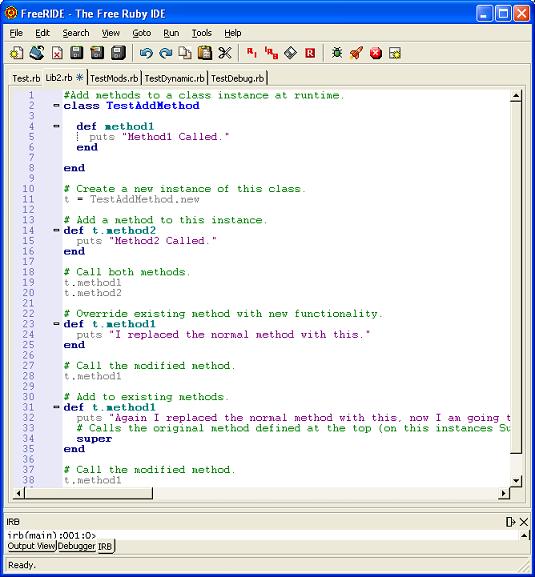
Introduction
This is a very brief article about one great feature of the Ruby language - dynamically adding methods to classes at runtime.
Basically I have been learning the Ruby language and thought I would share few snippets with people in small articles, so if you are not familiar with Ruby take a quick look and see what you think - you never know it might spark your interest!
Adding methods to class instances
One great feature of Ruby is that it allows you to add methods to individual class instances on the fly. The code is pretty self explanatory so I won't go into any details.
Update - 15 Sep 2006
- In response to some comments regarding how you use reflection etc in ruby I have added a new block of code showing how to reflect against a class, call methods dynamically and a simple benchmark showing the timings for each method.
## ---- Add methods to a class instance at runtime. ---- ##
# Create a very simple class.
<CODE __designer:dtid="281474976710672">class TestAddMethod
def method1
puts "Method1 Called."
end
end
</CODE>
# Create a new instance of this class.
<CODE __designer:dtid="281474976710672">t = TestAddMethod.new
</CODE>
# Add a method to this instance.
<CODE __designer:dtid="281474976710673">def t.method2
puts "Method2 Called."
end</CODE>
# Call both methods.
<CODE __designer:dtid="281474976710674">t.method1
t.method2
</CODE>
# Output:
# Method1 Called.
# Method2 Called.
# Override existing method with new functionality.
<CODE __designer:dtid="281474976710675">def t.method1
puts "I replaced the normal method with this."
end</CODE>
# Call the modified method.
<CODE __designer:dtid="281474976710676">t.method1</CODE>
# Output:
# I replaced the normal method with this.
# Add to existing methods.
<CODE __designer:dtid="281474976710677">def t.method1
puts "Again I replaced the normal method with this, now I am going to call the method on the original class: "
# Calls the original method defined at the top (on this instances SuperClass).
super
end</CODE>
# Call the modified method.
<CODE __designer:dtid="281474976710678">t.method1</CODE>
# Output:
# Again I replaced the normal method with this, now I am going to call the method on the original class:
# Method1 Called.
------- Section below added in response to some questions/comments from Baj22:
<CODE __designer:dtid="281474976710677">require "benchmark"
include Benchmark</CODE>
# Define a simple class.
<CODE __designer:dtid="281474976710677">class TestClass
def method1
puts "Called!"
end
def method2
puts "Called!"
end
def method3
puts "Called!"
end
def method4
end
end </CODE>
# Define a simple class.
<CODE __designer:dtid="281474976710677">class TestClass2 < TestClass
def method4
puts "Called!"
end
end </CODE>
# List the methods supported.
<CODE __designer:dtid="281474976710677">puts "TestClass supports #{TestClass.methods.length} method(s)" # Result: TestClass supports 76 method(s)
TestClass.methods.each do |method|
puts "\t #{method}"
end</CODE>
# Result:
# to_a
# respond_to?
# display
# etc, etc
# List the methods supported.
<CODE __designer:dtid="281474976710677">t = TestClass.new()
puts "T Instance supports #{t.methods.length} method(s)" #' Result: T Instance supports 46 method(s)
t.methods.each do |method|
puts "\t #{method}"
end</CODE>
# Result:
# to_a
# respond_to?
# display
# etc, etc
# Check if a class supports a method by name.
<CODE __designer:dtid="281474976710677">puts "r responds to 'Method1' = #{t.respond_to?("method1")}" # Result: true.
puts "r responds to 'UNKNOWN' = #{t.respond_to?("UNKNOWN")}" # Result: false.</CODE>
# Walk the inheritance tree.
<CODE __designer:dtid="281474976710677">cls = TestClass2
begin
print cls
cls = cls.superclass
print " < " if cls
end while cls</CODE> # Result: TestClass2 < TestClass < Object
# Call a method dynimically.
<CODE __designer:dtid="281474976710677">t.send(:method1)</CODE> # Result: Called!
<CODE __designer:dtid="281474976710677">method1 = t.method(:method1)
method1.call # Result: Called!
method4 = t.method(:method4)</CODE>
# Test dynamic calls.
<CODE __designer:dtid="281474976710677">n = 100000
bm(12) {|x|
x.report("Method.Call") { n.times { method4.call } }
x.report("Instance.send") { n.times { t.send(:method4) } }
x.report("Instance.Method") { n.times { t.method4} }</CODE>
}
#Result
# user system total real
#Method.Call 0.040000 0.000000 0.040000 ( 0.060000)
#Instance.send 0.080000 0.000000 0.080000 ( 0.090000)
#Instance.Method 0.050000 0.000000 0.050000 ( 0.051000)
More Info
There are other ways to add methods to classes - one is to use a mix-in - where you essentially include all of the methods from a module in a class definition. This allows you to mimic some of the multiple inheritance functionality seen in C++.
Anyway - thanks for your time. Any comments/questions are welcome. As I play with Ruby more I will try to post more articles on things that I find interesting.
History
Version 1.1
D Leverett is a software developer who has worked with a variaty of technologies over the years.
I enjoy learning new languages/skills and I am currently working my way through Ruby/Rails.
I am based in the UK and currently work for a recruitment CRM vendor.
MCSD(.NET), MCAD(.NET), MCTS(SQL 2005), MCSD(VB6/COM).