Introduction
Version management of the assemblies and the version publish is a widespread need. Comparing them with various colleagues, I have found different approaches.
I believe that my experience and code collage will be useful to those who want a quick and easy solution to manage both versions that the Copyright field.
Using the Code
The update process of the courts is based on a small C # project that is responsible for updating the AssemblyInfo.cs file and the project file.
We analyze the first batch being run at each compilation:
Rem UpdateVersion.Bat
Rem Autore : Argo 69
Rem Data : 16/12/2012
Rem Scopo : Esegue l'aggiornamento della versione e della Maior Release
Rem ************************************************************
rem %1 Configurazione corrente
rem %2 Percorso e nome del file di versione
Rem ************************************************************
echo ==== Aggiornamento della versione
rem Controlla variabili di ambiente e quelle passate
if A%1 == A goto errorUsage
if A%2 == A goto errorUsage
if %1 == Debug goto Debug
if %1 == Alpha goto Alpha
if %1 == Beta goto Beta
if %1 == Candidate goto Candidate
if %1 == RTM goto RTM
if %1 == Release goto Release
goto OUT
:errorUsage
echo Errore in UpdateVersion.Bat
echo Modo d'uso: UpdateVersion.bat $(ConfigurationName) VersionFile
goto OUT
:Debug
attrib -r "%2"
s:\batch\Versioner.exe "%2" 1 %date:~6,4% 666 d %3
goto end
:Alpha
attrib -r %2
s:\batch\Versioner.exe %2 1 %date:~6,4% 111 d %3
goto end
:Beta
attrib -r %2
s:\batch\Versioner.exe %2 1 %date:~6,4% 222 d %3
goto end
:Candidate
attrib -r %2
s:\batch\Versioner.exe %2 1 %date:~6,4% 333 d %3
goto end
:RTM
attrib -r %2
s:\batch\Versioner.exe %2 1 %date:~6,4% 888 d %3
goto end
:Release
attrib -r %2
s:\batch\Versioner.exe %2 1 %date:~6,4% 0 d %3
goto end
:end
attrib +r %2
echo ==== Aggiornamento versione %1 terminata
:OUT
The Major
release set directly in the Batch in the example is set to 1.
The Minor
release calculated directly from Batch in the example is equal to the current year.
Build the release is set by Batch according to the configuration.
The revision is enhanced as follows:
- If you set the
d
value shall be the day number DateTime.Now.DayOfYear.ToString ()
- If a numerical value is set, it will be used like this.
Now we analyze Versioner
:
using System;
using System.IO;
using System.Text.RegularExpressions;
using System.Xml;
namespace ARG.Versioner
{
internal class Versioner
{
static string EOM = "Argo New Deal - Italy";
private static void Reversion(FileInfo fi, string Major,
string Minor, string Build, string Revision = "", string @ProjectPath="")
{
if (Revision.ToLower() == "d")
{
Revision = DateTime.Now.DayOfYear.ToString();
}
FileStream fileStream = null;
string[] array2;
char c = '"';
try
{
fileStream = fi.Open(FileMode.Open, FileAccess.Read, FileShare.None);
array2 = new StreamReader(fileStream).ReadToEnd().Split(new char[]
{
'\n'
}, StringSplitOptions.RemoveEmptyEntries);
}
catch (Exception innerException)
{
throw new Exception("AssemblyInfo.cs - Could not read file", innerException);
}
finally
{
if (fileStream != null)
{
fileStream.Close();
fileStream = null;
}
}
for (int i = 0; i < array2.Length; i++)
{
if (array2[i].IndexOf("[assembly: AssemblyCompany") != -1)
{
array2[i] = string.Concat(new object[]
{
"[assembly: AssemblyCompany(",
c,
EOM,
c,
")]"
});
}
if (array2[i].IndexOf("[assembly: AssemblyCopyrig") != -1)
{
array2[i] = string.Concat(new object[]
{
"[assembly: AssemblyCopyright(",
c,
" Copyright ",
DateTime.Now.Year.ToString(),
" "+ EOM + " All rights reserved ",
c,
")]"
});
}
if (array2[i].IndexOf("[assembly: AssemblyVersion") != -1)
{
array2[i] = string.Concat(new object[]
{
