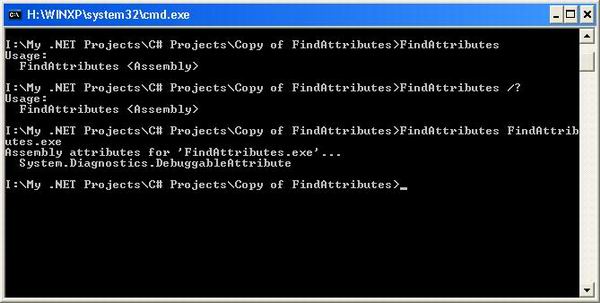
Introduction
Reflection allows you to programmatically inspect an assembly, and get information about the assembly, including all object types contained within. This information includes the attributes you have added to those types. The reflection objects reside within the System.Reflection
namespace.
This demo program will inspect an assembly and display a list of all attributes defined on the assembly. The entire source code is downloadable and I will explain how it works.
Source Code Explanation
The code first checks the parameters passed to the command-line - if none are supplied, or if the user types FindAttributes /? then the Usage()
method will be called, which will display a simple command usage summary:
if (args.Length == 0)
Usage();
else if((args.Length == 1)&&(args[0] == "/?"))
Usage();
Next, we reconstitute the command-line arguments into a single string. The reason for this is that it's common to have spaces in directory names, such as "Program Files", and this would be considered as two arguments by virtue of there being a space. So, we iterate through all the arguments stitching them back into a single string, and use this as the name of the assembly to load:
foreach(string arg in args)
{
if(assemblyName == null)
assemblyName = arg;
else
assemblyName = string.Format("{0} {1}", assemblyName, arg);
}
We then attempt to load the assembly, and retrieve all custom attributes defined on that assembly with the GetCustomAttributes()
method:
Assembly a = Assembly.LoadFrom(assemblyName);
object[] attributes = a.GetCustomAttributes(true) ;
if(attributes.Length > 0)
{
Console.WriteLine("Assembly attributes for '{0}'...", assemblyName);
foreach(object o in attributes)
Console.WriteLine(" {0}", o.ToString());
}
Any attributes that are found are output to the console.
You can build the executable of the source code by using the command-line compiler like this:
>csc FindAttributes.cs
Running the Program
When you test the program against the FindAttributes.exe file, an attribute called DebuggableAttribute
will be displayed, as shown in the screen shot. Although this attribute was not specified, it has been added by the C# compiler, and you will find that most of your executables have this attribute.
Additional Notes
You can alter the code as appropriate to add on as many assembly attributes as you wish. As an example, try modifying the source code by un-commenting the line after the using
directives at the beginning of the listing.
When you recompile and run the code, you will see an additional attribute has been added to the list, the System.Reflection.AssemblyTitleAttribute
.
Please check Karli Watson's Beginning Visual C# [ISBN 1-86100-758-2] by Wrox Press for more information.
Ahmed had his M.S. Degree in Electrical and Computer Engineering in USA and the B.Sc. Degree in Automatic Control and Computer Systems Engineering in Egypt. He programmed with Assembly, Fortran77, Prolog, C/C++, Microsoft Visual Basic, Microsoft Visual C++, ATL/COM, Microsoft Visual C#, VB.NET, ASP.NET, AJAX, SharePoint 2007/2010, Microsoft Commerce Server, and MATLAB and Maple for technical computing. His programming experience is about 15+ years and he is a Microsoft Certified Professional. He also has a working experience in Database technologies and administration of SQL Server databases on Windows NT/2000/2003/2008 Windows Server platforms. Ahmed is interested in developing enterprise business solutions and has participated in many business, services and consultancy projects.