Introduction
One feature from legacy web services that we would miss in this modern http services days is WSDL. It is the interface to client that:
- Lists down all the HTTP operations that can be performed on a Service
- Describes input and output parameter data types to invoke the operations
Though, Rest API services are very efficient, it is not self described. Client applications that consume the rest service have no clue about input and output data format unless explicit documentation is provided.
Swagger
Swagger comes to the rescue here. It provides a nice UI listing all the http endpoints and with sample of input and output parameters.
Official site - swagger.io
Let's show you the steps.
- Create a Web API project in VS. Create a new controller named '
Customer
' and paste the below code.
public class CustomerController : ApiController
{
static List<Customer> Customers = new List<Customer>();
static int counter = 0;
public IEnumerable<Customer> Get()
{
return Customers;
}
public Customer Get(int id)
{
return Customers.Where(c => c.Id == id).FirstOrDefault();
}
public void Post([FromBody]string value)
{
Customer c = new Customer() { Id = ++counter, Name = value };
Customers.Add(c);
}
public void Put(int id, [FromBody]string value)
{
Customers.Where(c => c.Id == id).Select(c => { c.Name = value; return c; }).ToList();
}
public void Delete(int id)
{
Customers.Remove(Customers.Where(c => c.Id == id).FirstOrDefault());
}
}
- Install Swagger through Nuget package manager console:
PM> Install-Package Swashbuckle
FYI. This installation will create a file - SwaggerConfig under App_Start.
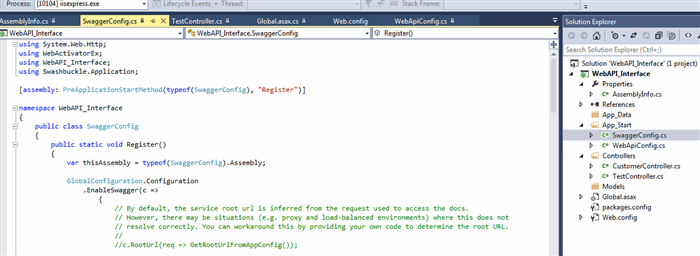
- Run the project and type swagger at end of the url in browser.
http:
You should be seeing this screen - Swagger
UI. Click on 'expand operations' to view all the http operations in a detailed way.

What this Swagger UI is Capable Of ?
- All type of Http requests -
GET
, POST
, PUT
and DELETE
can be triggered from this UI. Input proper data and click 'Try it now' button. Response will be shown right below. Something like Postman in Chrome, Fiddler. - Model Schema - Output model structure which supports both XML and JSON format.
- Response content type is editable.
- Response includes a lot of information like response data, code and headers.
- Shows cURL commands for the requests fired. cURL is a command line utility to create http requests using commands that support all features that can done through browser. First, cURL needs to be installed to use this.
What are you waiting for? Download the attached project and have fun!!
Yet another software developer who works on various Microsoft and Web technologies but curious to try something beyond an usual