Introduction
Microsoft POS for .NET provides .NET applications with simple and consistent interfaces for communicating with well over thirty Point of Service (POS) peripheral devices. The list of devices includes barcode scanners, magnetic stripe readers (MSR), Line Displays, PIN Pads, RFID readers, Biometric readers, receipt printers, cash drawers, and many others. POS for .NET is Microsoft’s implementation of the industry’s Unified Point of Service (UPOS) standard. POS for .NET makes it easy for both Independent Software Vendors and Independent Hardware Vendors to support a broad range of peripherals using Microsoft .NET. In addition, Microsoft POS for .NET provides many advantages to the ISV and end customer above and beyond Unified POS.
Microsoft Point of Service for .NET is available as a free download from Microsoft.
What to Do
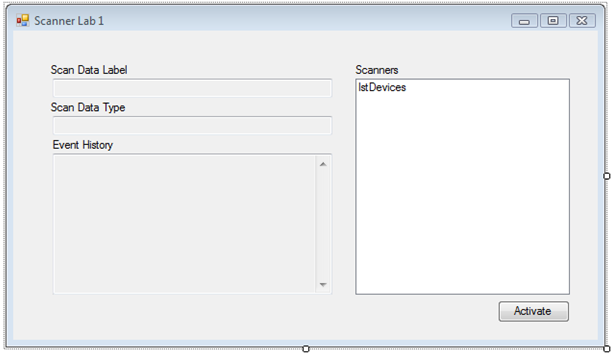
- Open Microsoft Visual C# 2008 Express Edition (or Visual Studio 2008 if available).
- Create a new project file:
- Select File->New->Project
- Select “Windows Forms Application” project under “Visual C# - Windows”
- Name the project “
ScannerSampleLab1
” and click “OK”
- The
Microsoft.PointOfService
assembly defines programming interface contracts between POS applications and service objects used to interface and control the many devices supported by POS for .NET. Add a reference to this assembly to the project:
- Right-click on “References” in the solution explorer, and click “Add Reference…”
- In the dialog box that pops up, select the “Browse” tab
- Select the reference “Microsoft.PointOfService.dll” file by navigating to the folder "%ProgramFiles%\Microsoft Point Of Service\SDK” where it is contained
- Click “OK” to add the reference.
- Using the property window, change the following properties for the main form as indicated:
- Name: ScannerLab1
- StartPosition: CenterScreen
- Text: Scanner Lab 1
- Feel free to adjust the size of the form as desired any time during the exercise
- While in the form designer view, use the toolbox common controls to add labels to the form with the following text values (see figure 1 for the general layout of the form):
- Scan Data Label
- Scan Data Type
- Event History
- Scanners
- Add a Text Box for the “Scan Data Label” label and set the properties as follows:
- Name: txtScanDataLabel
- ReadOnly: True
- Adjust the size of the textbox as desired
- Add a Text Box for the “Scan Data Type” label and set the properties as follows:
- Name: txtScanDataType
- ReadOnly: True
- Adjust the size of the textbox as desired.
- Add a TextBox for the “Event History” label and set the properties as follows:
- Name: txtEventHistory
- ReadOnly: True
- Multiline: True
- ScrollBars: Vertical
- Adjust the size of the textbox as desired
- Add a
ListBox
for the “Scanners
” label and set the properties as follows:
- Name: lstDevices
- Adjust the size and position as desired.
- Add a Binding source (available under Toolbox->Data) to the form and set the properties as follows:
- Name: scannerBindingSource
- Add a button to the form with the following properties:
- Name: btnActivateDevice
- Text: Activate
- Adjust the size of the button as desired
- Create a “Load” event handler for the main form. Don't worry about the code to go in this method for now, that will be done later on.
- Create a “Click” event handler for the “Activate” button. Again, don't worry about the code for this method as it will be added later on.
Verification
Build and run the project to verify that all of the steps were completed satisfactorily and that the UI looks similar to Figure 1 – Form UI Layout:
- From Visual Studio Select Debug->Start Debugging or Press the ‘F5’ key to start the debugger
- View the UI layout
- Press the Close (X) button when done.
