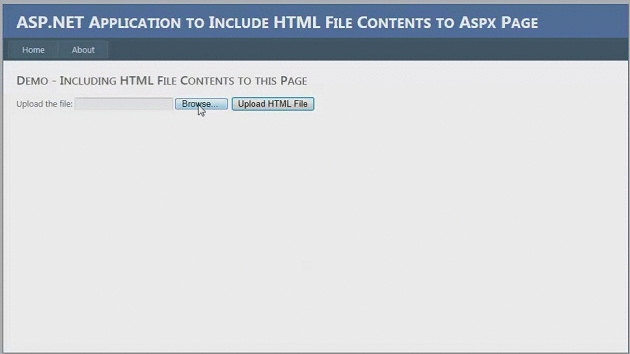
Introduction
This article explains the code to include the HTML file contents dynamically to the ASPX page. You can download the code and check how it is working.
Background
I always see this type of requirements asked by members in the Coding Forums. Finally I tried to achieve this. Once, the same thing came to one of my projects, so it also served the purpose. That's why, I am sharing with all of you. It may help you some day.
Using the code
Step-1: Add Required Controls
FileUpload
Control to select the HTML
File.
Upload the file:
<asp:FileUpload ID="fileHTMLToBeIncluded" runat="server" />
Button
to Upload the file onclick
.
<asp:Button ID="btnUploadFile" runat="server"
Text="Upload HTML File" OnClick="btnUploadFile_Click" />
Literal
Control to show the HTML
File contents.
<asp:Literal ID="ltHTMLBody" runat="server"></asp:Literal>
Label
to show any Errors/Exceptions.
<asp:Label ID="lblError" runat="server"></asp:Label>
Step-2: Button Upload Code
I will explain the concept. You can refer the whole code by downloading it.
Logic
- Upload and save the File.
- Open it using File.OpenText Method[^]. And then read to the end of File.
- Use Regex Class[^](
RegularExpression
) to get only the body part contents of HTML
File. - Assign this content to the
Literal
Control Text
Property.
Deeper into the Third Step (Regex)
Suppose the Hello World HTML file we want to include to ASPX page is something like below.
<html>
<head>
<title>
Demo:- Include HTML in Aspx Page
</title>
</head>
<body>
<div style="font-family: Verdana; font-size: 20px; color: green; font-weight: bold;">
<label>Hello World!!! From HTML Page.</label>
</div>
</body>
</html>
Now we just need to find the contents inside the body
tags (here the division
).
For that, we need to remove texts other than the division
.
<html>
<head>
<title>
Demo:- Include HTML in Aspx Page
</title>
</head>
<body>
<div style="font-family: Verdana; font-size: 20px; color: green; font-weight: bold;">
<label>Hello World!!! From HTML Page.</label>
</div>
</body>
</html>
To achieve this, we need two Regular Expressions
.
First One ( [\s\S]*<body[^<]*
) to match the string before the division
start.
Second One ( </body[\s\S]*
) to match the string after the division
end tag.
Let me explain one by one.
[\s\S]*<body[^<]*>
:-
This matches the whole data up to the end of body
start tag.
Here the first part [\s\S]*
will match each and every character before the body
start tag.
And the whole Regex
will match all the characters up to the end of body
start tag.
So, it will match (which is showing as strike through)...
<html>
<head>
<title>
Demo:- Include HTML in Aspx Page
</title>
</head>
<body>
<div style="font-family: Verdana; font-size: 20px; color: green; font-weight: bold;">
<label>Hello World!!! From HTML Page.</label>
</div>
</body>
</html>
As we get the matched string, we just need to make it blank by using <a href="http://msdn.microsoft.com/en-us/library/xwewhkd1.aspx">Regex.Replace Method (String, String)</a>[<a href="http://msdn.microsoft.com/en-us/library/xwewhkd1.aspx" target="_blank" title="New Window">^</a>]
.
start = new Regex(@"[\s\S]*<body[^<]*>", RegexOptions.IgnoreCase);
strHTML = start.Replace(strHTML, string.Empty);
Now after this coding line, we will have HTML
text (strHTML) as below.
<div style="font-family: Verdana; font-size: 20px; color: green; font-weight: bold;">
<label>Hello World!!! From HTML Page.</label>
</div>
</body>
</html>
</body[\s\S]*
:-
This Regex
will match every character starting from body
end tag till the end.
<div style="font-family: Verdana; font-size: 20px; color: green; font-weight: bold;">
<label>Hello World!!! From HTML Page.</label>
</body>
</html>
Now, we will follow the same procedure to replace the matched string with blank.
end = new Regex(@"</body[\s\S]*", RegexOptions.IgnoreCase);
strHTML = end.Replace(strHTML, string.Empty);
After this line, the HTML
text (strHTML) will contain the below text.
<div style="font-family: Verdana; font-size: 20px; color: green; font-weight: bold;">
<label>Hello World!!! From HTML Page.</label>
</div>
Last Step
Last step is to assign the above division
to the Literal
Control Text
.
ltHTMLBody.Text = strHTML;
History
- 03 July 2013 - First version submitted for approval.
- 29 Dec 2014 - Project Added for Download.