What is a Smart Client?
A Smart Client is an application that uses local processing, consumes XML Web Services
and can be deployed and updated from a centralized server. While the .NET Framework
(Windows Forms) and the .NET Compact Framework provide the ability to develop Smart
Clients with ease, other technologies can provide smart client applications by
utilizing the same architecture. Smart Client is the concept of architecting your
application solution into a smart, flexible and convenient platform that utilizes web services for communication.
Why do we need a Smart Client?
To understand the need lets see the pros and cons of both the existing architectures the Thin Client (Web) and the Thick Client (Desktop)
The Thin Client
The Thin clients or the Web application provided features like –
- Easy to Update – Single location update
- Easy to deploy – Single location Update
- Easy to manage.
If it had the above features then it also had the following issues –
- Network dependency – are usually Network based
- Poor user experience – mainly emit HTML
- Complex to develop.
The Thick Client
The Thick clients or the Rich client or Desktop Applications or Client/Server application provided features like –
- Rich User experience – by means of better user interface
- Offline capabilities – Need not be connected on a Network
- High Developer Productivity
- Responsive & Flexible
The Thick did provide the above feature but on the other hand they also had the following issues –
- Tough to Update – Each location needs modifications
- Tough to Deploy – Deployment had to be done at multiple location
- “DLL Hell”
A Smart Client
The above two architectures provide one feature while they lack the other. But a Smart Client combines the best features found in both the architectures.
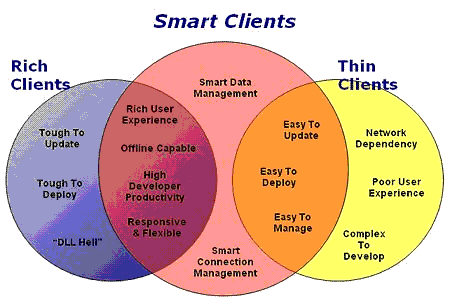
Smart Client Features
A smart client would have the following characteristics:
- Local Resource Utilization
- A smart client application always has code artifacts on the client that enable local resources to be utilized. What do we mean by local resources? We mean everything from hardware to software resources. A smart client may take advantage of the local CPU, local memory or disk, or any local devices connected to the client, such as a telephone, bar-code/RFID reader, and so on. But it may also take advantage of local software, such as Microsoft Office applications, or any installed line-of-business (LOB) applications that interact with it.
- Connected
- Smart client applications are never standalone and always form part of a larger distributed solution. This could mean that the application interacts with a number of Web services that provide access to data or an LOB application. Very often, the application has access to specific services that help maintain the application and provide deployment and update services.
- Offline Capable
- Because they are running on the local machine, one of the key benefits that smart client applications offer is that they can be made to work even when the user is not connected. For applications running in occasional or intermittent connectivity situations, such as those used by traveling workers or even those running on laptops, tablets, PDA's, and so on, where connectivity cannot be guaranteed at all times, being able to work while disconnected is essential. Even when the client is connected, the smart client application can improve performance and usability by caching data and managing the connection in an intelligent way.
- Intelligent Install and Update
- Smart client applications manage their deployment and update in a much more intelligent way than traditional rich client applications. The .NET framework enables application artifacts to be deployed using a variety of techniques, including simple file copy or download over HTTP. Applications can be updated while running and can be deployed on demand by clicking on a URL. The Microsoft(r) .NET Framework provides a powerful security mechanism that guarantees the integrity of the application and its related assemblies. Assemblies can be given limited permissions in order to restrict their functionality in semi-trusted scenarios.
- Client Device Flexibility
- The .NET Framework together with the .NET Compact Framework provides a common platform upon which smart client applications can be built. Often, there will be multiple versions of the smart client application, each targeting a specific device type and taking advantage of the devices unique features and providing functionality appropriate to its usage.
Let us look at one of the very important features of Smart clients – Intelligent Install and Update.
Intelligent Install and Update (Easy Deployment)
Smart client applications manage their deployment and update in a much more intelligent
way than traditional rich client applications.
Components
A Smart client application will have two components
- A very thin client application to be installed locally
- The actual application hosted on a Web Server build using Strong named assemblies
Process involved
When the user opens a Smart client Application –
- The User opens the Application.
- The application references a assembly hosted on a Web Server
- The .NET Framework checks if the previously downloaded assembly is the latest one.
- If not downloads the latest version from the server, loads the assembly locally and launches the application.
Advantages
Any updates to the application, a single change and the changes will be reflected to all the
clients when they are launched the next time. This is called a smart client.
Lets see the Client Application Code –
using System;
using System.Reflection;
using System.Windows.Forms;
namespace MySmartClient
{
class SmartClient
{
[STAThread]
static void Main(string[] args)
{
SmartClient objSmartClient = new SmartClient();
Splash splash = new Splash();
splash.Show();
Application.DoEvents();
string strURL = "http://Server/Smart/Bin/Release/Smart.exe";
string sClassName = "MySmartClient.SmartForm";
Assembly assemblyContent = null;
try
{
assemblyContent = Assembly.LoadFrom(strURL);
}
catch(Exception e)
{
}
splash.Close();
Type typeContent = assemblyContent.GetType(sClassName);
try
{
typeContent.InvokeMember ("Main", BindingFlags.Public |
BindingFlags.InvokeMethod | BindingFlags.Static, null, null, null);
}
catch(Exception e)
{
}
}
}
}
This is all that would be installed on the client. The actual application will be available on the Server.
First time the client application is launched, the .NET Framework will download the required assemblies locally.
Henceforth every time the application is launched, the .NET Framework will check for the version of the assemblies, if the server has a latest version, it will downloaded or else the
locally available assemblies will used to launch the application. This way, any updates to the application can be handled easily at one location, thereby making the deployment of application easy.
Download the client side application and the Server application from the link above.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.