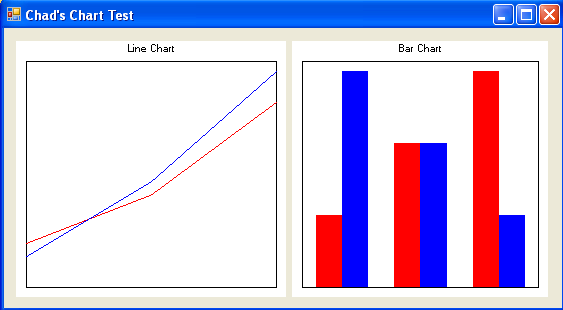
Introduction
I had some time this weekend, so I thought I’d try to throw together a charting package. The project was fun, but I did get a little carried away. The result is bar and line charting controls. The result contains both ASP.NET and Windows Forms based controls.
Using the code
The source code contains five projects, yes that is a lot. Two of the projects are test projects, ChadsCharts.WebTest
and ChadsCharts.Test
. Two projects are control wrappers, ChadsCharts.Web
and ChadsCharts.WinForm
. The last project is ChadsCharts.Imaging
that does the real heavy lifting. All coding was done in VS2005.
ChadsCharts.Imaging
Base rendering code. Generates the image for the chart.
ChadsCharts.Web
ASP.NET control wrappers for charts.
ChadsCharts.WinForm
WinForms control wrappers for charts.
WebTest
Web test application.
ChadsCharts.Test
Test application for ChadsCharts.WinForm
.
You can use the Imaging
classes directly to generate a chart, but you can also use controls in ChadsCharts.WinForm
and ChadsChart.Web
. To use the controls, you only really need to initialize a few members such as title and data. Each chart takes a DataSeriesCollection
as input, and then automatically generates the chart associated with the data. The sample code below shows how to initialize a bar chart:
Private Sub InitBarChart()
Dim series1 As New ChadsCharts.Imaging.DataSeries("Series1")
series1.Color = Color.Red
series1.Add(10)
series1.Add(20)
series1.Add(30)
BarChart1.Data.Add(series1)
Dim series2 As New ChadsCharts.Imaging.DataSeries("Sales2")
series2.Color = Color.Blue
series2.Add(30)
series2.Add(20)
series2.Add(10)
BarChart1.Data.Add(series2)
End Sub
The code above creates two data series. Each series is given a name in the constructor (currently not used). The color of the series is specified with the Color
member. Last items (all double
s) are added to the series through the Add
method. Below is another example that initializes the line chart control:
Private Sub InitLineChart()
Dim series1 As New ChadsCharts.Imaging.DataSeries("Series1")
series1.Color = Color.Red
series1.Add(10)
series1.Add(21)
series1.Add(42)
LineChart1.Data.Add(series1)
Dim series2 As New ChadsCharts.Imaging.DataSeries("Sales2")
series2.Color = Color.Blue
series2.Add(7)
series2.Add(24)
series2.Add(49)
LineChart1.Data.Add(series2)
End Sub
The imaging classes can be used without the chart controls. For example, to create a bar chart image, just use ChadsChart.Imaging.BarChartImage
. The code below shows how to use the BarChartImage
class:
Private Sub GenerateImage_NoControl()
Dim barChartImage As New ChadsCharts.Imaging.BarChartImage()
barChartImage.Title = "A bar chart"
barChartImage.Size = New Size(256, 256)
Dim series1 As New ChadsCharts.Imaging.DataSeries("Series1")
series1.Color = Color.Red
series1.Add(10)
series1.Add(20)
series1.Add(30)
barChartImage.Data.Add(series1)
Dim series2 As New ChadsCharts.Imaging.DataSeries("Sales2")
series2.Color = Color.Blue
series2.Add(30)
series2.Add(20)
series2.Add(10)
barChartImage.Data.Add(series2)
barChartImage.ToImageFile("a_bar_chart.png", _
System.Drawing.Imaging.ImageFormat.Png)
End Sub
Conclusion
I wouldn't recommend using this solution in anything that matters. As I said before, it was pretty much thrown together, but it was fun to do. This solution could be used as a jumping off point for other projects.
I am a software developer in Lincoln, NE. I do most of my work in VB.NET, but still dabble in C++.
I have a B.S. and M.S. from the University of Nebraska – Lincoln.