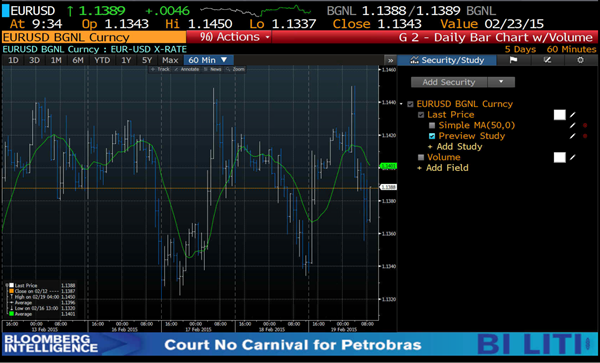
Introduction
Bloomberg L.P. provides financial software tools such as an analytics and equity trading platform, data services and news to financial companies and organizations through its Bloomberg Professional Service [1]. Bloomberg Terminal provides a number of API standards allowing extensions of its original capabilities. These include Bloomberg Study SDK and Bloomberg Application Portal SDK.
There is very little public information available on these SDK. I develop custom studies for Bloomberg Terminal using Bloomberg Study SDK for several years and would like to provide a quick start introduction to this technology as well as to give some deeper insights into little known caveats of development on Bloomberg platform.
Installation
To work with Bloomberg Study SDK you must have an active Bloomberg Professional Service account accessed via local Bloomberg installation - the shortcut on your desktop, not via Bloomberg Anywhere. You must have a valid license for using Bloomberg Study SDK on Bloomberg Professional Service before you can proceed.
As of the moment of writing this paper, Bloomberg Study SDK supports Microsoft Visual Studio 2013 and 2012. Express editions do not qualify.

To download and Install the SDK follow these steps:
- Make sure you have valid SDK license
- Type Bloomberg command: CSDK
- Use menu: SDK > Custom Study SDK
- Select latest SDK version from the table
- Click “Install” against the selected package in SDK table
- Wait for setup to complete
By default, the SDK is installed in the C:\Program Files\Bloomberg\SmartClient\Study SDK on your PC.

To verify installation open Visual Studio. You will see Bloomberg Study SDK toolbox on Visual Studio toolbar.
Toolbar offers the following features (ordered by button position on toolbar):
- Preview study in Bloomberg
- Debug study in Bloomberg
- Attach to study
- Detach from study
- Study settings
- Publish study
- Profile study
Next sections explain the use of these functions in more detail.
Create New Bloomberg Study ID
Creation of a new custom study is two-step process. Part of it is done in Bloomberg Terminal and another part in Visual Studio.
First, let us consider the first part: creation of new study ID on Bloomberg Terminal. To create new study follow these steps:
- Type “STDY” on Bloomberg command line to open Customs Study Manager
- Menu: “Create Study”

- Choose “CS.NET” study type

- Enter study name. For instance: “CodeProject Sample”
- Create a unique study identifier. For instance: “CODEPROJECT_SAMPLE.1”. There is a convention that study ID is named in capital letters with a version number appended at the end. Study ID should not contain whitespaces.

- The name and ID should be unique among all names and IDs of studies in Bloomberg network. If the name is not unique, error will appear. This might be confusing because you may not have access to third party studies causing conflicts and you may never be able watching them in the list of custom studies.
- Add Study Administrator
a. Administrative groups: “Add”
b. Add Role: Type your user name
c. Check box “Administrator” set checked

- Confirm all entered information.
- You will see confirmation on new study created

After completion of these steps you have new study ID on Bloomberg Terminal.
Create New Bloomberg Study Code
To create a new study project:
- Start Visual Studio.
- Select File > New > Project. The New Project dialog appears.
- Select Visual C# Project Types list.
- Select “Bloomberg Custom Study” under “My Templates” in the Templates pane.
- Enter a name for your project in the Name, e.g. "CodeProject", field and click OK.
- Add to or edit the generated skeleton code as needed.

