Introduction
The Presentation Model pattern, known as a variation of the Model-View-Controller (MVC) design pattern, usually is a self-contained class that contains the data and event publications of the user interface, but without knowing what kind of user interface it will be.
The Presentation Model has key features like:
- State is in the presentation model
- Logic is in the presentation model
- View observes the model and updates accordingly
- The view “knows” about the presentation model
- The presentation model does not “know” about the view
In my previous article, I described using the Presentation Model pattern in an ASP.NET website, Windows Forms application, and a WPF application. They are very different technologies, but by using the Presentation Model pattern, it is possible to create a presentation model class that is reused among those different applications.
If you haven’t seen how the Presentation Model works in an ASP.NET website, Windows Forms application, and a WPF application, please read the article titled Presentation Model in Action.
In this article, I am going to reuse the presentation model class from the previous article into SharePoint.
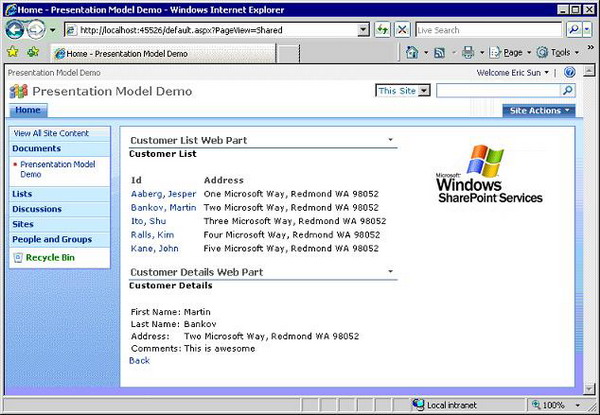
SharePoint as an Application Platform
SharePoint is a great platform to build business applications.
Having said that, I want to make clear that Windows SharePoint Services (WSS), SharePoint Portal Server (SPS), and Office SharePoint Server (MOSS) are designed to serve different levels of business requirements. SPS or MOSS might not meet your requirement, but WSS might. So, evaluate them one by one to see which one fits.
Here, we will do some work on Windows SharePoint Services 3.0. I like WSS 3.0 because it provides a set of core application basic services and infrastructure, all for free. When building applications on top of WSS 3.0, it is just like what Bill Carson said in his blog. It likes to build an 80-floor skyscraper, you start at the 60th floor, because the first 59 are literally out of the box.
To make the customer management ASP.NET website from the previous article in WSS 3.0, it requires only a few simple steps.
- Setup the SharePoint site to enable session and .NET 3.5 (optional)
- Copy the App_Code and bin folders and the user controls from the ASP.NET website to the SharePoint site
- Create WSS feature that has Web Parts made out of user controls
- Use the Web Parts in SharePoint pages
Configure the WSS 3.0 Site
To start with, I have created a new web application on port 45526 and a blank site. (If you want to learn more about creating a WSS site, please check out this link.)
Enable Session
The presentation model object’s lifecycle is the session scope (reasons explained in previous article), but WSS 3.0 by default disables the session. To enable the session, we can edit the web.config and un-comment this line:
<add name="Session" type="System.Web.SessionState.SessionStateModule" />
Then, find and set <pages> enableSessionState
attribute to be true.
Enable .NET 3.5
By default, the WSS 3.0 out of the box settings is to use .NET 2.0. The presentation model itself does not require .NET 3.5, but it is required because I use the .NET 3.5 features such as Automatic Properties and LINQ to entities in the Demo.DataModel library.
namespace Demo.DataModel
{
public class CustomerService : ICustomerService
{
#region ICustomerService Members
public IEnumerable<customer> GetAllCustomers()
{
return new List<customer>
{
new Customer{
FirstName = "Jesper",
LastName = "Aaberg",
Address = "One Microsoft Way, Redmond WA 98052",
Comments = "CAB Rocks!",
},
new Customer{
FirstName = "Martin",
LastName = "Bankov",
Address = "Two Microsoft Way, Redmond WA 98052",
Comments = "This is awesome",
},
new Customer{
FirstName = "Shu",
LastName = "Ito",
Address = "Three Microsoft Way, Redmond WA 98052",
Comments = "N/A",
},
new Customer{
FirstName = "Kim",
LastName = "Ralls",
Address = "Four Microsoft Way, Redmond WA 98052",
Comments = "N/A",
},
new Customer{
FirstName = "John",
LastName = "Kane",
Address = "Five Microsoft Way, Redmond WA 98052",
Comments = "N/A",
},
};
}
public IEnumerable<customer> GetSearchCustomers(string name)
{
return from customer in GetAllCustomers()
where customer.FirstName.Contains(name) ||
customer.LastName.Contains(name)
select customer;
}
#endregion
}
}
To turn on .NET 3.5, in web.config, first add 3.5 assemblies:
<assemblies>
<add assembly="Microsoft.SharePoint, Version=12.0.0.0,
Culture=neutral, PublicKeyToken=71e9bce111e9429c" />
<add assembly="System.Core, Version=3.5.0.0,
Culture=neutral, PublicKeyToken=B77A5C561934E089" />
<add assembly="System.Xml.Linq, Version=3.5.0.0,
Culture=neutral, PublicKeyToken=B77A5C561934E089" />
</assemblies>
Then, instruct it to use .NET 3.5 C# compile by adding the following session before </configuration>
:
<system.codedom>
<compilers>
<compiler language="c#;cs;csharp" extension=".cs" warningLevel="4"
type="Microsoft.CSharp.CSharpCodeProvider, System, Version=2.0.0.0,
Culture=neutral, PublicKeyToken=b77a5c561934e089">
<providerOption name="CompilerVersion" value="v3.5" />
<providerOption name="WarnAsError" value="false" />
</compiler>
</compilers>
</system.codedom>
Now, the WSS site is session enabled and .NET 3.5 enabled. Next is to copy the files to the WSS site.
Copy Files to WSS 3.0 Site

