Introduction
This tutorial will explain how to create a simple Windows Store app that provides functionality similar to the one found in Google Calendar or Outlook Calendar. We will use an open source JavaScript component dhtmlxScheduler (Windows 8 Edition) that allows us to build such an app in seven simple steps. The final code of the tutorial can be used as a start point for building Windows store apps for event management or appointment booking.
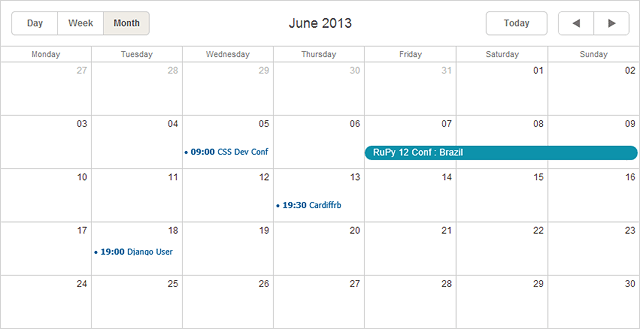
The final demo includes a basic scheduler that displays events in Day, Week, and Month view. You can use this demo project as a basis of your future app.
If you are already acquainted with the creation of JavaScript based Windows 8 apps, you can download the demo app with the source code to see how it can be done. Otherwise, just keep reading.
Step 1 - Creating a Project
First, we need to create a new project in Microsoft Visual Studio 2012. So, launch the Visual Studio. In the left pane, go to Start → New Project and select Templates → JavaScript → Windows Store → Blank App. Name the project, for example, SchedulerApp.
Step 2 - Adding Scripts of dhtmlxScheduler
To display the scheduler on a page, you need to include the necessary files of dhtmlxScheduler (.js, .css, images) in your project.
Download the latest version of dhtmlxScheduler for Windows 8 and unpack it. Then do the following:
- In Visual Studio, right click on the Solution Explorer, select Add → New Folder and name the new folder as 'dhtmlxScheduler'.
- Right click on the 'dhtmlxScheduler' folder, select Add → Add existing files and add the files of the dhtmlxScheduler package you've downloaded to the project.
Finally, your project solution will look like this:

Step 3 - Including Scheduler to the Start Page
One of the files that Visual Studio creates automatically when the project is created is default.html. This is the app's start page. When the app runs, it displays the content of this file. We won't change the start page of the project and will place our calendar on default.html.
Let's start modifying the start page from including the required scheduler code files to it.
Required code files are:
- core files: dhtmlxscheduler.js, dhtmlxscheduler.css
- skin files: dhtmlxscheduler_dhx_terrace.js, dhtmlxscheduler_dhx_terrace.css
This is the minimum set of files needed to run an app with dhtmlxScheduler. If you would like to use the advanced functionality of dhtmlxScheduler, which is stored in scheduler's extension files, you need to include the related extension file(s) additionally (they can be found in the 'ext' folder).
This is the code of the default.html file:
//default.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>SchedulerApp</title>
<!--
<link href="./Microsoft.WinJS.1.0/css/ui-dark.css" rel="stylesheet" />
<script src="./Microsoft.WinJS.1.0/js/base.js"></script>
<script src="./Microsoft.WinJS.1.0/js/ui.js"></script>
<!--
<link href="./css/default.css" rel="stylesheet" />
<script src="./js/default.js"></script>
<!--
<script src="./dhtmlxScheduler/dhtmlxscheduler.js"></script>
<link href="./dhtmlxScheduler/dhtmlxscheduler.css" rel="stylesheet" />
<!--
<script src="./dhtmlxScheduler/ext/dhtmlxscheduler_dhx_terrace.js"></script>
<link href="./dhtmlxScheduler/dhtmlxscheduler_dhx_terrace.css" rel="stylesheet" />
</head>
<body>
<p>Content goes here</p>
</body>
</html>
Step 4 - Scheduler Markup on the Start Page
The next thing we need to do is to prepare a place for the scheduler, i.e., define the related DIV containers for the scheduler elements on the start page.