Note: apart from “Bloomberg Custom Study” project type, one can create other types of Bloomberg projects listed in dialog. These projects differ with the scope of API available and original code template used on creation. We recommend using “Bloomberg Custom Study” as a default, unless you have reasons for other choice and know for sure what you are doing.
Coding Your First Study
Bloomberg Custom Study after completion provides you with ready to run sample study code.
Wizard creates a new study project according to selected project name, for instance: "CodeProject". Created project contains just one editable code file named equally to project name: “CodeProject.cs”. This file contains namespace and partial class also named equally to the project name:
namespace CodeProject
{
public partial class CodeProject
{
In addition, a supplementary designer file “CodeProject.Designer.cs” is created:
namespace CodeProject
{
[BlpCustomStudy(Id = "CodeProject", Description = "Custom Study")]
public partial class CodeProject : CustomStudy
{
public CodeProject (IDictionary input)
: base(input)
{
Initialize();
}
}
}
Normally, you should never edit this file. However, it can be useful in debugging and understanding the interaction of the study with Bloomberg Terminal.
Lets return to the main study file “CodeProject.cs”. The code of generated sample study is self-explanatory and already contains detailed comments on its structure and steps necessary to modify and run it.
The class contains definitions of parameters for the study and a template to create more parameters of different types:
#region Code Example
public StudyPriceProperty Price = new StudyPriceProperty(PriceType.Close);
public StudyIntegerProperty Period = new StudyIntegerProperty(11);
#endregion
Here is a default example of Initialize() method created by the wizard:
private void Initialize()
{
#region Code Example
Output.Add("AvgData", new TimeSeries());
StudyLine avgLine = new StudyLine("AvgData", Color.Lime);
ParentPanel.Visuals.Add("Average", avgLine);
#endregion
}
Here is a default example of Calculate() method created by the wizard:
public override void Calculate()
{
#region Code Example
TimeSeries input = Input[Price.Value];
Output.Update("AvgData", Indicator.SMAvg(input, Period.Value));
#endregion
}
You must just uncomment few lines, as illustrated above, and then you can run your first study and see it on Bloomberg chart.
If you omit all comments, the complete study code appears incredibly simple:
using System;
using System.Collections.Generic;
using System.Text;
using System.Drawing;
using Bloomberg.Study.API;
using Bloomberg.Study.CoreAPI;
using Bloomberg.Study.Util;
using Bloomberg.Study.TA;
using Bloomberg.Math;
namespace CodeProject
{
public partial class CodeProject
{
public StudyPriceProperty Price = new StudyPriceProperty(PriceType.Close);
public StudyIntegerProperty Period = new StudyIntegerProperty(11);
private void Initialize()
{
Output.Add("AvgData", new TimeSeries());
StudyLine avgLine = new StudyLine("AvgData", Color.Lime);
ParentPanel.Visuals.Add("Average", avgLine);
}
public override void Calculate()
{
TimeSeries input = Input[Price.Value];
Output.Update("AvgData", Indicator.SMAvg(input, Period.Value));
}
}
}
Now let us see how it looks like when run on Bloomberg Terminal.
Preview a Study on Bloomberg Terminal
To preview a Custom Study do the following steps:
- Compile your custom study.
- Click the Study Settings icon on the Study Tools toolbar.
- The Project Settings dialog opens.
- Select the study project from the Project list.
- Enter the single-security G chart # on which to preview the study.

- Click Save.
- Click the Preview Study in Bloomberg icon on the Study Tools toolbar.
- The G chart is launched in a Bloomberg panel with the study previewed.
- Right-click on the chart and select Preview Study > Settings to launch the Study
- Properties popup for the previewed study.
- Modify the settings of the previewed study.
- Select the Display tab to modify the visual properties of the previewed study.
- Click Update to refresh the chart with the new properties of the previewed study.
Publishing a Study
When publishing a new study you should us new custom study profile created above:
- Click the Publish Study icon on the Study Tools toolbar to launch the Publishing Wizard.
- Click Next to go to the Publish Study page of the wizard.
- Select the study project to publish from the Project list.

