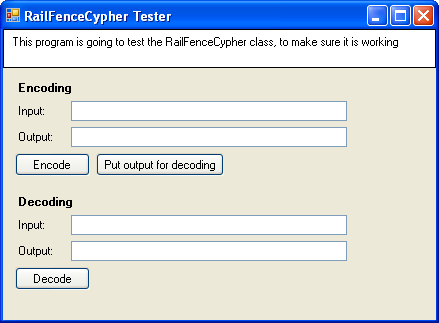
Introduction
The rail fence cipher is where you have some text like joejoe and you split it on two lines like:
j e o
o j e
Now you combine the two lines and get: jeooje
Encoding
At school, I have been taught how to encode stuff into the rail fence cipher. Here's how:
public static string Encode(string input)
{
char[] inputc = input.ToCharArray();
string pasA = "";
string pasB = "";
bool x = true;
foreach(char ch in inputc)
{
if(x == true)
{
pasA += ch;
x = false;
}
else
{
x = true;
pasB += ch;
}
}
return pasA + pasB;
}
Decoding
This is how to decode the stuff:
public static string DeCode(string input)
{
int lnl = (int)Math.Round((decimal)input.Length / 2);
char[] ln1, ln2;
ln1 = input.Substring(0, lnl).ToCharArray();
ln2 = input.Substring(lnl).ToCharArray();
#if DEBUG
Debug.WriteLine("Line 1: " + input.Substring(0, lnl));
Debug.WriteLine("Line 2: " + input.Substring(lnl, input.Length - lnl));
#endif
bool top = true;
string output = "";
int x, topx, botx;
x = 1; topx = 0; botx = 0;
while( x != input.Length + 1)
{
if(top == true)
{
if(topx > ln1.Length - 1)
{
return output;
}
output += ln1[topx]; topx += 1; top = false;
#if DEBUG
Debug.WriteLine("topx: " + topx + " ln1 length: " + ln1.Length);
#endif
}
else
{
output += ln2[botx]; botx += 1; top = true;
}
x++;
}
return output;
}
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.