Introduction
I was looking for an encryption method for my project, and I came across the TripleDES algorithm. But, later I found it annoying to declare and initialize the TripleDES
class again and again. So, I created a simple and basic class which solves the purpose.
Background
The idea behind the class was to make it simple to encrypt and decrypt data that I want to store in a database, like the username, password, and other stuff which are sensitive to be exposed. The class that I created is simple to use, with just the methods for a basic implementation. Let's see the class diagram:
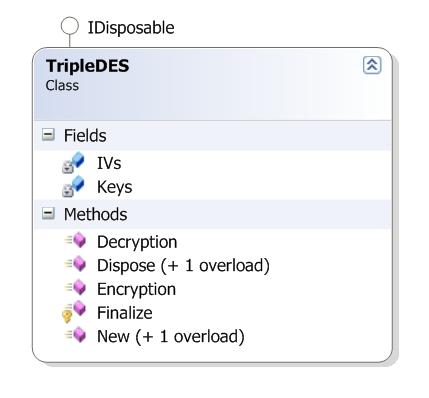
Using the code
To implement the class, you need to initialize the class. During the initialization of the class, the new method has been overloaded so that a user can define his own key and Vector (IV) for the symmetric algorithm. The default value for the key and the vector is as follows:
Dim Newkey() As Byte = {7, 99, 3, 44, 52, 76, 79, 84, 63, 63, 95, _
12, 10, 14, 15, 16, 64, 99, 38, 77, 68, 2, 3, 74}
Dim Newiv() As Byte = {75, 220, 255, 151, 65, 212, 209, 162}
Here is how the new class has been defined:
Public Sub New()
Dim Newkey() As Byte = {7, 99, 3, 44, 52, 76, 79, 84, 63, 63, 95, _
12, 10, 14, 15, 16, 64, 99, 38, 77, 68, 2, 3, 74}
Dim Newiv() As Byte = {75, 220, 255, 151, 65, 212, 209, 162}
Keys = Newkey
IVs = Newiv
End Sub
Public Sub New(ByVal Key() As Byte, ByVal IV() As Byte)
Keys = Key
IVs = IV
End Sub
To encrypt plain text
To encrypt text, you need to create an instance of a class and call the the Encryption
function. The return type of this function is an array of Byte
s. Here is how it is done:
Private NewTripleDES as new TripleDES()
Private EncryptedBytes() as Byte
EncrpytedBytes() = NewTripleDES.Encryption("PlainText")
Now, let's see the Encryption
function:
Public Function Encryption(ByVal plainText As String) As Byte()
Dim utf8encoder As UTF8Encoding = New UTF8Encoding()
Dim inputInBytes() As Byte = utf8encoder.GetBytes(plainText)
Dim tdesProvider As TripleDESCryptoServiceProvider = _
New TripleDESCryptoServiceProvider()
Dim cryptoTransform As ICryptoTransform = tdesProvider.CreateEncryptor(Me.Keys, Me.IVs)
Dim encryptedStream As MemoryStream = New MemoryStream()
Dim cryptStream As CryptoStream = New CryptoStream(encryptedStream, _
cryptoTransform, CryptoStreamMode.Write)
cryptStream.Write(inputInBytes, 0, inputInBytes.Length)
cryptStream.FlushFinalBlock()
encryptedStream.Position = 0
Dim result(encryptedStream.Length - 1) As Byte
encryptedStream.Read(result, 0, encryptedStream.Length)
cryptStream.Close()
Return result
End Function
To decrypt the encrypted data
To decrypt the encrypted data, you need to call the decryption function. The return type of this function is String
. Here is how it is done:
Private DecrytedText as String
DecrytedText = NewTripleDES.Decryption(EncryptedBytes())
Now, let's see the Decryption
function:
Public Function Decryption(ByVal cipherBytes() As Byte) As String
Dim utf8encoder As UTF8Encoding = New UTF8Encoding()
Dim tdesProvider As TripleDESCryptoServiceProvider = _
New TripleDESCryptoServiceProvider()
Dim cryptoTransform As ICryptoTransform = _
tdesProvider.CreateDecryptor(Me.Keys, Me.IVs)
Dim decryptedStream As MemoryStream = New MemoryStream()
Dim cryptStream As CryptoStream = New CryptoStream(decryptedStream, _
cryptoTransform, CryptoStreamMode.Write)
cryptStream.Write(cipherBytes, 0, cipherBytes.Length)
cryptStream.FlushFinalBlock()
decryptedStream.Position = 0
Dim result(decryptedStream.Length - 1) As Byte
decryptedStream.Read(result, 0, decryptedStream.Length)
cryptStream.Close()
Dim myutf As UTF8Encoding = New UTF8Encoding()
Return myutf.GetString(result)
End Function
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.