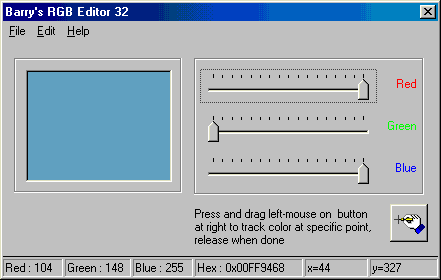
Introduction
This article demonstrates the technique of creating a RGB color editor, useful at web-page and software development time, to know the RGB code for different combinations of Red, Green and Blue colors. The article has two projects both independent, one was developed using the popular MFC and the other in Win32 (a.k.a. SDK). Both applications are identical in functionality and helps developers who are not very familiar with Win32 programming, to compare and see how easy it is to develop applications in Win32.
These applications also have an additional facility of tracking RGB color code of any point on the desktop in Hex and Red, Green and Blue codes, and x and y co-ordinates of the cursor location. You can also copy the codes to the clipboard making it easy to incorporate the color code in your source code.
Background
The idea for writing this application came from a similar utility I was using when developing some accounting applications in Power-Builder; this application is called RGB Calculator in Power-Builder, and another shareware utility for tracking color codes of images on screen. I combined functionality of both these utilities in these applications.
I added the Win32 application to the article since the MFC application was working as expected (refer the "Know problems" section), and not to show my programming capabilities, but I found similar problems with the Win32 application also.
Points of interest
Copying to clipboard
if(::OpenClipboard(NULL))
{
LPTSTR lptstrCopy;
HGLOBAL hglbCopy;
int cch = 30;
hglbCopy = GlobalAlloc(GMEM_DDESHARE,
(cch + 1) * sizeof(TCHAR));
if (hglbCopy == NULL)
{
CloseClipboard();
return;
}
char lpStr[30];
wsprintf(lpStr, "RGB(%d, %d , %d)" , m_Slider_Red.GetPos() ,
m_Slider_Green.GetPos(), m_Slider_Blue.GetPos());
lptstrCopy = (LPTSTR)GlobalLock(hglbCopy);
memcpy(lptstrCopy, lpStr, cch * sizeof(TCHAR));
lptstrCopy[cch] = (TCHAR) 0;
GlobalUnlock(hglbCopy);
::EmptyClipboard();
::SetClipboardData(CF_TEXT, hglbCopy);
::CloseClipboard();
}
Both these are dialog-based applications, but I have added tool and status bars to them through code like this:
Toolbar
TBBUTTON tb;
m_ToolBar.Create(
WS_CHILD|WS_VISIBLE|WS_BORDER|TBSTYLE_FLAT,
CRect(0,0,0,0), this, 0);
m_ToolBar.SetImageList(&m_pImageList);
tb.iBitmap = 0;
tb.iString = NULL;
tb.fsState = TBSTATE_ENABLED;
tb.fsStyle = TBSTYLE_BUTTON;
tb.idCommand = ID_FILE_EXIT;
m_ToolBar.AddButtons(1, &tb);
tb.iBitmap = 1;
tb.idCommand = ID_ABOUTBOX;
m_ToolBar.AddButtons(1, &tb);
Status bar
m_StatusBar.Create(WS_CHILD|WS_VISIBLE|SBT_OWNERDRAW,
CRect(0,0,0,0), this, 0);
int strPartDim[4]= {80, 160, 240, -1};
m_StatusBar.SetParts(4, strPartDim);
A point of irritation in a dialog-based MFC application is that when you press any key (specially Enter), the application closes. I have prevented it by overriding the following function:
BOOL CColorCoderDlg::PreTranslateMessage(MSG* pMsg)
{
if (pMsg->message == WM_KEYDOWN)
return FALSE;
return CDialog::PreTranslateMessage(pMsg);
}
The MFC application sets up a Windows hook whenever it needs to track color code and cursor positions of any location. It first changes the pointer cursor and sets it back to original when you release left-button. I found the hook unavoidable because if you change the mouse cursor without a hook, the changed cursor is only available within the client-area, not outside of it.
Windows hook function was not required in the Win32 application.
Known problems
- When tracking color codes and cursor location by pressing and dragging left-mouse button under Windows 98, Windows Me, the mouse cursor flickers to a very large extent (it virtually disappears for a second before reappearing), but under Windows XP (and maybe under Windows 2000) it seems fine, no prizes for guessing.
- In the Win32 application if you click on the close button (x) at the top-right of the main window, nothing happens. I have not added code to close the application for this message.
- Only RGB code is copied to clipboard, if you want the hex code to be copied to clipboard make changes in the source as shown in the function
OnCopytoClipboard()
.
Win32 applications
Some developers may be wondering, is development under Win32 easier, is it better then MFC. I am not competent enough to answer such questions but if I am asked to develop an application and I know I can easily develop it in either, I would settle for Win32. Win32 applications are robust, less prone to errors at run-time and you do not have to worry about "overridable" functions, as in MFC applications.
You are however required to know about Window messages in a big way since class-wizard used in MFC is not available here. Visual Studio's "Spy++" utility is the most useful tool available for tracking messages.
Note: I had to override 2 functions in the MFC application viz OnSetCursor(CWnd* pWnd, UINT nHitTest, UINT message)
and PreTranslateMessage(MSG* pMsg)
.
Spy++ utility
Spy++ is a utility which is part of Visual Studio (Professional Edition). I have only used it so far to track Windows messages, maybe it can be used for other purposes, if you want to track messages.
- Start you application (preferably not in full-screen).
- Start Spy++
- From Spy++ menu select Spy->Messages
- Press and drag left-button on the "Bullseye" icon (right of 'Finder Tool'), onto you application, select entire window or a control depending on you message requirement
- Your mouse cursor will change to "Bullseye" (round)
- Release mouse button
- Click Ok on Spy++
- Do not iconize Spy++
If you have a command button on your application and want to know what message will be sent and where.
Note the last message number in Spy++ (left-most number in list box) and then click on the command button in your application, a line (at times many) will be added to Spy++ list of messages.
Note: Spy++ can be used for all types of applications, be it in MFC, Win32 , ATL etc.
History