- Select the same study ID created in the previous section to use as the publishing target.
- Click Next to go to the Study Dependencies page of the wizard.

- Click Next to go to the Additional Resources page of the wizard.
- Click Next to go to the Version Summary page of the wizard.
- Enter a version Label for the custom study, if necessary.
- Enter Comments for the custom study, if necessary, and review the Summary.

- Click Next to publish the custom study.
- Click OK to close the publishing wizard. The study should appear on STDY<GO> in approximately 10 minutes.
Using a Published Custom Study
To use a published custom study, add it to a single-security G chart:
- Create a new single-security chart or open an existing one.
- Select Edit > Data Series & Studies to launch the Properties popup to the Data Series & Studies panel.
- Expand the User Defined Studies node on the left.

- Expand the My Studies node.
- Click the custom study’s name to add it to the right side of the panel.
- Enter 1<GO> or click Update to refresh the chart with the custom study.

To edit properties of a custom study on a G chart:
- Right-click on the chart and select Custom Study Name > Settings to launch the Study Properties popup.
- Modify the settings of the custom study.
- Select the Display tab to modify the visual properties of the custom study.
- Click Update to refresh the chart with the new properties of the custom study.
Publishing a New Version of an Existing Study
- In Visual Studio, open the custom study project to be updated.
- Modify the code.
- Preview the new version of the custom study, following the steps in “Previewing a Custom Study.”
- Click the Publish Study icon on the Study Tools toolbar to launch the Publishing Wizard.
- Click Next to go to the Publish Study page of the wizard.
- Select the study project to publish from the Project list.
- Select the same study ID used in the previous version as publishing target.
- Click Next to go to the Study Dependencies page of the wizard.
- Click Next to go to the Additional Resources page of the wizard.
- Click Next to go to the Version Summary page of the wizard.
- Enter a version Label for the new version of the custom study, if necessary.
- Enter Comments for the custom study, if necessary, and review the Summary.
- Click Next to publish the custom study.
- Click OK to close the publishing wizard. The new version will be available in approximately 10 minutes.
- Reopen a G chart containing this custom study, or add it to a new G chart by following the steps in “Using a Custom Study.”
Advanced Study Programming
This paper is getting too long already to cover all aspects of study programming in Bloomberg Terminal. In the next article we will cover advanced programming topics, such as:
- Detailed class structure of study SDK
- Advanced study calculation logic
- Study updates on real time charts
- Studies with multiple assemblies
- Partial trust environment
- Sharing study with your colleagues
Post your comments and ask for more topics to cover!
Disclaimer
The product names used in this document are for identification purposes only. All trademarks and registered trademarks are the property of their respective owners. The following trademarks may or may not be marked in this document:
Bloomberg Terminal is a trademark or registered trademark of Bloomberg L.P. in United States and/or other countries.
Other company, product, and service names may be trademarks, registered trademarks, or service marks of other owners.
References
http://www.bloomberg.com/
http://www.bloomberg.com/professional/
A proven experience in business process migration among all major platforms and environments. Reengineering, Analysis and Redocumentation with SAP® Solution Manager, Oracle® BPA Suite, Software AG (IDS Scheer) ARIS® Platform, Fujitsu Interstage BPM Suite (i-Flow), TIBCO® Business Studio, Casewise® Corporate Modeler Suite, IBM Rational Method Composer and WebSphere® Business Modeler, Metastorm® ProVision, Microsoft® SharePoint® and Visio®, UDDI Webservice Repositories, Sparx® Enterprise Architect, HP Universal CMDB with XML, XPDL, XMI, eEPC, BPMN, UML.