Introduction
This is my very first article to any open source site. Since I've gotten a lot of useful articles from this site, I'll be glad if I can to contribute to this great site. This is a simple application to get some knowledge of using the OWC11 in a .NET application for chart representation.
Office Web Components (OWC) are a group of OLE classes implemented as ActiveX controls in Microsoft Office 2000, Office XP, and Office 2003. The Office Web Components have been discontinued in Office 2007, and are not included, except as a part of the Office Project Server 2007. OWC can be used by any COM-compliant Component Object Model programming language.
Background
Any application that uses the Office Web Components 11 requires that the OWC 11 package be installed on the system. OWC 11 is a separate download available on the Microsoft Website or in the Microsoft Office 2003 Installation Kit. Office Web Components 11 does not require support from Microsoft Office.
Office 2003 Web Components do not provide event handling by default. We need to do some alterations. So, please refer this link first: HOW TO: Handle Events for the Office 2003 Web Components in Visual Studio.NET. Using this link, we can get AxOWC11.dll. Add this newly-compiled AxOWC11.dll to the Toolbox. Now, the Toolbox contains the AxChartSpace
control, the AxPivotTable
control, and the AxSpreadsheet
control.
Column Chart
A column chart displays values and series groups as sets of vertical columns that are grouped by category. Values are represented by the height of the columns as measured by the y-axis. Category labels are displayed on the x-axis. Column charts are typically used to compare values between categories. There are three types of column charts:
- Column
- Stacked Column
- 100% Stacked Column
A column chart displays series as individual columns, grouped by category. The height of each column is determined by the series value.
A stacked column chart displays all series stacked in a single column for each category. The height of each column is determined by the total of all the series values for the category.
A 100% stacked column chart displays all series stacked in a single column for each category. The height of each column is always the full height of the chart. The series values are displayed as percentages of each column.
The abbove definition of the column chart is picked from the MSDN. For more details, please refer: Column Chart.
Steps for Creating the Chart
First of all, from the Toolbox property window, select the AxChartSpace
control and drag it on to the design surface. After this step, our form looks like the following:
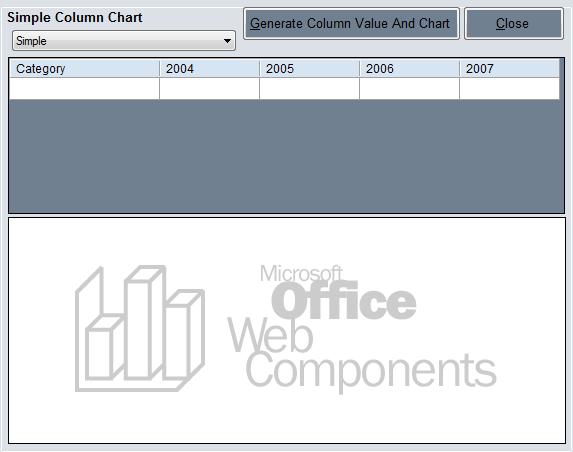
Figure-1
Now, in the code window, write the following statement:
Imports owc11 = Microsoft.Office.Interop.Owc11
Since the code only shows how can we create the Column Chart, the code is not efficient. Here, we are skipping some statements which fill dummy data in the DataGridView
. Now, we declare two variables for holding data for the categories (related to the x-axis) and the values (related to the y-axis):
Dim categories(3)
For i As Integer = 0 To 3
categories(i) = DataGridView1.Columns(i + 1).HeaderText.ToString
Next
Dim values(3)
Dim chConstants
For clearing the contents of the chart workspace, use the following statement. The following statement removes any old charts that may already exist and adds a chart to the chart space.
AxChartSpace1.Clear()
AxChartSpace1.Charts.Add()
chConstants = AxChartSpace1.Constants
Now, add series to the existing chart. Using the following statement, you can add as much series as you want.
AxChartSpace1.Charts(0).SeriesCollection.Add()
Now, we supply the data to each series as follows:
Dim MaxTotal As Integer = 0
For j As Integer = 0 To DataGridView1.Rows.Count - 2
AxChartSpace1.Charts(0).SeriesCollection(j).SetData(chConstants.chDimCategories, _
chConstants.chDataLiteral, categories)
For i As Integer = 1 To DataGridView1.Columns.Count - 1
values(i - 1) = Val(DataGridView1.Rows(j).Cells(i).Value)
Next
AxChartSpace1.Charts(0).SeriesCollection(j).SetData(chConstants.chDimValues, _
chConstants.chDataLiteral, values)
AxChartSpace1.Charts(0).SeriesCollection(j).Caption = _
DataGridView1.Rows(j).Cells(0).Value
MaxTotal = MaxTotal + Val(DataGridView1.Rows(j).Cells(4).Value)
Next
We can also set the color for each series as follows:
AxChartSpace1.Charts(0).SeriesCollection(0).Interior.Color = "DarkOrange"
AxChartSpace1.Charts(0).SeriesCollection(1).Interior.Color = "Cyan"
AxChartSpace1.Charts(0).SeriesCollection(2).Interior.Color = "Yellow"
AxChartSpace1.Charts(0).SeriesCollection(3).Interior.Color = "Red"
AxChartSpace1.Charts(0).SeriesCollection(4).Interior.Color = "Black"
Notice one thing in the above statements. I supply the color for a series as a string. It does not support the Color
enum. At this point, we decide which type of chart we want (simple, stacked, or 100 % stacked column). Also, we can draw charts with 3D effect. Use the following statement for a simple and a 3D stacked column.
If cboChartType.Text = "3D" Then
AxChartSpace1.Charts(0).Type = owc11.ChartChartTypeEnum.chChartTypeColumnStacked3D
Else
AxChartSpace1.Charts(0).Type = owc11.ChartChartTypeEnum.chChartTypeColumnStacked
(See Figure-3)
End If

