Introduction
Below is a very simple example of how to consume and use jQuery in HTML pages. The example will show some features of jQuery, like jQuery selectors $()
and animation. And to tie everything together, we will create a simple image carousel. Our on screen image carousel will loop through a set of images for our users in a fadeout/fadein animation. A fiddle is created here.
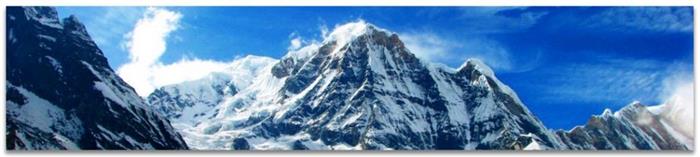
jQuery is a library built on top of JavaScript, to facilitate easy client side development. When I mean client side development – I mean development that produces code, that executes on the clients browser and the code is - JavaScript.
One very important feature of jQuery is that, in most cases it takes care of all the different browser anomalies and you don’t have to write browser specific code. But please note, when a browser doesn’t support certain HTML features, jQuery/JavaScript cannot help much.
Now To Our Code...
All the code is contained in an HTML file - Carousel.html. The jQuery library is stored in folder /Scripts and the images for our carousel are stored in the /images folder.
In our HTML file, the code is divided into three parts.
1. HTML markup
Most people can skip this part and jump to the JavaScript code, as most of us are familiar with HTML.
<body>
<div align="center" style="width: 100%; height: 100%;">
<div>
<div id="carousel_main">
<div id="carousel">
<img width="960" id="carousel_1" src="#" />
<img width="960" id="carousel_2" src="#" />
</div>
<div id="imageList" style="visibility: hidden;">
<ul>
<li>
<img src="images/image1.jpg" />
</li>
<li>
<img src="images/image2.jpg" />
</li>
<li>
<img src="images/image3.jpg" />
</li>
<li>
<img src="images/image4.jpg" />
</li>
<li>
<img src="images/image5.jpg" />
</li>
</ul>
</div>
</div>
</div>
</div>
</body>
As you can see above, the HTML <body>
tag has a number of <div>
s, a couple of <img>
s and a <ul>
with <li>
.
We have center aligned our outer most div
, to keep our content in the center and given it 100% width and height.
The div
with id=“carousel”
contains two image placeholders, carousel_1
and carousel_2
. We will change the source of the image placeholders in our jQuery code.
The div
containing the <ul>
(unsorted list) and <li>
(list item) is hidden (style="visibility: hidden"
) and its list items have some images loaded in them. The only function of this list is to maintain a list of images for our carousel.
2. JavaScript
<script src="Scripts/jquery-1.4.1.js" type="text/javascript"></script>
The above line in our HTML page loads the jQuery library and makes itself available on the page to be used by us.
First, let’s talk a bit about jQuery Selectors: the $()
function, which lets us find and select the element(s) in our markup using expressions. We will see some examples from our code below...
$("#imageList").find("li").first().addClass("selected");
In the above code, we have $("#imageList")
, which tells jQuery to go into the HTML markup and look for an HTML element with the id=imageList
. In the same line of code, we are asking jQuery to find and select the first li
element inside the element imageList
and add a CSS class “selected
” to the selected li
element.
Whats going on here? Well, jQuery can search for elements based on their ids, element type, an elements property or a CSS class applied to an element. Some examples below...

I think I must have covered all the selectors we have in the code. Now let's move to the implementation.
Logical Implementation
We have two images - carousel_1
and carousel_2
, at any time only one will be visible to the user. When it's time to change the image, the first image will fade out and vanish and the second image will fade in. The then invisible image will be loaded with the next image in the carousel, in preparation to be shown to the user.
Detailed Implementation
Our execution starts at $(document).ready()
. The $(document).ready()
is a jQuery event, which is executed after the DOM(Document Object Model) of our HTML page is fully loaded and ready. We write our code inside the ready event.
$("#imageList").find("li").first().addClass("selected");
$("#carousel img").first().attr("src",
$("#imageList li.selected").find("img").attr("src")).fadeIn($transition_time);
$("#carousel img:last").hide();
We start by giving the first image in the hidden <ul>
, a class “selected
”. We use this “selected
” tagged image in the <ul>
as a source to the first image in our carousel and hide the last image in our carousel. An interesting point worth noting here is, the class “selected
” does not exist, and has no properties, but it gets added to the element and is used as a reference point.
In our code, we are executing a method, setCarouselImage()
in a loop at a pre set interval - $time_between_slides = 3000;
. We are executing this method via a JavaScript method: setInterval(function{ }, interval);
The setInterval()
method executes the setCarouselImage()
method every 3 seconds till infinity of the browser session!
var $visibleCarousel = $("#carousel img:visible");
var $invisibleCarousel = $("#carousel img:hidden");
In the setCarouselImage()
method, we get references to the visible and invisible images in the carousel.
We then, set the next image in the ul
(unsorted list) to the invisible image in the carousel. We also switch the “selected
” class between the images.
Next is the interesting bit.
$visibleCarousel.animate({
opacity: "0"
}, $transition_time, function () {
$visibleCarousel.css("display", "none");
$visibleCarousel.css("opacity", "");
$invisibleCarousel.fadeIn($transition_time * 2);
});
The jQuery animate()
function, which executed on the visible image, slowly hides the visible image. Waits for the opacity to turn zero, and then shows - fadeIn()
the invisible image. It creates an interesting fadeout/fadein effect.
There is a local function getNextSwitch()
, which is used to get the next available image from our image list <ul>
.
3. Cascading Style Sheet (CSS)
<style type="text/css">
#carousel
{
-webkit-box-shadow: 0 5px 8px 1px Gray;
-moz-box-shadow: 0 5px 8px 1px Gray;
box-shadow: 0 5px 8px 1px Gray;
width: 960px;
height: 200px;
background: white;
position: relative;
overflow: hidden;
}
</style>
We have applied a small CSS on our div
– carousel. Here, we are setting the dimensions and other attributes of the div
. We are also giving a drop shadow to the div
so that our image carousel stands out.
As you can see, the CSS is applied to a – Mr. #carousel! Interestingly we have passed the same in the jQuery $()
function to select the carousel div
. That means jQuery uses the CSS selectors as expressions in the $()
function.
Just a small connection between CSS and jQuery I wanted you to see...
Detailed explanation on the drop shadow feature is not provided in this text, I will try to write a text on advanced CSS 3.0 features.