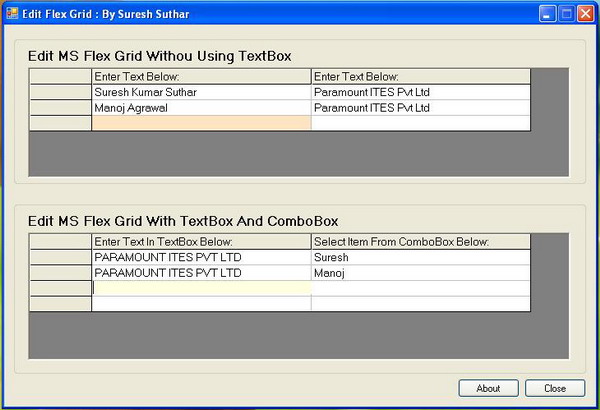
Introduction
This is my second article on CodeProject. This is about how to edit an MS Flex Grid. I was using a TextBox
and ComboBox
to edit a flex grid at run time. For that, I had to add a reference to Microsoft.VisualBasic.Compatibility
and some complex coding to move the TextBox
or ComboBox
on the grid. This is a good idea if some one wants to edit a grid with some predefined items using a ComboBox
.
But if you want to edit text, then there is a simple way to do it: use the Text
property of the MS Flex Grid control.
Using the code
To edit the MS Flex Grid without using a TextBox
, we have to use the Text
property and the KeyPress
event of the MS Flex Grid control.
Private Sub AxMSFlexGrid1_KeyPressEvent(ByVal sender As Object, _
ByVal e As AxMSFlexGridLib.DMSFlexGridEvents_KeyPressEvent) _
Handles AxMSFlexGrid1.KeyPressEvent
Select Case e.keyAscii
Case 30 To 136
AxMSFlexGrid1.Text += Chr(e.keyAscii)
Case 8
If AxMSFlexGrid1.Text <> "" Then
AxMSFlexGrid1.Text = Mid(AxMSFlexGrid1.Text, 1, _
Len(AxMSFlexGrid1.Text) - 1)
Case 13 And AxMSFlexGrid1.Col <> 2
AxMSFlexGrid1.Col = 2
Case 13 And AxMSFlexGrid1.Col = 2
AxMSFlexGrid1.Rows = AxMSFlexGrid1.Rows + 1
AxMSFlexGrid1.Col = 1
AxMSFlexGrid1.Row = AxMSFlexGrid1.Row + 1
End Select
End Sub
Using the second technique, I used a function name moveTextBox
to move the TextBox
to the appropriate position, and to set the text in the grid, I used the Text
property of the grid control.
Private Sub moveTextBox()
TxtEntry.Visible = True
TxtEntry.Left = VB6.TwipsToPixelsX(AxMSFlexGrid2.CellLeft + _
VB6.TwipsToPixelsX(AxMSFlexGrid2.Left)) + 17
TxtEntry.Top = VB6.TwipsToPixelsY(AxMSFlexGrid2.CellTop + _
VB6.TwipsToPixelsY(AxMSFlexGrid2.Top)) + AxMSFlexGrid2.Top
TxtEntry.Width = VB6.TwipsToPixelsX(AxMSFlexGrid2.CellWidth)
TxtEntry.Height = VB6.TwipsToPixelsY(AxMSFlexGrid2.CellHeight)
TxtEntry.BringToFront()
TxtEntry.Focus()
End Sub
On the TextBox
's KeyDown
event, I assigned the TextBox
's Text
property to the Flex Grid's Text
property.
Private Sub TxtEntry_KeyDownEvent(ByVal sender As Object, _
ByVal e As System.Windows.Forms.KeyEventArgs) _
Handles TxtEntry.KeyDown
If AxMSFlexGrid2.Col = 1 Then
If e.KeyCode = Keys.Enter Then
AxMSFlexGrid2.Text = TxtEntry.Text
TxtEntry.Clear()
AxMSFlexGrid2.Col = 2
End If
End If
End Sub
On the EnterCell
event of the grid control, I have called this function:
Private Sub AxMSFlexGrid2_EnterCell(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles AxMSFlexGrid2.EnterCell
If AxMSFlexGrid2.Col = 2 Then
Call moveCombo()
If AxMSFlexGrid2.get_TextMatrix(AxMSFlexGrid2.Row, 2) <> "" Then
CmbEntry.Text = AxMSFlexGrid2.get_TextMatrix(AxMSFlexGrid2.Row, 2).ToString
Else
CmbEntry.Text = ""
End If
Else
CmbEntry.Visible = False
End If
If AxMSFlexGrid2.Col = 1 Then
Call moveTextBox()
If AxMSFlexGrid2.get_TextMatrix(AxMSFlexGrid2.Row, 1) <> "" Then
TxtEntry.Text = AxMSFlexGrid2.get_TextMatrix(AxMSFlexGrid2.Row, 1).ToString
Else
TxtEntry.Clear()
End If
Else
TxtEntry.Visible = False
End If
End Sub
Thanks and happy coding..