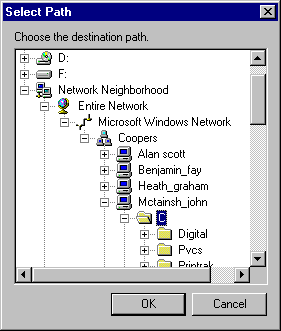
Introduction
This dialog class displays a directory tree similar to the one found in the "Find in Files" Browse button as shown in the image above.
I have noticed several applications requiring a user to select a path that often use CFileDialog
which does not fit the task very well. A better option is to use a Tree control, but I have not found any that allow access to the network other than via mapped drives. Both methods lack the style that "Find in Files" provides. I have also spent some time trying to use SHBrowseForFolder
but to date have had little success.
Why Not Just Use SHBrowseForFolder
Although SHBrowseForFolder
provides all this functionality and more, it does come with the following drawbacks:
- Documentation is difficult to understand and lacks good examples.
- Syntax is very cryptic.
- Many lines of code plus callbacks are necessary to do simple tasks such as set the initial path.
- The
SHBrowseForFolder
dialog cannot be customized to any great extent. For example, changing the dialog's layout or adding a control showing information about the selected directory is not possible.
How to Use It
I have developed this code into a dialog for easy implementation. The source is written such as to put the dialog object into a single class so it can be hacked out and used in any willing developers code. To use:
- Copy the source files DlgGetPath.cpp and DlgGetPath.h to your project directory and add them to your project.
- From within your project, open the TryNetworkTree.rc file and drag the Bitmap (
IDB_FILE_TYPES
) and dialog (IDD_GET_PATH
) into your project. - Add the mpr.lib library in, to be linked into your application. To do this, select 'Project->Settings' to open the project settings dialog. Select the 'Link' tab and from the drop down box, select the 'General' category. In the 'Object/Link modules' edit box, type in mpr.lib.
- Add the following code where you want to activate the picker dialog, for instance within a button handler.
#include "DlgGetPath.h" //Definition to get path
...
void CBlarOnButtonBrowse()
{
CDlgGetPath dlgPath( this );
dlgPath.SetPath( _T("C:\\Temp") );
if( dlgPath.DoModal() == IDOK )
{
}
}
Using Selection Event
When the user selects a branch in the tree, it is possible to catch this event and use the selected path to perform some action. For example, Windows Explorer uses the selection of a branch in the left pane to display the directory in the right pane. To do this, place code at the end of CDlgGetPath::OnSelchangedTree
at around line 391. The path is available and is displayed at the top of the dialog for this sample.
ASSERT( GetDlgItem( IDC_STATIC_NOTE ) );
SetDlgItemText( IDC_STATIC_NOTE, sPath );
Enhancements
- Display the recycle bin appropriately.
- Display the contents of the recycle bin with out S- numbers.
- Sort items when added except for 'Entire Network'.
- Check on a more complex network.
- Determine why icon disappears from the ALT-TAB bar when active?
- Handle
WNetEnumResource
for more than 16384 bytes of data. - It would be nice to read the system icons with
SHGetFileInfo
and use them.
License
This article has no explicit license attached to it, but may contain usage terms in the article text or the download files themselves. If in doubt, please contact the author via the discussion board below.
A list of licenses authors might use can be found here.
I starting writing code when Apple IIe was all the rage (1982?). Graduated Mechanical Engineering in New Zealand, so I could build Robots. Took up Commerical diving in Asia for a few years to make some quick money (Spent it all). I have been writing C/C++/Assembly and building underwater robots since MS QuickC/Quick Assembler in 1989. I currently live in Australia and work mosly with MS VisualC.