Exercise 2
Developing a POS Application
This exercise will start to add POS functionality to the application created in the previous exercise. In this exercise, you will learn how easy it is to use POS for .NET in your applications, thus allowing you to easily interface with POS peripherals, such as a barcode scanner. You will be introduced to the ‘POSExplorer
’ class which will allow you to explore the system for available POS peripherals. You will enhance the POS application to discover all available scanners and to respond to events from a barcode scanner (or a simulated barcode scanner). Finally, you will enhance the POS application to read and display data from the barcode scanner.
Overview
- Add required
using
statements
- Introduction to ‘
POSExplorer
’
- Use ‘
POSExplorer
’ to discover all available barcode scanners
- Use ‘
POSExplorer
’ to interface with one of the available barcode scanners
Instructions
- Open the form1.cs file to view the code-behind the designer view. This can be done by right-clicking on the form and selecting “View Code.”
- Add a few
using
statements to make life a bit easier:
using Microsoft.PointOfService;
using System.Collections;
Note: Microsoft.PointOfService
is the main assembly reference for POS for .NET.
- POS for .NET provides the ‘
POSExplorer
’ class. This class can be used to perform queries on the system of available POS device peripherals, to instantiate service objects which act as device drivers for the peripherals, and to receive notification of Plug-n-Play (PnP) events for POS devices. The ‘Scanner
’ class represents a service object for barcode scanner peripherals. The instance of the ‘Scanner
’ is device specific and is provided by the ‘POSExplorer
’. Add member fields to the form for the explorer, the active scanner, and a running list of available scanners:
private PosExplorer explorer;
private ArrayList scannerList;
private Scanner activeScanner;
- Before hooking up the events, a few utility methods are in order. The first utility method will be used to log activities and display them in the UI as they occur. The ‘
txtEventHistory
’ control will be used to maintain and display this log. So the first method will add the new event to the top of the log while maintaining history of past events. Add an ‘UpdateEventHistory
’ method to maintain this log:
private void UpdateEventHistory
{
txtEventHistory.Text = newEvent + System.Environment.NewLine +
txtEventHistory.Text;
}
- The next utility method will be used to deactivate the active scanner (if any). Because calls to the service objects exposed by various devices may result in a ‘
PosControlException
’, these will be handled by simply logging the exception if it is raised. After the active scanner has been closed, code should be added to set the ‘activeScanner
’ variable to null
to indicate that there is no longer an active scanner:
private void DeactivateScanner()
{
if (activeScanner != null)
{
UpdateEventHistory(Deactivate Current Scanner);
try
{
activeScanner.Close();
}
catch (PosControlException)
{
UpdateEventHistory(Close Failed);
}
finally
{
activeScanner = null;
}
}
}
- ‘
DeviceInfo
’ class is used by POS for .NET to identify and represent information about an available device. This class can be provided to the ‘PosExplorer
’ in order to instantiate a service object for the device identified by the ‘DeviceInfo
’ class. A utility method is needed to activate a scanner identified by ‘DeviceInfo
’. This method will first verify that the ‘DeviceInfo
’ provided identifies a device other than the one that is already in use. It will then deactivate the current scanner, create a new instance member for the active scanner, open the scanner, claim the scanner for exclusive use by the application, enable the device, subscribe to events from the device, and enable data events for the device. If any exception occurs, this will be logged and the active scanner will be set to none. All of this can be done as follows:
private void ActivateScanner(DeviceInfo selectedScanner)
{
if (selectedScanner != null && !selectedScanner.IsDeviceInfoOf(activeScanner))
{
DeactivateScanner();
UpdateEventHistory(string.Format(Activate Scanner: {0}",
selectedScanner.ServiceObjectName));
try
{
activeScanner = (Scanner)explorer.CreateInstance(selectedScanner);
activeScanner.Open();
activeScanner.Claim(1000);
activeScanner.DeviceEnabled = true;
activeScanner.DataEvent += newDataEventHandler(activeScanner_DataEvent);
activeScanner.ErrorEvent += new DeviceErrorEventHandler(
activeScanner_ErrorEvent);
