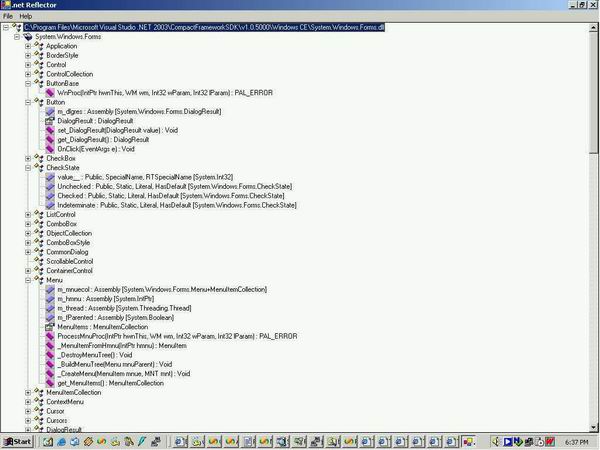
Introduction
Reflector acts as a class browser of an assembly whether it may be an executable or any DLL. Here in the Reflector, we can see how to rive an assembly using Reflection in .NET.
OverView
This code uses FieldInfo
to get the fields (what we call data members in C#) using GetFields(BindingFlags)
, PropertyInfo
to get the properties (to get or set the fields) using GetProperties(BindingFlags)
, MethodInfo
to the methods that work on the data using GetMethods(BindingFlags)
and ParameterInfo
to get the parameters that are passed using GetParameters()
.
CodeListing
To load an assembly:
assembly = Assembly.LoadFrom(this.assemblyName);
To Load all the types in an assembly:
public ArrayList LoadAllTypes()
{
AssemblyTypeDetails typeDetails = null;
try
{
Type []types = assembly.GetExportedTypes();
typeDetails = null;
typeList = new ArrayList();
foreach(Type type in types)
{
typeDetails = new AssemblyTypeDetails();
typeDetails.TypeFullName = type.FullName;
typeDetails.TypeName = type.Name;
typeList.Add(typeDetails);
}
}
catch(Exception ex)
{
throw new Exception(ex.StackTrace);
}
finally
{
typeDetails = null;
}
return typeList;
}
To Load Fields:
public ArrayList GetFields(AssemblyTypeDetails typeDetails)
{
ArrayList fields = null;
try
{
fields = new ArrayList();
Type type = assembly.GetType(typeDetails.TypeFullName, true, true);
if(type != null)
{
foreach(FieldInfo field in type.GetFields(BindingFlags.Public
| BindingFlags.Instance | BindingFlags.Static
|BindingFlags.NonPublic |BindingFlags.DeclaredOnly))
{
fields.Add(field.Name.ToString()
+" : "+field.Attributes.ToString()
+" ["+field.FieldType.FullName+"]");
}
}
}
catch(Exception ex)
{
throw new Exception(ex.Message);
}
return fields;
}
To Load Properties:
public ArrayList GetProperties(AssemblyTypeDetails typeDetails)
{
ArrayList props = null;
try
{
props = new ArrayList();
Type type = assembly.GetType(typeDetails.TypeFullName, true, true);
if(type != null)
{
foreach(PropertyInfo prop in type.GetProperties(BindingFlags.Public
| BindingFlags.Instance
| BindingFlags.Static
| BindingFlags.NonPublic
|BindingFlags.DeclaredOnly))
{
props.Add(prop.Name.ToString()
+" : "+prop.PropertyType.Name);
}
}
}
catch(Exception ex)
{
throw new Exception(ex.Message);
}
return props;
}
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.