Introduction
This article is for someone who, like me, has coded before -- but never for Apple technologies.
A good friend gave me an iPhone primer book. So I installed Xcode and dived in. It has been a rocky six weeks. While all of the problems I have had to deal with are still fresh in my head, I will try to make the path a little easier for the next traveler. If you, like me, lack patience, this guide is for you.
I see a lot of books reinventing the wheel by writing their own Hello World applications, encouraging the reader to depend on the book. This is naughty. Some of the Apple documentation is very good!
Resources
- Register with Apple ( 100 bucks )
First of all, register as an Apple developer on their website http://developer.apple.com/. Once in, you have access to their developer videos, you can download and install Xcode, you have access to their forums. - IRC -- irc.freenode.net#iphonedev
This channel is very active -- ask a good question and they will help you. as with any IRC chat room, asking a question without checking the obvious places first will not endear you to the community. (www.mibbit.com if you don't have a client). - Stack Overflow
Is it a wiki? is it a forum? No, it is... Stack overflow! A groundbreaking new technology with very rapid turnaround -- often your questions will get answered almost as fast as asking on IRC. - Apple's doc
...of which Xcode downloads and keeps a local copy. - Vidz!
Stanford University -- CS 193P iPhone Application Development
All of these 20 lectures are available on video, though not from that site (but DO get the source code from that site!). You can get them from iTunes -> iTunesU -> ...(then you have to hunt for it). I recommend downloading all of these videos and flicking through them every time you have a spare half-hour. I like to skip to the end part where he puts it all together with a sample application, and then move back through the video if I am curious enough about anything I saw. My concentration span gives out if I just try to watch them all the way through.
WWDC09/10
Similarly for the WWDC09/10 videos on the Apple developer site. I recommend downloading everything and skipping through, letting the PowerPoint slides catch your eye, and you will gradually build your neural matrix. These videos are mainly for people already fluent with the technology, but some of them are designed for getting started.
- Use the Source, Luke
create a folder somewhere .../source/samples/ and into it download lots of juicy source code.
Apple developer documentation samples
there are hundreds of samples (100? 1000? I forget) on the Apple developer site. Some of them are listed, but some of them aren't! and you only find them by random chance on Google, or by pulling up the help screen on some keyword from within Xcode, and low and behold it has something under ' related sample code '.
WWDC10 source code
Can't remember off the top of my head how to get this -- Google knows!
GitHub
Again, thousands of projects people have open sourced. unfortunately there is no tidy way to browse through these projects, the best you will get his throwing 'iphone' into the search field and looking through 1000 or so entries. I really hope that somebody makes a wiki for organising these, and eventually they become more accessible.
- Google & Blogs
I recommend some GoogleFu to look for getting started links: here is one: http://stackoverflow.com/questions/1939/how-to-articles-for-iphone-development-objective-c Once you have got your teeth into it, probably about three months down the line (which is where I am at now) you will probably want to start following all of the top bloggers & get twitter so you get notified whenever one of them tweets out a new article. because you will always be stuck somewhere, and generally someone has already been stuck there and figured it out and wrote it up. http://stackoverflow.com/questions/232570/what-are-the-best-cocoa-touch-iphone-programming-blogs is a list for examples. Is missing some great ones, and contains some awful ones. So, use your discrimination. - Books
Also, if you are new to coding, you need to go: C -> ObjC -> iPhone programming
Bookwise, that translates as:
The C Programming Language , the C Bible, written by Dennis Ritchie -- the guy that wrote C. Everyone who has read it tells everyone else to read it. I read it. Read it!
Programming in Objective-C 2.0 appears to be the Bible for Objective-C.
The iPhone Developer's Cookbook: Building Applications with the iPhone 3.0 SDK by Erica Sadun.
Xcode's Documentation - Setting Some Bookmarks
This documentation is a bit of a mess -- despite it physically being installing onto your hard drive when you install Xcode, Xcode cannot find this version, and instead tries to download the latest help files which are 300 megs. You can see this in action: Xcode -> Preferences -> Documentation. If you have a slow Internet pipe, that's just too bad -- these downloads don't seem to support resuming. I had to leave my MacBook at the local Internet cafe overnight.
Let's take a moment to customise help ...
From Xcode, Help -> Developer documentation should take you to the quick start page; bookmark it, as it's easy to lose it.
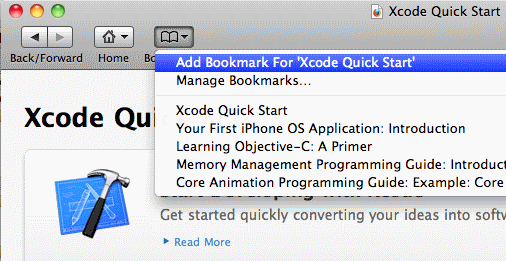
See if you can find a local copy of the Hello World tutorial. Here is the web-based version, at the time of writing: Your First iOS Application.
If your brain works like mine and jumps all over the place, you may miss the home pull down.