Copy the App_Code folder that has the CustomerPresentationModel
class, and the bin folder that has the Demo.DataModel.dll and Demo.PresentationModel.dll and two user controls, CustomerList.ascx and CustomerEdit.ascx into the WSS site’s root folder. All the files and DLLs are from the previous article, no extra code needed.
While in a regular ASP.NET website the building blocks are user controls, in WSS 3.0, Web Parts are mainly the building blocks. It could be a frustrating and scary job to build SharePoint Web Parts before WSS 3.0 because of two big barriers:
- Need a reference to
Microsoft.SharePoint.WebPartPages.WebPart
- Need to programmatically create the entire user interface of your control with no help from the designer
Once again, thanks Microsoft has made WSS 3.0 on top of the ASP.NET 2.0 API, these are no longer issues. ASP.NET 2.0 Web Parts can be used in WSS 3.0 directly. And, ASP.NET 2.0 Web Parts allow user controls to be loaded directly in a Web Part, with no modifications. (See this on MSDN.)
Next, we will create a WSS feature project and two Web Parts to load a customer list and a customer details user control.
Create WSS Feature and Web Parts
In WSS, custom functionalities are grouped into features. Developers create and package business process functions into features. Administrators can install them into SharePoint sites for users to activate and deactivate according to their needs.
Here, I have created a feature project using the STSDEV tool.

This article is not going to describe how to create a WSS feature and Web Parts. Please refer to other sources for feature definition, development, and deployment information. I highly recommend you visit STSDEV's screencasts to learn how to use this great tool.
Here, I just want to show how easy it is to make a Web Part out of the user control. Listed below is the code of CustomerEditWebPart
which loads the CustomerEdit.ascx user control:
public class CustomEditWebPart : System.Web.UI.WebControls.WebParts.WebPart
{
protected override void CreateChildControls()
{
Control control = Page.LoadControl("~/CustomerEdit.ascx");
control.ID = "CustomerEdit";
Controls.Add(control);
}
}
That's it. As simple as three lines, the customer details user control is a Web Part now.
Build and deploy the project. On the WSS site settings screen, the feature is installed and ready to be activated for use.

The customer list and customer details Web Parts are shown in the Web Part gallery once the feature is activated.

The next screenshot shows the home page in edit mode when adding the Web Parts to the home page.

Another screenshot shows that the demo Web Parts are used in a Web Part page within the document library.

It is also possible to create an ASPX page using SharePoint Designer 2007 and add the user controls to that page. But here, we will continue on discussing the Presentation Model pattern.
Back to the Presentation Model Pattern
Within the Presentation Model pattern, the presentation model class is nothing but a plain .NET class. This makes it possible to be widely used in ASP.NET websites, Windows Forms, WPF, and SharePoint.
public abstract class PresentationModel<t> : INotifyPropertyChanged
{
#region INotifyPropertyChanged Members
public event PropertyChangedEventHandler PropertyChanged;
protected void NotifyPropertyChanged(string propertyName)
{
if (PropertyChanged != null)
{
PropertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
#endregion
protected int itemCount;
public int ItemCount
{
get { return itemCount; }
set { itemCount = value; NotifyPropertyChanged("ItemCount"); }
}
protected IEnumerable<t> items;
public virtual IEnumerable<t> Items
{
get { return GetItems(); }
set { items = value; NotifyPropertyChanged("Items"); }
}
protected abstract IEnumerable<t> GetItems();
private T selectedItem;
public virtual T SelectedItem
{
get { return selectedItem; }
set { selectedItem = value; NotifyPropertyChanged("SelectedItem"); }
}
protected void Reset()
{
Items = null;
SelectedItem = default(T);
}
}
The presentation model class holds the data used by the views and sends events when the data changes. Events are broadcasted. There can be many views and classes observing the events.
A presentation model class does not know anything about the view, whether views are ASP.NET user controls, Windows Forms user controls, WPF user controls, SharePoint Web Parts, or any other classes. There are no limitations on specific application platforms.
Views have references to the presentation model class. Views pull data from the presentation model class and listen to the events. Views decide if the data changed events are relevant that need actions.
The presentation model class and views are loosely coupled through data and events.
Compared to some other development platforms (Java, PHP …), .NET has huge advantages in areas of data binding and event handling. To use the Presentation Model on other platforms, it may require some work in synchronizing the state between the view and the presentation model and in sending messages between views and presentation model. But, .NET has delegates, anonymous methods, and two-way data binding. We can and should take the advantages of these advanced technologies to unleash the power of the Presentation Model pattern.
Conclusion
This article demonstrated using the Presentation Model in a SharePoint site. The code is copied from the previous ASP.NET website without changes. Once again, it proved the Presentation Model as a powerful and useful pattern.
History
- Feb 3, 2008: First version.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.