How to create selective Drag n Drop handler
I'm writing this code such that the user of my software can just drag n drop anything he wants and from anywhere. The software will decide what will do with the drag objects.
Introduction
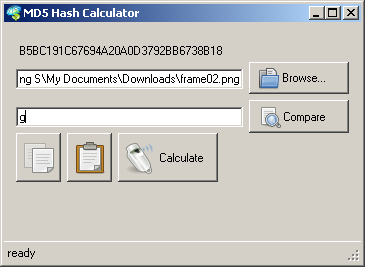
Background
Many software support drag n drop operation. But many of them need user to drag n drop to specified place.Using the code
To create drag n drop operation, we just need 3 important things to do:- Allow drop operation in specified control
- Write drag enter handler
- Write drag drop handler
1. Allow drop operation
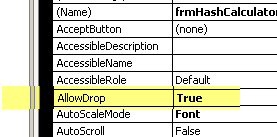
2. Write drag enter handler
This will enable the program to receive drag objects. For example, in this case, I make copied objects to dropped in my application. You can makes it like cut n paste if you want by specifyDragDropEffects.Move
instead of DragDropEffects.Copy
.
private void frmHashCalculator_DragEnter(object sender, DragEventArgs e)
{
e.Effect = DragDropEffects.Copy;
}
3. Write drop handler
this is the code where your program use the dropped object.private void frmHashCalculator_DragDrop(object sender, DragEventArgs e)
{
if (e.Data.GetDataPresent(DataFormats.FileDrop ,true ))
{
string[] lokasi = (string[])e.Data.GetData(DataFormats.FileDrop, true);
txtLink.Text = lokasi[0];
}
else if (e.Data.GetDataPresent(DataFormats.Text ,true ))
{
txtComparer.Text = e.Data.GetData(DataFormats.Text, true).ToString();
}
}
By checking what the data present type, we can decide what to do with specified data type. In this example, if user drag a file, then the file path will appear to file path section. If user drag a text, then the text will copied to text comparator section.
Points of Interest
Since my application is used to calculate MD5 hash of file, then I'll show you how easy to calculate MD5 hash.Cursor = Cursors.WaitCursor;
byte[] retval;
StringBuilder sb;
try
{
FileInfo fi = new FileInfo(txtLink.Text); //this from file path section
if (fi.Exists) //check if file exist of course
{
file= fi.Open(FileMode.Open, FileAccess.Read);
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
//throw;
}
if (file != null)
{
MD5 md5 = new MD5CryptoServiceProvider(); //just easy as 2 lines of code
retval = md5.ComputeHash(file);
file.Close(); // close the streaam so that the file unlocked
sb = new StringBuilder(); //since the result is char array, we can create
for (int i = 0; i < retval.Length; i++) //string builder for this
{
sb.Append(retval[i].ToString("X2"));
}
lblHash.Text = sb.ToString();
}
Cursor = Cursors.Default ;
after obtain the result, we can simply comparing the result with text comparator section