR szDefExt, PTSTR szDlgTitle);
Return values from Open/Save functions reflect successful or unsuccessful selection.
I have added a small demo program just to demonstrate how it should work in a real application.
About the author
Denis Ponomarenko is a Ukrainian developer, now working in United Arab Emirates, Sharjah
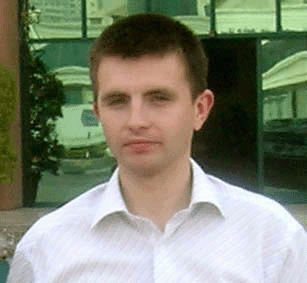