"[assembly: AssemblyVersion(",
c,
Major.ToString(),".",Minor.ToString(),".",
Build.ToString(),".",Revision.ToString(),c ,
")]"
});
}
if (array2[i].IndexOf("[assembly: AssemblyFileVer") != -1)
{
array2[i] = string.Concat(new object[]
{
"[assembly: AssemblyFileVersion(",
c,
Major.ToString(),".",Minor.ToString(),".",
Build.ToString(),".",Revision.ToString(),c ,
")]"
});
}
}
try
{
fileStream = fi.Open(FileMode.Create, FileAccess.Write, FileShare.None);
using (StreamWriter streamWriter = new StreamWriter(fileStream))
{
for (int j = 0; j < array2.Length; j++)
{
streamWriter.WriteLine(array2[j].Trim(new char[]
{
'\r'
}));
}
}
}
catch (Exception innerException2)
{
throw new Exception("AssemblyInfo.cs - Could not write file", innerException2);
}
finally
{
if (fileStream != null)
{
fileStream.Close();
fileStream = null;
}
}
UpdatePublish(@ProjectPath, Major, Minor, Build, Revision);
}
private static bool UpdatePublish(string ProjectPath,
string Major, string Minor, string Build, string Revision = "")
{
try
{
Microsoft.Build.Evaluation.Project p =
new Microsoft.Build.Evaluation.Project(ProjectPath);
Console.WriteLine("Old Publish Version: " +
p.GetPropertyValue("ApplicationVersion"));
p.SetProperty("ApplicationVersion",
Major+"."+Minor+"."+Build+"."+Revision);
p.Save();
Console.WriteLine("Project file updated "+ ProjectPath);
}
catch (Exception err)
{
throw new Exception("ProjectFile - File non trovato: " +
ProjectPath +" ||"+ err.Message + " ||");
}
return true;
}
private static string EomInfo()
{
FileStream fileStream = null;
string[] array2;
FileInfo fileInfo = new FileInfo(Environment.ExpandEnvironmentVariables("EomFileDef.Txt"));
if (!fileInfo.Exists)
{
throw new Exception("Eom File Def - Did not find file: " + fileInfo.FullName);
}
string EomInfo = "";
try
{
fileStream = fileInfo.Open(FileMode.Open, FileAccess.Read, FileShare.None);
array2 = new StreamReader(fileStream).ReadToEnd().Split(new char[]
{
'\n'
}, StringSplitOptions.RemoveEmptyEntries);
}
catch (Exception innerException)
{
throw new Exception("Eom File Def - Could not read file", innerException);
}
finally
{
if (fileStream != null)
{
fileStream.Close();
fileStream = null;
}
}
EomInfo = array2[0];
return EomInfo;
}
[STAThread]
private static void Main(string[] args)
{
string _EomInfo = EomInfo();
if (_EomInfo != "") EOM = _EomInfo;
try
{
if (args.Length > 0)
{
FileInfo fileInfo = new FileInfo(Environment.ExpandEnvironmentVariables(args[0]));
if (!fileInfo.Exists)
{
throw new Exception("AssemblyInfo.cs - Did not find file: " + fileInfo.FullName);
}
if (args.Length == 4)
{
Versioner.Reversion(fileInfo, args[1], args[2], args[3], "","");
}
if (args.Length == 5)
{
Versioner.Reversion(fileInfo, args[1], args[2], args[3], args[4],"");
}
if (args.Length ==6)
Versioner.Reversion(fileInfo, args[1], args[2], args[3], args[4], @args[5]);
}
else
{
Console.WriteLine("Syntax: Versioner assemblyinfo.cs,
Major,Minor,NumberOfBuild,ProjectPath ");
}
}
catch (Exception innerException)
{
while (innerException != null)
{
Console.Write(innerException.Message);
innerException = innerException.InnerException;
}
}
}
}
}
As we see, also search a file EomFileDef.Txt that will contain the business name to be used to Copyright & Company field.
How To Use It
Copy the Batch and the executable Versione.exe in a folder in the example S:\Batch.
- Add in all the projects in the Build Events - Prebuild the following
string
:
s:\Batch\UpdateVersion.bat $(ConfigurationName) "$(ProjectDir)Properties\AssemblyInfo.cs"
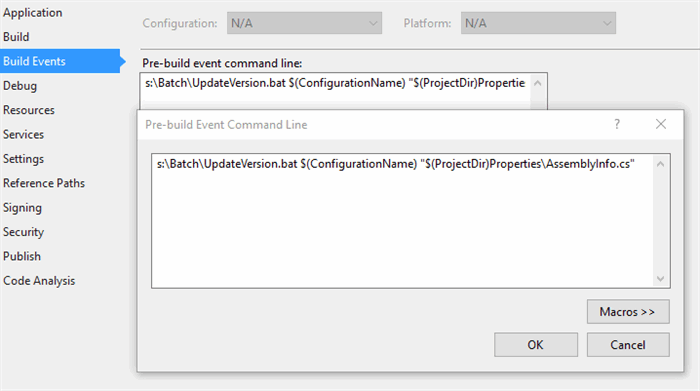
In the output folder of the project, add the file EomFileDef.Txt containing the name of our client or of our society.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.