Introduction
In this tutorial, we are going to create a simple dialog based application. We will use Button, Edit Box, Combo Box, List Box and Static text controls to develop our project. We will be going through the process of adding these controls into their dialog box, using the ClassWizard
to link these controls to variables, and handle messages sent by the controls.
I am going to assume you have good knowledge in C++ and you are an absolute beginner to Visual C++. The first thing you need to do is load up your Visual C++ 6 software and create a new project.
Creating a New Project
To create a new project, start by clicking on File in the menu of the Visual C++ 6 window, then on New.
The view should look like the image below:
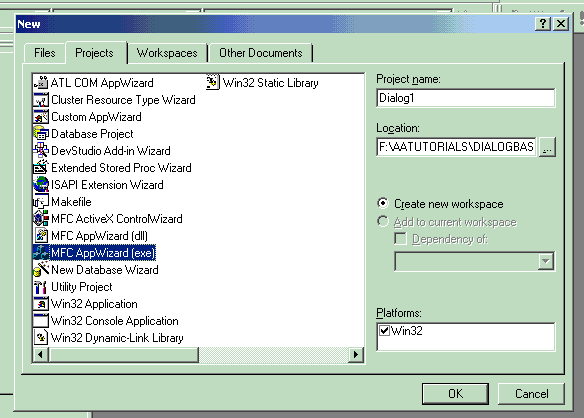
Write Dialog1 in the project name and choose an MFC AppWizard (exe) from the list of the available Wizards, then click OK. The MFC Application Wizard will start.
- In Step 1 of the Wizard, click on Dialog based option, then click Next.
- In Steps 2 and 3, accept the default settings, just click Next to do this.
- In Step 4, click Finish, then you will get the project information. Click OK.
Now the Wizard has created for you a simple empty Dialog Based application. You will need to make few changes and write the necessary code for your application to customise it to your specifications.
Designing the Dialog
Click on the text TODO : Place dialog controls here, then press Delete. This will remove the unwanted text label.

Click on bottom right edge of the dialog box. A rectangle appears around the edges of the box itself. Resize the dialog box using the sizing points as shown below.
Click on the sizing point on the bottom-right corner, keeping the mouse button pressed. Move the mouse, keeping the button pressed. An outline of the new dialog box size is shown as the mouse is moved. Release the mouse button when the dialog box size is 230 x 126.

Click on the Cancel button and press Delete to remove the Cancel button, then right click the mouse on the OK button and a context menu will appear as shown below.
Select Properties from the context menu.

The Push Button Properties dialog box appears. Select the General tab; then in Caption box, replace the word OK with Close as shown below.

On the controls toolbar, select the static text control. Click the dialog box near the upper left corner. A static text control appears on the dialog box, with the default text Static.
Static text controls can be used to display some information (we are going to use few of them as labels). You need three static text controls. Therefore, you need to repeat the process of dropping static text control on to the dialog box. Make sure to drop each control next to each other.
On the controls toolbar, select the edit control and as you did before with text control. Drop two edit controls. Select Combo Box from the controls toolbar and drop it into the dialog box. Then select List Box control and drop it to the dialog box. Select button control from the controls toolbar and drop it into the dialog box.
Change the Caption of the new button to Add and its ID to IDC_ADD, then arrange the controls on the dialog box as show below. Right click static text control and change its properties change Caption to Title as shown below:

Change the Caption for each static text control as shown below. Change the ID in Edit Properties for first Edit Box control to IDC_FIRSTNAME and the second edit control to IDC_LASTNAME.

Change the ID in List Box Properties to IDC_NAMELIST and the ID Combo Box Properties to IDC_TITLE.
Click on Data in Combo Box Properties and populate the Data as shown below. After each entry is added in the list, remember to press Ctrl + Enter to go to a new line.

On the Styles tab, change the Combo Box's Type to Drop List. Position your mouse over the Combo Box dropdown button, then left-click. Another rectangle will appear (see below) that allows you to specify the size of the open Combo Box's List.

Assigning Member Variables to Controls
Press Ctrl + W to start ClassWizard, or from the View menu select ClassWizard. The MFC ClassWizard
dialog box appears as shown below. Select the Member Variables tab, then select the dialog class in the Class name : combo box; use CDialog1Dlg. Select IDC_FIRSTNAME, click Add Variable button. The dialog box appears (see below). Select Value from Category and select CString
from Member variable type.
Type m_strFirstName
for Member variable name.
Repeat the same process to add CString
member variable m_strLastName for IDC_LASTNAME, and m_strTitle for IDC_TITLE.

For IDC_NAMELIST, add a Member variable of Category Control and Variable Type CListBox as shown below. Select the ClassView tab from the project workspace pane. The class list will appear as shown below. Right click CDialog1Dlg class and select add member variable. The Add Member Variable dialog box will appear. Type CString in the variable and m_strFullName in Variable Name.

Adding Message Handlers for the Controls
Press Ctrl + W to start the ClassWizard, or right click the Add button and select ClassWizard from the context menu. Open ClassWizard, select Message Maps tab. In Class name select CDialog1Dlg
, on Object IDs select IDC_ADD , then choose BN_CLICKED from the Messages list as shown below.
Click on Add Function. Click OK, then click Edit Code. The ClassWizard will write a new empty function called Add()
with the proper prototype in the class header.

Add the code in bold - just below //TODO: Add your control notification handler code here
:
void CDialog1Dlg::OnAdd()
{
CString strTitle ;
int nIndex;
UpdateData();
nIndex = GetDlgItemText(IDC_TITLE, strTitle); m_strFullName = strTitle + " " + m_strFirstName + " " + m_strLastName;
m_NameList.AddString(m_strFullName);
UpdateData(FALSE); }
Building and Running the Program
Click on the Title Combo Box and choose a Title. Write your first name in first name edit box and last name in last name edit box then click Add. If everything goes as planned, then you will get your full name and correct title in the list box as shown below.

This program could be part of a large database project, but for now we going to leave it at that. I hope you enjoyed the tutorial. In my next tutorial, I am going to use some of these controls to develop a simple, but very useful project: a multi-internet search engine and much more.
License
This article has no explicit license attached to it, but may contain usage terms in the article text or the download files themselves. If in doubt, please contact the author via the discussion board below. A list of licenses authors might use can be found here.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.