The standard set of 'divs' (needed for the scheduler) looks like this:
//default.html
<div id="scheduler_here" class="dhx_cal_container" style='width:100%; height:100%;'>
<div class="dhx_cal_navline">
<div class="dhx_cal_prev_button"> </div>
<div class="dhx_cal_next_button"> </div>
<div class="dhx_cal_today_button"></div>
<div class="dhx_cal_date"></div>
<div class="dhx_cal_tab" name="day_tab" style="right:204px;"></div>
<div class="dhx_cal_tab" name="week_tab" style="right:140px;"></div>
<div class="dhx_cal_tab" name="month_tab" style="right:76px;"></div>
</div>
<div class="dhx_cal_header">
</div>
<div class="dhx_cal_data">
</div>
</div>
Step 5 - Initialization
When we have finished these preparations, we can move to the scheduler initialization. Note that the scheduler is a static object and can be instantiated on the page once. To refer to the scheduler, we need to use dhtmlxScheduler
or simply scheduler
. The scheduler is initialized by a simple command:
scheduler.init('scheduler_here', new Date(), "week");
Where the parameters are:
- the id or object of the HTML container that the scheduler will be created inside
- the initial date of the scheduler (by default, the current date)
- the name of the initial view (by default, "week")
Then open the /js/default.js file (automatically created by Visual Studio) and initialize the scheduler in the app.onactivated
event handler code, like shown below:
app.onactivated = function (args) {
if (args.detail.kind === activation.ActivationKind.launch) {
if (args.detail.previousExecutionState !== activation.ApplicationExecutionState.terminated) {
WinJS.Utilities.ready(function(){
scheduler.init('scheduler_here', new Date(), "week");
}, true);
} else { }
args.setPromise(WinJS.UI.processAll());
}
};
The WinJS.Utilities.ready()
function ensures that the specified function is executed only after the page has completely loaded.
If you want to configure the scheduler, you can use the same technique as used for the web version (related documentation). For example, to make weeks in the scheduler start from Monday instead of Sunday, we need to add the following code:
scheduler.config.start_on_monday = true;
...
scheduler.init('scheduler_here');
Step 6 - Loading Data in the Calendar
If you run the app now, you will see an empty calendar on the page. It doesn't contain any data yet. There are various ways to store data for the scheduler but there is the only way to load data into it - the parse
method (related documentation):
scheduler.parse(data, datatype);
Let's populate our calendar with some data. For example, we will display a set of developer conferences that take place around the world in July 2013. The data will be provided in JSON format.
var conferences = [
{ text: "EnyoJS workshop at dotJS",
start_date: "06.01.2013 14:00", end_date: "06.01.2013 18:00" },
{ text: "CSS Dev Conf",
start_date: "06.05.2013 09:00", end_date: "06.05.2013 17:00" },
{ text: "RuPy 12 Conf : Brazil",
start_date: "06.07.2013 08:30", end_date: "06.09.2013 18:00" },
{ text: "Cardiffrb",
start_date: "06.13.2013 19:30", end_date: "06.13.2013 22:30" },
{ text: "Django User Group Berlin #32",
start_date: "06.18.2013 19:00", end_date: "06.18.2013 21:00" }
];
Then, in the final default.js file it will be like this (we've moved the scheduler-related code to a separate function to keep the app initialization code clean and set the initial date of the scheduler to make it display our conferences initially) :
(function () {
"use strict";
WinJS.Binding.optimizeBindingReferences = true;
var app = WinJS.Application;
var activation = Windows.ApplicationModel.Activation;
app.onactivated = function (args) {
if (args.detail.kind === activation.ActivationKind.launch) {
if (args.detail.previousExecutionState !==
activation.ApplicationExecutionState.terminated) {
WinJS.Utilities.ready(initScheduler);
} else {
}
args.setPromise(WinJS.UI.processAll());
}
};
app.start();
})();
function initScheduler(){
scheduler.init('scheduler_here', new Date(2012, 11,1));
var conferences = [
{ text: "EnyoJS workshop at dotJS",
start_date: "12.01.2012 14:00",
end_date: "12.01.2012 18:00" },
{ text: "CSS Dev Conf",
start_date: "12.05.2012 09:00",
end_date: "12.05.2012 17:00" },
{ text: "RuPy 12 Conf : Brazil",
start_date: "12.07.2012 08:30",
end_date: "12.09.2012 18:00" },
{ text: "Cardiffrb",
start_date: "12.13.2012 19:30",
end_date: "12.13.2012 22:30" },
{ text: "Django User Group Berlin #32",
start_date: "12.18.2012 19:00",
end_date: "12.18.2012 21:00" }
];
scheduler.parse(conferences, 'json');
}
Step 7 - Storing the Calendar Data
There are different ways to store data for Windows Store apps, including web resources, Indexed Database, HTML5 localStorage, etc. I won't detail implementing CRUD with all possible data sources but will give you some suggestions on this point.
To track user operations in your app, use the next events of dhtmlxScheduler:
onEventDeleted
- fires after the user has deleted the event from the scheduler onEventChanged
- fires after the event has been modified (moved, resized or edited through the details form) onEventAdded
- fires when the used creates a new event
scheduler.attachEvent("onEventDeleted",
function(event_id,event_object){
});
scheduler.attachEvent("onEventChanged", function(event_id, event_object){
});
scheduler.attachEvent("onEventAdded", function(event_id, event_object){
});
Conclusion
If you followed the steps of this tutorial, you now have built a basic Windows Store app with a nice-looking scheduler interface that allows the end users to manage their events and appointments. You can download the source code of the app and customize it further to meet your needs. Using the features of dhtmlxScheduler, you can build more advanced event calendar apps for Windows Store.
Building web apps since 2001. Rich experience in HTML frontends for different platforms.