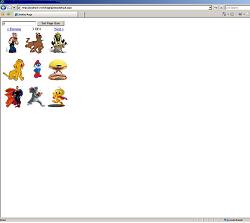
Introduction
I have created a ControlPaging
class to implement paging on the DataRepeater
, DataList
, and even on the DataGridView
. The primary purpose of this tutorial is to facilitate the developer to implement paging in his/her controls without any difficulty by calling a few properties. A Utility assembly (Utility.dll) has been used for this purpose. The source files contains the source code of the assembly as well. You can modify this code in order to make it better. Please do point out any weakness or any areas where it can be made better.
Before we begin, let me tell explain the flow for implementing paging using the ControlPaging
class. Here are the steps:
- Include the ControlPaging assembly in your application (in the Bin folder)
- Add the namespace
SyedFasih
above the code file (using SyedFasih;
) - Create an instance of the
ControlPaging
class (ControlPaging objPaging = new ControlPAging();
) - Set the
PageIndex
to the first page (objPaging.CurrentPageIndex = 0
) - Set the number of records you want to be displayed per page (
objPaging.PageSize = 10
) - Set the DataSource of the
ControlPaging
instance (objPaging.DataSource=<DataTable>
)
Now your paged data source is ready; you just have to set it as a data source of your control like:
MyDataListControl.DataSource = objPaging;
MyDataListControl.DataBind();
Using the code
These are the basic steps for implementing paging on your control. Explanation of the code is included inline:
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
using System.Data.OleDb;
using SyedFasih;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
DataTable dt = new DataTable();
if (!IsPostBack)
{
Connection.GetDatabseFile =
Request.PhysicalApplicationPath.ToString()+@"\DB\Cartoon.mdb";
OleDbConnection con = Connection.CreateConnection;
OleDbCommand cmd =
new OleDbCommand("SELECT * FROM CartoonCharacters", con);
OleDbDataAdapter da = new OleDbDataAdapter(cmd);
da.Fill(dt);
ControlPaging cpage = new ControlPaging();
try
{
cpage.CurrentPageIndex = 0;
cpage.PageSize = 6;
cpage.DataSource = dt;
Session["DT"] = dt;
Session["CPAGE"] = cpage;
BindList(cpage, dt);
}
catch (Exception)
{
}
finally
{
cpage = null;
}
}
}
void BindList(ControlPaging cpage, DataTable dt)
{
try
{
DLstCartoon.DataSource = cpage.DoPaging;
DLstCartoon.DataBind();
lnkPrev.Enabled = !cpage.IsFirstPage;
lnkNext.Enabled = !cpage.IsLastPage;
lblPageStatus.Text = NavigationIndicator();
}
catch (Exception)
{
}
finally
{
cpage = null;
}
}
string NavigationIndicator()
{
string str = string.Empty;
str = ((Convert.ToString((((
ControlPaging)Session["CPAGE"]).CurrentPageIndex + 1)) + " Of " + ((
ControlPaging)Session["cpage"]).PageCount.ToString()));
return str;
}
protected void Button1_Click(object sender, EventArgs e)
{
ControlPaging cpage = (ControlPaging)Session["CPAGE"];
cpage.CurrentPageIndex = 0;
Session["CPAGE"] = cpage;
try
{
cpage.PageSize = int.Parse(txtPageSize.Text);
BindList(cpage, ((DataTable)Session["DT"]));
}
catch (Exception)
{
Response.Write("Please enter an integer in the text box");
BindList(((ControlPaging)Session["CPAGE"]),
((DataTable)Session["DT"]));
}
finally
{
cpage = null;
}
}
protected void lnkNext_Click(object sender, EventArgs e)
{
((ControlPaging)Session["cpage"]).CurrentPageIndex += 1;
BindList(((ControlPaging)Session["cpage"]),
((DataTable)Session["DT"]));
}
protected void lnkPrev_Click(object sender, EventArgs e)
{
((ControlPaging)Session["cpage"]).CurrentPageIndex -= 1;
BindList(((ControlPaging)Session["cpage"]),
((DataTable)Session["DT"]));
}
}
ControlPaging class (Utility.dll)
The ControlPaging
class has the following properties:
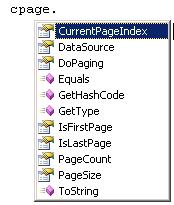
The ControlPaging
class uses PagedDataSource
to implement paging. PagedDatasource
has been wrapped around the ControlPaging
class to ease the paging process, so the user just need to call the properties to use the class.
using System;
using System.Collections.Generic;
using System.Text;
using System.Web;
using System.Web.UI.WebControls;
using System.Data;
namespace SyedFasih
{
public class ControlPaging
{
PagedDataSource oPage;
static int _CurrentPageIndex = 0;
static int _PageSize = 10;
DataTable dt;
public ControlPaging()
{
oPage = new PagedDataSource();
}
public int CurrentPageIndex
{
get
{
return _CurrentPageIndex;
}
set
{
_CurrentPageIndex = value;
}
}
public int PageSize
{
get
{
return _PageSize;
}
set
{
_PageSize = value;
}
}
public DataTable DataSource
{
set
{
dt = new DataTable();
dt = value;
}
get
{
return dt;
}
}
public bool IsLastPage
{
get
{
return oPage.IsLastPage;
}
}
public bool IsFirstPage
{
get
{
return oPage.IsFirstPage;
}
}
public int PageCount
{
get
{
return oPage.PageCount;
}
}
public PagedDataSource DoPaging
{
get
{
oPage.AllowPaging = true;
oPage.PageSize = PageSize;
oPage.CurrentPageIndex = CurrentPageIndex;
oPage.DataSource = DataSource.DefaultView;
return oPage;
}
}
}
}