Figure-2

Figure-3
And for a simple and 3D 100% stacked column, use the following statement:
If cboChartType.Text = "3D" Then
AxChartSpace1.Charts(0).Type = owc11.ChartChartTypeEnum.chChartTypeColumnStacked1003D
(see Figure-4)
Else
AxChartSpace1.Charts(0).Type = owc11.ChartChartTypeEnum.chChartTypeColumnStacked100
(see Figure-5)
End If

Figure-4

Figure-5
And finally, for a simple and 3D column, use the following statement:
If cboChartType.Text = "3D" Then
AxChartSpace1.Charts(0).Type = owc11.ChartChartTypeEnum.chChartTypeColumn3D
(see Figure-6)
End If

Figure-6

Figure-7
Along with the above statements, the way we provide the data to all the charts is also responsible for getting the correct results. In all the charts, the variable MaxTotal
also plays a role which decides the scaling of the chart.
AxChartSpace1.Charts(0).Axes(1).Scaling.Maximum = MaxTotal
AxChartSpace1.Charts(0).Axes(1).MajorUnit = MaxTotal / 4
AxChartSpace1.Charts(0).Axes(1).Scaling.Minimum = 0
Now, in the final step, we assign proper title and font to the chart axes, as follows:
AxChartSpace1.Charts(0).Axes(0).HasTitle = True
AxChartSpace1.Charts(0).Axes(0).Title.Caption = "Time Range"
AxChartSpace1.Charts(0).Axes(0).Title.Font.Name = "Arial"
AxChartSpace1.Charts(0).Axes(0).Title.Font.Size = 9
AxChartSpace1.Charts(0).Axes(1).HasTitle = True
AxChartSpace1.Charts(0).Axes(1).Title.Caption = "Number of Members"
AxChartSpace1.Charts(0).Axes(1).Title.Font.Name = "Arial"
AxChartSpace1.Charts(0).Axes(1).Title.Font.Size = 9
Points of Interest
When I use the OWC 11 chart component in real applications, I get some strange results. If we declare the variable categories
as follows:
Dim categories(3) As String
then the chart object does not display any series. However, the legend shows all the series names. One more thing, if we change the series color before supplying the data, then the series does not display the proper color.
I hope you've enjoyed this little tutorial. In future, I will provide you a lot more stuff. And, I am expecting your valuable suggestions.
Finally, please excuse me for my English.