activeScanner.DecodeData = true;
activeScanner.DataEventEnabled = true;
}
catch (PosControlException)
{
// Log error and set the active scanner to none
UpdateEventHistory(string.Format(Activation Failed: {0}",
selectedScanner.ServiceObjectName));
activeScanner = null;
}
}
}
- It is now time to create an instance of a ‘
POSExplorer
’. The explorer will be used to find the list of available devices and instantiate service objects (SO) for the devices as needed. It can also be used to provide PnP events as POS devices that are added and removed from the system. Events from devices may be generated in a thread asynchronously outside of the UI. However, you want all of the events to be processed within the UI thread. Luckily POS explore makes this easy, simply identify the form in the constructor and the ‘POSExplorer
’ will ensure that all events are marshaled into the UI thread. Add the code to create the instance inside the Form’s load event as follows:
explorer = new PosExplorer(this);
- Within the Forms load event, use the explorer to create a list of ‘
DeviceInfo
’ for all available POS scanners. This list will be saved in an ArrayList
and bound to the data source for the ‘lstDevices
’ control via the binding source that was created earlier. Finally, the ‘lstDevices
’ will set the DisplayMember
to indicate that only the service object name should be displayed in the UI:
scannerList = new ArrayList(explorer.GetDevices(Scanner));
scannerBindingSource.DataSource = scannerList;
lstDevices.DataSource = scannerBindingSource;
lstDevices.DisplayMember = ServiceObjectName;
- Add code to the bottom of the forms load event to activate the first available scanner (if any) identified by the ‘
lstDevices
’ control. Remember that the ‘lsDevices
’ is bound to a list of ‘DeviceInfo
’ objects contained in the scannerList
. The code is as follows:
if (lstDevices.SelectedItem != null)
{
ActivateScanner((DeviceInfo)lstDevices.SelectedItem);
}
- Modify the button click code so that it will activate the selected scanner using the same code in the previous step:
if(lstDevices.SelectedItem != null)
{
ActivateScanner((DeviceInfo)lstDevices.SelectedItem);
}
- Upon receiving a scan event from the barcode scanner, the following actions are desired: log the data event, update the label and data type information with the data provided by the scanner, re-enable the data event for any subsequent scans, and log any exceptions that may have occurred. In the event handler, one is faced with a choice of using the sender object to identify the scanner, or directly using the active scanner. In order to support multiple scanners in the same event handler, the later choice would be the desired approach. For simplicity, however, go ahead and use the ‘
activeScanner
’ directly:
void activeScanner_DataEvent(object sender, DataEventArgs e)
{
UpdateEventHistory(Data Event);
ASCIIEncoding encoder = new ASCIIEncoding();
try
{
txtScanDataLabel.Text = encoder.GetString(activeScanner.ScanDataLabel);
txtScanDataType.Text = activeScanner.ScanDataType.ToString();
activeScanner.DataEventEnabled = true;
}
catch (PosControlException)
{
UpdateEventHistory(DataEvent Operation Failed);
}
}
- In the event that an error event is received from the scanner, simply log the occurrence and re-enable the scanner for future events:
void activeScanner_ErrorEvent(object sender, DeviceErrorEventArgs e)
{
UpdateEventHistory(Error Event);
try
{
activeScanner.DataEventEnabled = true;
}
catch (PosControlException)
{
UpdateEventHistory(ErrorEvent Operation Failed);
}
}
Verification
Now it is time to verify the application. POS for .NET includes a set of “simulated” devices including a “Microsoft Scanner Simulated” that can be used to simulate a physical scanner. This will be used here to verify the application. Later on, you will have the opportunity to develop your own scanner service object that will interface with a physical scanner (normally, only IHV would need to write a managed service object, but they are simple enough even with relatively little hardware experience as POS for .NET does much of the heavy lifting of implementing in accordance with the Unified POS standard).
- Compile and run the Scanner Lab 1 application.
- The “Microsoft Scanner Simulator” should be selected by default, if not, select this scanner and press the “Activate” button.
- A window is displayed titled, “Microsoft Scanner Simulator” that is used to simulate a physical scanner. Go ahead and try sending different scan events to your application while entering different barcode values.