Introduction
The idea is to draw pictures into sounds. In particular, draw pictures into the spectral representation of the sound. A sound can be represented in many ways. In particular, it can be drawn with Amplitude-Time representation (Waveform) and Frequency-Time representation (Spectrum). The first shows the amplitude of the sound in time:
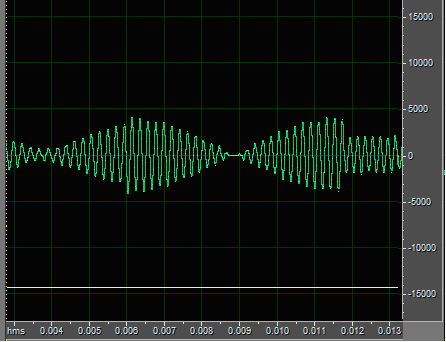
The X-axis is the time while the Y-axis is the amplitude of the sound. The next kind of view shows the audio signal as components of different frequencies:

Here, the X-axis is time while the Y-axis is the frequency. In particular, lighter colors (white) means higher value of the component of the specific frequency, while darker colors (black) means a lower component. Here, it’s possible to see how the sound changes in time and frequency.
The Fast Fourier Transform
The Fourier Transform is an operation that transforms a signal (in our case, the audio signal) into its frequency domain representation. So, it is possible to see which frequencies are present in the input signal. The FFT is an algorithm that computes the Fourier Transform efficiently.

The inverse operation (Inverse Fast Fourier Transform) takes data from the frequency domain and gives the values in the time domain. We can use this algorithm to draw a sound. Just imagine the draw as an input like if it was the spectral representation of the signal. Then, we apply the IFFT algorithm, and we retrieve the audio waveform which is used to create the output wave file.
The Program

The blackboard is the frequency-time representation of the audio signal. It is possible to paint into it using the color of the bar on the left side of the start button. Clicking the bar, it’s possible to change the color. The best results can be obtained using darker colors (like dark gray). When you click the Start button, the IFFT algorithm begins to compute data and the output audio file is generated.
Just draw something:

We can view the results with programs like Cooledit.

The Code
The program is written in C#. We use two fundamental libraries here:
- Wave File Library written by Garrett Hoofman. The library has been used to produce the Wav output file.
- A library that implements the IFFT algorithm.
using System;
using System.Collections.Generic;
using System.Text;
using System.Drawing;
using WaveLibrary;
namespace Img2Wav
{
class Core_Img2Wav
{
private const double MAX_DATA = +50;
private const double MIN_DATA = -50;
private const String NoInputBitmap = "No input bitmap";
public Bitmap InputBitmap { get; set; }
public WaveFile OutputWav { get; set; }
public void Start()
{
int NumSamples = InputBitmap.Width * InputBitmap.Height;
byte[] Samples = new byte[NumSamples];
OutputWav = new WaveFile(1, 16, 44000);
if (InputBitmap == null) throw new Exception(NoInputBitmap);
double[] data = new double[InputBitmap.Height];
int w = 0;
for (int i = 0; i < InputBitmap.Width; i++)
{
for (int j = 0; j < InputBitmap.Height; j++)
{
Color C = InputBitmap.GetPixel(i,j);
data[j] = (C.R + C.G + C.B ) / 3;
}
FFT_Img2Wav.inverse(data);
for (int x = 0; x < data.Length; x++)
{
Samples[w] = (byte)(MAX_DATA * data[x]);
w++;
}
}
OutputWav.SetData(Samples,NumSamples);
}
}
}
Angelo Gattuso graduated in November 2003 from "Politecnico di Torino" in Software Engineering. He is a Software Engineer with experience in projecting and developing Businness Applications. Areas of expertise include ASP .Net design and develompemnt of three-tier applications.
He has interests in music, reading and cooking even if he prefers eating.