You can see the Hello World app guide highlighted there. Bookmark it.
I recommend you also find the Objective-C guide, and bookmark that. Notice that I have restricted the doc set, otherwise it throws up too much junk. At the bottom of this primer is a link to a full Objective-C reference. If you are new to Objective-C, it's worth skimming this so that you know where to look up weird looking syntax.

As a last bookmark for now, find the memory management guide. Don't bother reading it all for now -- just have it ready so that when your code crashes in two weeks' time, with a totally unhelpful 'bad access' error, you can navigate to the cardinal rule:

Four bookmarks -- that's enough for now!
A Little Preliminary Reading
Next, I recommend looking through the videos in the QuickStart -- that shows you how to use context sensitive help etc., and having a little skim of the Objective-C guide -- if you don't know any Objective-C, you will just get confused by the tutorial. If you are new to Objective C, you need to know: it is an extension of C. You can write in C code all the way if you want. But then you would waste thousands of hours of recoding classes to draw text on the screen that Apple has already written in their SDK. In fact, you can write C++. You can mix all of the different dialects of C and C++ with Objective-C.
Hello World Tutorial
Next, crack open the Hello World tutorial (the second link we created) and go through it. It's very good. For the most part. Not entirely -- it creates confusion in the way it names properties. I have corrected this -- you can download my version of this Hello World project from the link above.
But before I discuss the changes I have made, I want to make a quick point about colour schemes.
Colour Schemes
I recommend setting a good colour scheme that lets you see what is what. For example, rather than simply trying to get something looking pretty, I have clustered user defined objects into the red orange yellow spectrum, with the standard objects in the blue purple end. Here's a screenshot of what I'm using:

You can see that vc
is my own custom class I have created, coming up in red. UIWindow
is one of Apple's classes. Instance variables show up in yellow.

Here you can see: for Apple's classes, purple methods come from blue classes. For my classes, orange methods come from red classes. [vc alloc]
has red vc
, since I created that class, but I inherited it from NSObject
(which almost every class should do). Hence alloc
is in purple -- it is a method of NSObject
.
Feel free to download my colour scheme (from the link above) and stick it in ~/Library/Application Support/Xcode/Color Themes (you will probably need to create a folder) and Xcode -> Preferences -> Fonts and colours -> Colour scheme should pick it up. But I do recommend you go there first and look at your options.
Properties
I will simply list the two relevant source files, as the code is commented generously. I prefer to comment the code, rather than discuss in the article, as it lets you step through and read without referring back and forth. In a nutshell, the Apple tutorial creates a double confusion through its naming of properties. All I do in my code is change the names, thus making clear distinctions between the view controller class (vc
), the application delegate's instance of this class (_myVc
), and the application delegate's getter and setter methods for getting and setting this instance (myVc
, setMyVc
).
@class vc;
@interface wndAppDelegate : NSObject <UIApplicationDelegate>
{
UIWindow *_window;
vc *_myVc;
}
@property (nonatomic, retain) IBOutlet UIWindow *window;
@property (nonatomic, retain) vc *myVc;
@end
#import "wndAppDelegate.h"
#import "vc.h"
@implementation wndAppDelegate
@synthesize window = _window;
@synthesize myVc = _myVc;
#pragma mark -
#pragma mark Application lifecycle
- (BOOL)application:(UIApplication *)application
didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
{
vc *tmp = [vc alloc];
NSLog(@"vc *tmp = [vc alloc]; // [tmp retainCount] now: %d",
[tmp retainCount] );
[tmp initWithNibName:@"vc"
bundle:[NSBundle mainBundle]];
[self setMyVc: tmp];
if (0.0)
self.myVc = tmp;
NSLog(@"[self setMyVc: tmp]; // [tmp retainCount] now: %d",
[tmp retainCount] );
if (NO+NO+NO*1000)
_myVc = tmp;
[tmp release];
NSLog(@"[tmp release]; // [tmp retainCount] now: %d",
[tmp retainCount] );
}
UIView* myV = self.myVc.view;
[self.window addSubview:myV];
[self.window makeKeyAndVisible];
return YES;
}
- (void)dealloc
{
[self.myVc release];
[self.window release];
[super dealloc];
}
Where to go next?
I recommend checking out the developer videos available to you when you log into the Apple developer website. You can also find a ton of sample source code -- you shouldn't have to look too far to find it.